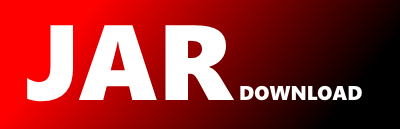
org.sirix.access.ResourceStoreImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sirix-core Show documentation
Show all versions of sirix-core Show documentation
SirixDB is a hybrid on-disk and in-memory document oriented, versioned database system. It has a lightweight buffer manager, stores everything in a huge persistent and durable tree and allows efficient reconstruction of every revision. Furthermore, SirixDB implements change tracking, diffing and supports time travel queries.
package org.sirix.access;
import org.sirix.api.NodeReadOnlyTrx;
import org.sirix.api.NodeTrx;
import org.sirix.api.ResourceManager;
import org.sirix.cache.BufferManager;
import org.checkerframework.checker.nullness.qual.NonNull;
import java.nio.file.Path;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import static com.google.common.base.Preconditions.checkNotNull;
public class ResourceStoreImpl>
implements ResourceStore {
/**
* Central repository of all open resource managers.
*/
private final Map resourceManagers;
private final PathBasedPool> allResourceManagers;
private final ResourceManagerFactory resourceManagerFactory;
public ResourceStoreImpl(final PathBasedPool> allResourceManagers,
final ResourceManagerFactory resourceManagerFactory) {
this.resourceManagers = new ConcurrentHashMap<>();
this.allResourceManagers = allResourceManagers;
this.resourceManagerFactory = resourceManagerFactory;
}
@Override
public R openResource(final @NonNull ResourceConfiguration resourceConfig,
final @NonNull BufferManager bufferManager,
final @NonNull Path resourceFile) {
return this.resourceManagers.computeIfAbsent(resourceFile, k -> {
final var resourceManager = this.resourceManagerFactory.create(resourceConfig, bufferManager, resourceFile);
this.allResourceManagers.putObject(resourceFile, resourceManager);
return resourceManager;
});
}
@Override
public boolean hasOpenResourceManager(final Path resourceFile) {
checkNotNull(resourceFile);
return resourceManagers.containsKey(resourceFile);
}
@Override
public R getOpenResourceManager(final Path resourceFile) {
checkNotNull(resourceFile);
return resourceManagers.get(resourceFile);
}
@Override
public void close() {
resourceManagers.forEach((resourceName, resourceMgr) -> resourceMgr.close());
resourceManagers.clear();
}
@Override
public boolean closeResourceManager(final Path resourceFile) {
final R manager = resourceManagers.remove(resourceFile);
this.allResourceManagers.removeObject(resourceFile, manager);
return manager != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy