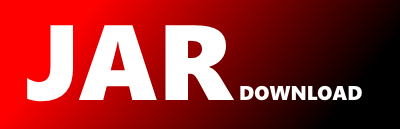
io.sirix.access.trx.node.AbstractDeweyIDManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sirix-core Show documentation
Show all versions of sirix-core Show documentation
SirixDB is a hybrid on-disk and in-memory document oriented, versioned database system. It has a lightweight buffer manager, stores everything in a huge persistent and durable tree and allows efficient reconstruction of every revision. Furthermore, SirixDB implements change tracking, diffing and supports time travel queries.
package io.sirix.access.trx.node;
import io.sirix.api.NodeCursor;
import io.sirix.api.NodeTrx;
import io.sirix.exception.SirixException;
import io.sirix.node.SirixDeweyID;
public abstract class AbstractDeweyIDManager {
/** The node transaction. */
private final W nodeTrx;
/**
* Constructor.
* @param nodeTrx the node transaction
*/
protected AbstractDeweyIDManager(W nodeTrx) {
this.nodeTrx = nodeTrx;
}
/**
* Get an optional first child {@link SirixDeweyID} reference.
*
* @return optional first child {@link SirixDeweyID} reference
* @throws SirixException if generating an ID fails
*/
public SirixDeweyID newFirstChildID() {
SirixDeweyID id = null;
if (nodeTrx.storeDeweyIDs()) {
if (nodeTrx.hasFirstChild()) {
nodeTrx.moveToFirstChild();
id = SirixDeweyID.newBetween(null, nodeTrx.getDeweyID());
} else {
id = nodeTrx.getDeweyID().getNewChildID();
}
}
return id;
}
/**
* Get an optional last child {@link SirixDeweyID} reference.
*
* @return optional last child {@link SirixDeweyID} reference
* @throws SirixException if generating an ID fails
*/
public SirixDeweyID newLastChildID() {
SirixDeweyID id = null;
if (nodeTrx.storeDeweyIDs()) {
if (nodeTrx.hasLastChild()) {
nodeTrx.moveToLastChild();
id = SirixDeweyID.newBetween(nodeTrx.getDeweyID(), null);
} else {
id = nodeTrx.getDeweyID().getNewChildID();
}
}
return id;
}
/**
* Get an optional left sibling {@link SirixDeweyID} reference.
*
* @return optional left sibling {@link SirixDeweyID} reference
* @throws SirixException if generating an ID fails
*/
public SirixDeweyID newLeftSiblingID() {
SirixDeweyID id = null;
if (nodeTrx.storeDeweyIDs()) {
final SirixDeweyID currID = nodeTrx.getDeweyID();
if (nodeTrx.hasLeftSibling()) {
nodeTrx.moveToLeftSibling();
id = SirixDeweyID.newBetween(nodeTrx.getDeweyID(), currID);
nodeTrx.moveToRightSibling();
} else {
id = SirixDeweyID.newBetween(null, currID);
}
}
return id;
}
/**
* Get an optional right sibling {@link SirixDeweyID} reference.
*
* @return optional right sibling {@link SirixDeweyID} reference
* @throws SirixException if generating an ID fails
*/
public SirixDeweyID newRightSiblingID() {
SirixDeweyID id = null;
if (nodeTrx.storeDeweyIDs()) {
final SirixDeweyID currID = nodeTrx.getDeweyID();
if (nodeTrx.hasRightSibling()) {
nodeTrx.moveToRightSibling();
id = SirixDeweyID.newBetween(currID, nodeTrx.getDeweyID());
nodeTrx.moveToLeftSibling();
} else {
id = SirixDeweyID.newBetween(currID, null);
}
}
return id;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy