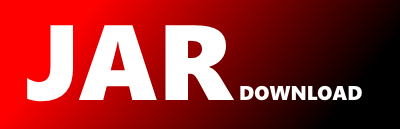
io.serverlessworkflow.api.states.ForEachState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serverlessworkflow-api Show documentation
Show all versions of serverlessworkflow-api Show documentation
Java SDK for Serverless Workflow Specification
package io.serverlessworkflow.api.states;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.validation.Valid;
import javax.validation.constraints.DecimalMin;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyDescription;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import io.serverlessworkflow.api.actions.Action;
import io.serverlessworkflow.api.end.End;
import io.serverlessworkflow.api.error.Error;
import io.serverlessworkflow.api.filters.StateDataFilter;
import io.serverlessworkflow.api.interfaces.State;
import io.serverlessworkflow.api.timeouts.TimeoutsDefinition;
import io.serverlessworkflow.api.transitions.Transition;
/**
* Execute a set of defined actions or workflows for each element of a data array
*
*/
@JsonInclude(JsonInclude.Include.NON_NULL)
@JsonPropertyOrder({
"inputCollection",
"outputCollection",
"iterationParam",
"batchSize",
"actions",
"usedForCompensation",
"mode"
})
public class ForEachState
extends DefaultState
implements Serializable, State
{
/**
* Workflow expression selecting an array element of the states data
*
*/
@JsonProperty("inputCollection")
@JsonPropertyDescription("Workflow expression selecting an array element of the states data")
private java.lang.String inputCollection;
/**
* Workflow expression specifying an array element of the states data to add the results of each iteration
*
*/
@JsonProperty("outputCollection")
@JsonPropertyDescription("Workflow expression specifying an array element of the states data to add the results of each iteration")
private java.lang.String outputCollection;
/**
* Name of the iteration parameter that can be referenced in actions/workflow. For each parallel iteration, this param should contain an unique element of the inputCollection array
*
*/
@JsonProperty("iterationParam")
@JsonPropertyDescription("Name of the iteration parameter that can be referenced in actions/workflow. For each parallel iteration, this param should contain an unique element of the inputCollection array")
private java.lang.String iterationParam;
/**
* Specifies how many iterations may run in parallel at the same time. Used if 'mode' property is set to 'parallel' (default)
*
*/
@JsonProperty("batchSize")
@JsonPropertyDescription("Specifies how many iterations may run in parallel at the same time. Used if 'mode' property is set to 'parallel' (default)")
@DecimalMin("0")
private int batchSize = 0;
/**
* Actions to be executed for each of the elements of inputCollection
*
*/
@JsonProperty("actions")
@JsonPropertyDescription("Actions to be executed for each of the elements of inputCollection")
@Valid
private List actions = new ArrayList();
/**
* If true, this state is used to compensate another state. Default is false
*
*/
@JsonProperty("usedForCompensation")
@JsonPropertyDescription("If true, this state is used to compensate another state. Default is false")
private boolean usedForCompensation = false;
/**
* Specifies how iterations are to be performed (sequentially or in parallel)
*
*/
@JsonProperty("mode")
@JsonPropertyDescription("Specifies how iterations are to be performed (sequentially or in parallel)")
private ForEachState.Mode mode = ForEachState.Mode.fromValue("parallel");
private final static long serialVersionUID = -3623129984146655307L;
/**
* No args constructor for use in serialization
*
*/
public ForEachState() {
}
/**
*
* @param name
* @param type
*/
public ForEachState(java.lang.String name, DefaultState.Type type) {
super(name, type);
}
/**
* Workflow expression selecting an array element of the states data
*
*/
@JsonProperty("inputCollection")
public java.lang.String getInputCollection() {
return inputCollection;
}
/**
* Workflow expression selecting an array element of the states data
*
*/
@JsonProperty("inputCollection")
public void setInputCollection(java.lang.String inputCollection) {
this.inputCollection = inputCollection;
}
public ForEachState withInputCollection(java.lang.String inputCollection) {
this.inputCollection = inputCollection;
return this;
}
/**
* Workflow expression specifying an array element of the states data to add the results of each iteration
*
*/
@JsonProperty("outputCollection")
public java.lang.String getOutputCollection() {
return outputCollection;
}
/**
* Workflow expression specifying an array element of the states data to add the results of each iteration
*
*/
