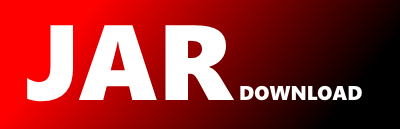
trip.spi.ServiceProvider Maven / Gradle / Ivy
package trip.spi;
import trip.spi.helpers.filter.Condition;
/**
* The main tRip Context. It manages singleton and stateless instances,
* inject data into beans and create new instance of classes that could
* benefits with the tRip's injection mechanism.
*/
public interface ServiceProvider {
/**
* Load a service represented by the argument {@code interfaceClazz}.
* If no service was found, it will try to instantiate the class and
* inject data.
*
* @param interfaceClazz - the service interface(or class) representation
* @return - the loaded or created service.
*/
T load(Class interfaceClazz);
/**
* Load a service represented by the argument {@code interfaceClazz}.
* If no service was found, it will try to instantiate the class and
* inject data.
*
* @param interfaceClazz - the service interface(or class) representation
* @param condition - a filter condition
* @return - the loaded or created service.
*/
T load(Class interfaceClazz, Condition condition);
T load(Class interfaceClazz, ProviderContext context);
T load(Class interfaceClazz, Condition condition, ProviderContext context);
/**
* Load all services represented by the argument {@code interfaceClazz}.
* If no service was found, it will try to instantiate the class,
* inject data and return an {@link Iterable} with this instance.
*
* @param interfaceClazz - the service interface(or class) representation
* @param condition - a filter condition
* @return - all loaded or created services.
*/
Iterable loadAll(Class interfaceClazz, Condition condition);
/**
* Load all services represented by the argument {@code interfaceClazz}.
* If no service was found, it will try to instantiate the class,
* inject data and return an {@link Iterable} with this instance.
*
* @param interfaceClazz - the service interface(or class) representation
* @return - all loaded or created services.
*/
Iterable loadAll(Class interfaceClazz);
/**
* Defines a factory to be invoked every time a service represented with
* {@code interfaceClazz} is requested.
*
* @param interfaceClazz - the service interface(or class) representation
* @param provider - the producer implementation
*/
void providerFor(Class interfaceClazz, ProducerFactory provider);
/**
* Defines a factory to be invoked every time a service represented with
* {@code interfaceClazz} is requested.
*
* @param interfaceClazz - the service interface(or class) representation
* @param object - the service implementation
*/
void providerFor(Class interfaceClazz, T object);
/**
* Inject data into all objects present in {@code iterable}.
*
* @param iterable - the set of objects that will receive "injectable" services.
*/
void provideOn(Iterable iterable);
/**
* Inject data into {@code object}.
*
* @param object - the objects that will receive "injectable" services.
*/
void provideOn(Object object);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy