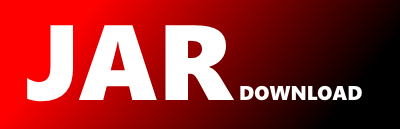
kikaha.caffeine.CacheProducer Maven / Gradle / Ivy
package kikaha.caffeine;
import com.github.benmanes.caffeine.cache.*;
import kikaha.config.Config;
import kikaha.core.cdi.CDI;
import kikaha.core.cdi.ProviderContext;
import lombok.Getter;
import lombok.NonNull;
import lombok.RequiredArgsConstructor;
import lombok.experimental.Delegate;
import lombok.extern.slf4j.Slf4j;
import javax.enterprise.inject.Produces;
import javax.inject.Inject;
import javax.inject.Named;
import javax.inject.Singleton;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import static java.util.concurrent.CompletableFuture.completedFuture;
/**
* A producer of Caffeine caches.
* Created by miere.teixeira on 27/10/2017.
*/
@SuppressWarnings("unchecked")
@Singleton @Slf4j
public class CacheProducer {
static final CacheLoader EMPTY_CACHE_LOADER = s -> null;
static final AsyncCacheLoader EMPTY_ASYNC_CACHE_LOADER = (key, executor) -> completedFuture(null);
private final Map caches = new HashMap<>();
private final Map loadingCaches = new HashMap<>();
private final Map asyncLoadingCaches = new HashMap<>();
@Inject CDI cdi;
@Inject Config config;
@Produces Cache produceCache(ProviderContext context){
final String name = getNameFrom( context );
return new LazyCache( name );
}
@Produces LoadingCache produceLoadingCache(ProviderContext context){
final String name = getNameFrom( context );
return new LazyLoadingCache(
() -> loadingCaches.computeIfAbsent( name,
n -> buildLoadingCache( name, createNewCacheBuilder( name ) ))
);
}
@Produces AsyncLoadingCache produceAsyncLoadingCache(ProviderContext context){
final String name = getNameFrom( context );
return new LazyAsyncLoadingCache( name );
}
private Caffeine
© 2015 - 2025 Weber Informatics LLC | Privacy Policy