@JsonProperty("outputCollection")
public void setOutputCollection(java.lang.String outputCollection) {
this.outputCollection = outputCollection;
}
public ForEachState withOutputCollection(java.lang.String outputCollection) {
this.outputCollection = outputCollection;
return this;
}
/**
* Name of the iteration parameter that can be referenced in actions/workflow. For each parallel iteration, this param should contain an unique element of the inputCollection array
*
*/
@JsonProperty("iterationParam")
public java.lang.String getIterationParam() {
return iterationParam;
}
/**
* Name of the iteration parameter that can be referenced in actions/workflow. For each parallel iteration, this param should contain an unique element of the inputCollection array
*
*/
@JsonProperty("iterationParam")
public void setIterationParam(java.lang.String iterationParam) {
this.iterationParam = iterationParam;
}
public ForEachState withIterationParam(java.lang.String iterationParam) {
this.iterationParam = iterationParam;
return this;
}
/**
* Specifies how many iterations may run in parallel at the same time. Used if 'mode' property is set to 'parallel' (default)
*
*/
@JsonProperty("batchSize")
public int getBatchSize() {
return batchSize;
}
/**
* Specifies how many iterations may run in parallel at the same time. Used if 'mode' property is set to 'parallel' (default)
*
*/
@JsonProperty("batchSize")
public void setBatchSize(int batchSize) {
this.batchSize = batchSize;
}
public ForEachState withBatchSize(int batchSize) {
this.batchSize = batchSize;
return this;
}
/**
* Actions to be executed for each of the elements of inputCollection
*
*/
@JsonProperty("actions")
public List getActions() {
return actions;
}
/**
* Actions to be executed for each of the elements of inputCollection
*
*/
@JsonProperty("actions")
public void setActions(List actions) {
this.actions = actions;
}
public ForEachState withActions(List actions) {
this.actions = actions;
return this;
}
/**
* If true, this state is used to compensate another state. Default is false
*
*/
@JsonProperty("usedForCompensation")
public boolean isUsedForCompensation() {
return usedForCompensation;
}
/**
* If true, this state is used to compensate another state. Default is false
*
*/
@JsonProperty("usedForCompensation")
public void setUsedForCompensation(boolean usedForCompensation) {
this.usedForCompensation = usedForCompensation;
}
public ForEachState withUsedForCompensation(boolean usedForCompensation) {
this.usedForCompensation = usedForCompensation;
return this;
}
/**
* Specifies how iterations are to be performed (sequentially or in parallel)
*
*/
@JsonProperty("mode")
public ForEachState.Mode getMode() {
return mode;
}
/**
* Specifies how iterations are to be performed (sequentially or in parallel)
*
*/
@JsonProperty("mode")
public void setMode(ForEachState.Mode mode) {
this.mode = mode;
}
public ForEachState withMode(ForEachState.Mode mode) {
this.mode = mode;
return this;
}
@Override
public ForEachState withId(java.lang.String id) {
super.withId(id);
return this;
}
@Override
public ForEachState withName(java.lang.String name) {
super.withName(name);
return this;
}
@Override
public ForEachState withType(DefaultState.Type type) {
super.withType(type);
return this;
}
@Override
public ForEachState withEnd(End end) {
super.withEnd(end);
return this;
}
@Override
public ForEachState withStateDataFilter(StateDataFilter stateDataFilter) {
super.withStateDataFilter(stateDataFilter);
return this;
}
@Override
public ForEachState withMetadata(Map metadata) {
super.withMetadata(metadata);
return this;
}
@Override
public ForEachState withTransition(Transition transition) {
super.withTransition(transition);
return this;
}
@Override
public ForEachState withOnErrors(List onErrors) {
super.withOnErrors(onErrors);
return this;
}
@Override
public ForEachState withCompensatedBy(java.lang.String compensatedBy) {
super.withCompensatedBy(compensatedBy);
return this;
}
@Override
public ForEachState withTimeouts(TimeoutsDefinition timeouts) {
super.withTimeouts(timeouts);
return this;
}
public enum Mode {
SEQUENTIAL("sequential"),
PARALLEL("parallel");
private final java.lang.String value;
private final static Map CONSTANTS = new HashMap();
static {
for (ForEachState.Mode c: values()) {
CONSTANTS.put(c.value, c);
}
}
private Mode(java.lang.String value) {
this.value = value;
}
@Override
public java.lang.String toString() {
return this.value;
}
@JsonValue
public java.lang.String value() {
return this.value;
}
@JsonCreator
public static ForEachState.Mode fromValue(java.lang.String value) {
ForEachState.Mode constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy