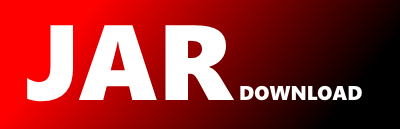
io.serverlessworkflow.api.sleep.Sleep Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessworkflow-api Show documentation
Show all versions of serverlessworkflow-api Show documentation
Java SDK for Serverless Workflow Specification
package io.serverlessworkflow.api.sleep;
import java.io.Serializable;
import javax.validation.constraints.NotNull;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"before",
"after"
})
public class Sleep implements Serializable
{
/**
* Amount of time (ISO 8601 duration format) to sleep before function/subflow invocation. Does not apply if 'eventRef' is defined.
* (Required)
*
*/
@JsonProperty("before")
@JsonPropertyDescription("Amount of time (ISO 8601 duration format) to sleep before function/subflow invocation. Does not apply if 'eventRef' is defined.")
@NotNull
private String before;
/**
* Amount of time (ISO 8601 duration format) to sleep after function/subflow invocation. Does not apply if 'eventRef' is defined.
* (Required)
*
*/
@JsonProperty("after")
@JsonPropertyDescription("Amount of time (ISO 8601 duration format) to sleep after function/subflow invocation. Does not apply if 'eventRef' is defined.")
@NotNull
private String after;
private final static long serialVersionUID = 921205403250153351L;
/**
* No args constructor for use in serialization
*
*/
public Sleep() {
}
/**
*
* @param before
* @param after
*/
public Sleep(String before, String after) {
super();
this.before = before;
this.after = after;
}
/**
* Amount of time (ISO 8601 duration format) to sleep before function/subflow invocation. Does not apply if 'eventRef' is defined.
* (Required)
*
*/
@JsonProperty("before")
public String getBefore() {
return before;
}
/**
* Amount of time (ISO 8601 duration format) to sleep before function/subflow invocation. Does not apply if 'eventRef' is defined.
* (Required)
*
*/
@JsonProperty("before")
public void setBefore(String before) {
this.before = before;
}
public Sleep withBefore(String before) {
this.before = before;
return this;
}
/**
* Amount of time (ISO 8601 duration format) to sleep after function/subflow invocation. Does not apply if 'eventRef' is defined.
* (Required)
*
*/
@JsonProperty("after")
public String getAfter() {
return after;
}
/**
* Amount of time (ISO 8601 duration format) to sleep after function/subflow invocation. Does not apply if 'eventRef' is defined.
* (Required)
*
*/
@JsonProperty("after")
public void setAfter(String after) {
this.after = after;
}
public Sleep withAfter(String after) {
this.after = after;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy