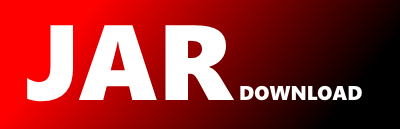
io.sirix.index.AtomicUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sirix-core Show documentation
Show all versions of sirix-core Show documentation
SirixDB is a hybrid on-disk and in-memory document oriented, versioned database system. It has a lightweight buffer manager, stores everything in a huge persistent and durable tree and allows efficient reconstruction of every revision. Furthermore, SirixDB implements change tracking, diffing and supports time travel queries.
package io.sirix.index;
import io.brackit.query.QueryException;
import io.brackit.query.atomic.*;
import io.brackit.query.expr.Cast;
import io.brackit.query.jdm.Type;
import io.sirix.exception.SirixException;
import io.sirix.exception.SirixRuntimeException;
import io.sirix.utils.Calc;
/**
*
* @author Sebastian Baechle
*
*/
public final class AtomicUtil {
// public static Field map(Type type) throws DocumentException {
// if (!type.isBuiltin()) {
// throw new DocumentException("%s is not a built-in type", type);
// }
// if (type.instanceOf(Type.STR)) {
// return Field.STRING;
// }
// if (type.isNumeric()) {
// if (type.instanceOf(Type.DBL)) {
// return Field.DOUBLE;
// }
// if (type.instanceOf(Type.FLO)) {
// return Field.FLOAT;
// }
// if (type.instanceOf(Type.INT)) {
// return Field.INTEGER;
// }
// if (type.instanceOf(Type.LON)) {
// return Field.LONG;
// }
// if (type.instanceOf(Type.INR)) {
// return Field.BIGDECIMAL;
// }
// if (type.instanceOf(Type.DEC)) {
// return Field.BIGDECIMAL;
// }
// }
// throw new DocumentException("Unsupported type: %s", type);
// }
public static byte[] toBytes(Atomic atomic, Type type) throws SirixException {
if (atomic == null) {
return null;
}
return toBytes(toType(atomic, type));
}
public static byte[] toBytes(Atomic atomic) {
if (atomic == null) {
return null;
}
Type type = atomic.type();
if (!type.isBuiltin()) {
throw new SirixRuntimeException("%s is not a built-in type", type);
}
if (type.instanceOf(Type.STR)) {
return Calc.fromString(atomic.stringValue());
}
if (type.instanceOf(Type.BOOL)) {
return atomic.booleanValue() ? new byte[] { (byte) 1 } : new byte[] { (byte) 0 };
}
if (type.isNumeric()) {
if (type.instanceOf(Type.DBL)) {
return Calc.fromDouble(((Numeric) atomic).doubleValue());
}
if (type.instanceOf(Type.FLO)) {
return Calc.fromFloat(((Numeric) atomic).floatValue());
}
if (type.instanceOf(Type.INT)) {
return Calc.fromInt(((Numeric) atomic).intValue());
}
if (type.instanceOf(Type.LON)) {
return Calc.fromLong(((Numeric) atomic).longValue());
}
if (type.instanceOf(Type.INR)) {
return Calc.fromBigDecimal(((Numeric) atomic).decimalValue());
}
if (type.instanceOf(Type.DEC)) {
return Calc.fromBigDecimal(((Numeric) atomic).decimalValue());
}
}
throw new SirixRuntimeException("Unsupported type: %s", type);
}
public static Atomic fromBytes(byte[] b, Type type) {
if (!type.isBuiltin()) {
throw new SirixRuntimeException("%s is not a built-in type", type);
}
if (type.instanceOf(Type.STR)) {
return new Str(Calc.toString(b));
}
if (type.instanceOf(Type.BOOL)) {
return new Bool(b[0] == 1);
}
if (type.isNumeric()) {
if (type.instanceOf(Type.DBL)) {
return new Dbl(Calc.toDouble(b));
}
if (type.instanceOf(Type.FLO)) {
return new Flt(Calc.toFloat(b));
}
if (type.instanceOf(Type.INT)) {
return new Int32(Calc.toInt(b));
}
if (type.instanceOf(Type.LON)) {
return new Int64(Calc.toLong(b));
}
if (type.instanceOf(Type.INR)) {
return new Int(Calc.toBigDecimal(b));
}
if (type.instanceOf(Type.DEC)) {
return new Dec(Calc.toBigDecimal(b));
}
}
throw new SirixRuntimeException("Unsupported type: %s", type);
}
public static Atomic toType(Atomic atomic, Type type) {
try {
return Cast.cast(null, atomic, type);
} catch (final QueryException e) {
throw new SirixRuntimeException(e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy