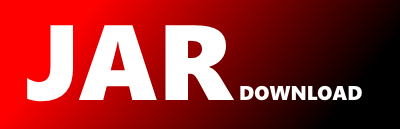
io.sitoolkit.wt.gui.pres.TestCaseDialogController Maven / Gradle / Ivy
The newest version!
package io.sitoolkit.wt.gui.pres;
import java.io.File;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import java.util.ResourceBundle;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.Node;
import javafx.scene.control.ButtonType;
import javafx.scene.control.CheckBox;
import javafx.scene.control.Dialog;
import javafx.scene.control.Label;
import javafx.scene.layout.FlowPane;
import javafx.util.Callback;
public class TestCaseDialogController implements Initializable {
@FXML
private Label testScriptLabel;
@FXML
private FlowPane caseNoFlowPane;
@FXML
private Node content;
TestRunnable testRunnable;
public TestCaseDialogController() {}
@Override
public void initialize(URL location, ResourceBundle resources) {
}
public void showSelectDialog(File currentFile, List caseIdList) {
showDialog(currentFile, caseIdList, false);
}
public void showSelectDebugDialog(File currentFile, List caseIdList) {
showDialog(currentFile, caseIdList, true);
}
public void setTestRunnable(TestRunnable testRunnable) {
this.testRunnable = testRunnable;
}
class CheckBoxResultConverter implements Callback> {
List checkBoxes = new ArrayList<>();
void add(CheckBox checkbox) {
checkBoxes.add(checkbox);
}
@Override
public List call(ButtonType buttonType) {
List selected = new ArrayList<>();
if (buttonType == ButtonType.OK) {
for (CheckBox checkBox : checkBoxes) {
if (checkBox.isSelected()) {
selected.add(checkBox.getText());
}
}
}
return selected;
}
}
private void showDialog(File currentFile, List caseIdList, boolean isDebug) {
caseNoFlowPane.getChildren().clear();
Dialog> dialog = new Dialog<>();
dialog.setTitle("ケースを指定して実行");
dialog.setHeaderText("実行するテストケースを選択してください。");
dialog.getDialogPane().getButtonTypes().addAll(ButtonType.OK, ButtonType.CANCEL);
testScriptLabel.setText(currentFile.getName());
CheckBoxResultConverter resultConverter = new CheckBoxResultConverter();
dialog.setResultConverter(resultConverter);
for (String caseId : caseIdList) {
CheckBox checkBox = new CheckBox(caseId);
resultConverter.add(checkBox);
caseNoFlowPane.getChildren().add(checkBox);
}
dialog.getDialogPane().setContent(content);
Optional> result = dialog.showAndWait();
result.ifPresent(selectedCaseNos -> {
if (!selectedCaseNos.isEmpty()) {
testRunnable.runTest(isDebug, false, currentFile, selectedCaseNos);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy