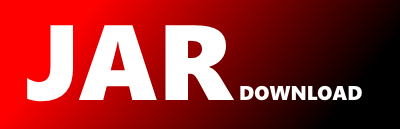
kikaha.uworkers.core.RESTFulExchange Maven / Gradle / Ivy
package kikaha.uworkers.core;
import kikaha.urouting.SimpleExchange;
import kikaha.urouting.api.DefaultResponse;
import kikaha.uworkers.api.*;
import kikaha.uworkers.local.LocalExchange;
import lombok.RequiredArgsConstructor;
import lombok.extern.slf4j.Slf4j;
import java.io.IOException;
import java.util.Map;
import java.util.function.BiConsumer;
/**
* An {@link Exchange} implementation that bridges the communication
* between the REST interface and the uWorkers endpoint.
*/
@RequiredArgsConstructor
public class RESTFulExchange implements Exchange {
final LocalExchange localExchange;
final SimpleExchange exchange;
final byte[] bytes;
@Override
@SuppressWarnings("unchecked")
public REQ request() {
try {
return (REQ)exchange.getRequestBody( Map.class, bytes );
} catch (IOException e) {
throw new IllegalStateException( e );
}
}
@Override
public REQ requestAs(Class targetClass) throws IOException {
try {
return exchange.getRequestBody( targetClass, bytes );
} catch (IOException e) {
throw new IllegalStateException( e );
}
}
@Override
public RESP response() {
return localExchange.response();
}
@Override
public RESP responseAs(Class targetClass) {
return localExchange.responseAs( targetClass );
}
@Override
public Response then(BiConsumer listener) {
return localExchange.then( listener );
}
@Override
public Exchange reply(RESP response) {
return localExchange.reply( response );
}
@Override
public Exchange reply(Throwable error) {
return localExchange.reply( error );
}
}
@Slf4j
@RequiredArgsConstructor( staticName = "to" )
class RESTFulUWorkerResponse implements BiConsumer {
final static kikaha.urouting.api.Response NO_CONTENT = DefaultResponse.noContent();
final SimpleExchange exchange;
@Override
public void accept(Response.UndefinedObject undefinedObject, Throwable throwable) {
try {
sendResponse( undefinedObject, throwable );
} catch ( Throwable cause ) {
log.error( "Could not send response", cause );
} finally {
exchange.endExchange();
}
}
private void sendResponse( Response.UndefinedObject undefinedObject, Throwable throwable ) throws IOException {
if (throwable != null)
exchange.sendResponse(throwable);
else {
final Object object = undefinedObject.get();
if ( object == null || object instanceof TimeStamp )
exchange.sendResponse( NO_CONTENT );
else
exchange.sendResponse( object );
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy