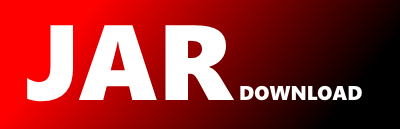
overflowdb.Node Maven / Gradle / Ivy
package overflowdb;
import java.util.Iterator;
import java.util.Map;
import org.apache.tinkerpop.gremlin.structure.Vertex;
public interface Node extends OdbElement, Vertex {
/**
* Add an outgoing edge to the node with provided label and edge properties as key/value pairs.
* These key/values must be provided in an even number where the odd numbered arguments are {@link String}
* property keys and the even numbered arguments are the related property values.
*/
// TODO drop suffix `2` after tinkerpop interface is gone
OdbEdge addEdge2(String label, Node inNode, Object... keyValues);
/**
* Add an outgoing edge to the node with provided label and edge properties as key/value pairs.
*/
// TODO drop suffix `2` after tinkerpop interface is gone
OdbEdge addEdge2(String label, Node inNode, Map keyValues);
/**
* Add an outgoing edge to the node with provided label and edge properties as key/value pairs.
* These key/values must be provided in an even number where the odd numbered arguments are {@link String}
* property keys and the even numbered arguments are the related property values.
* Just like {{{addEdge2}}, but doesn't instantiate and return a dummy edge
*/
void addEdgeSilent(String label, Node inNode, Object... keyValues);
/**
* Add an outgoing edge to the node with provided label and edge properties as key/value pairs.
* Just like {{{addEdge2}}, but doesn't instantiate and return a dummy edge
*/
void addEdgeSilent(String label, Node inNode, Map keyValues);
// TODO drop suffix `2` after tinkerpop interface is gone
long id2();
/* adjacent OUT nodes (all labels) */
Iterator out();
/* adjacent OUT nodes for given labels */
Iterator out(String... edgeLabels);
/* adjacent IN nodes (all labels) */
Iterator in();
/* adjacent IN nodes for given labels */
Iterator in(String... edgeLabels);
/* adjacent OUT/IN nodes (all labels) */
Iterator both();
/* adjacent OUT/IN nodes for given labels */
Iterator both(String... edgeLabels);
/* adjacent OUT edges (all labels) */
Iterator outE();
/* adjacent OUT edges for given labels */
Iterator outE(String... edgeLabels);
/* adjacent IN edges (all labels) */
Iterator inE();
/* adjacent IN edges for given labels */
Iterator inE(String... edgeLabels);
/* adjacent OUT/IN edges (all labels) */
Iterator bothE();
/* adjacent OUT/IN edges for given labels */
Iterator bothE(String... edgeLabels);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy