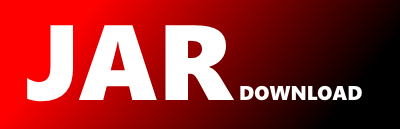
io.servicecomb.core.endpoint.AbstractEndpointsCache Maven / Gradle / Ivy
/*
* Copyright 2017 Huawei Technologies Co., Ltd
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.servicecomb.core.endpoint;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.springframework.util.StringUtils;
import io.servicecomb.core.Transport;
import io.servicecomb.core.transport.TransportManager;
import io.servicecomb.serviceregistry.cache.CacheEndpoint;
import io.servicecomb.serviceregistry.cache.InstanceCache;
import io.servicecomb.serviceregistry.cache.InstanceCacheManager;
/**
* registry模块不理解core中的概念
* 所以要将字符串的各种信息转义一下,方便运行时使用
*/
public abstract class AbstractEndpointsCache {
protected static InstanceCacheManager instanceCacheManager;
protected static TransportManager transportManager;
protected List endpoints = new ArrayList<>();
protected String transportName;
protected InstanceCache instanceCache = null;
public static void init(InstanceCacheManager instanceCacheManager, TransportManager transportManager) {
AbstractEndpointsCache.instanceCacheManager = instanceCacheManager;
AbstractEndpointsCache.transportManager = transportManager;
}
/**
* transportName 可能为"",表示走任意健康的地址即可
*/
public AbstractEndpointsCache(String appId, String microserviceName, String microserviceVersionRule,
String transportName) {
this.transportName = transportName;
this.instanceCache = new InstanceCache(appId, microserviceName, microserviceVersionRule, null);
}
public List getLatestEndpoints() {
InstanceCache newCache = instanceCacheManager.getOrCreate(instanceCache.getAppId(),
instanceCache.getMicroserviceName(),
instanceCache.getMicroserviceVersionRule());
if (!instanceCache.cacheChanged(newCache)) {
return endpoints;
}
// 走到这里,肯定已经是存在"有效"地址了(可能是个空列表,表示没有存活的实例)
// 先创建,成功了,再走下面的更新逻辑
List tmpEndpoints = createEndpints(newCache);
this.instanceCache = newCache;
this.endpoints = tmpEndpoints;
return endpoints;
}
protected List createEndpints(InstanceCache newCache) {
Map> transportMap = getOrCreateTransportMap(newCache);
return createEndpoints(transportMap);
}
protected List createEndpoints(Map> transportMap) {
List tmpEndpoints = new ArrayList<>();
for (Entry> entry : transportMap.entrySet()) {
Transport transport = transportManager.findTransport(entry.getKey());
if (transport == null) {
continue;
}
List endpointList = entry.getValue();
if (endpointList == null) {
continue;
}
for (CacheEndpoint cacheEndpont : endpointList) {
ENDPOINT endpoint = createEndpoint(transport, cacheEndpont);
tmpEndpoints.add(endpoint);
}
}
return tmpEndpoints;
}
private Map> getOrCreateTransportMap(InstanceCache newCache) {
Map> allTransportMap = newCache.getOrCreateTransportMap();
if (StringUtils.isEmpty(transportName)) {
// 未指定tranport,将所有transport全取出来
return allTransportMap;
}
Map> transportMap = new HashMap<>();
transportMap.put(transportName, allTransportMap.get(transportName));
return transportMap;
}
protected abstract ENDPOINT createEndpoint(Transport transport, CacheEndpoint cacheEndpoint);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy