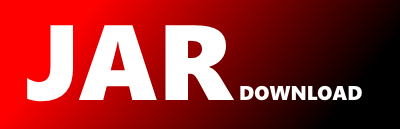
io.smallrye.beanbag.Injector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smallrye-beanbag Show documentation
Show all versions of smallrye-beanbag Show documentation
A trivial programmatic bean container implementation
The newest version!
package io.smallrye.beanbag;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import io.smallrye.common.constraint.Assert;
/**
* An injector step which populates a given instance.
*
* @param the instance type
*/
interface Injector {
void injectInto(Scope scope, C instance);
static Injector forField(Field field, BeanSupplier supplier) {
Assert.checkNotNullParam("field", field);
Assert.checkNotNullParam("supplier", supplier);
final int mods = field.getModifiers();
if (Modifier.isFinal(mods)) {
throw new IllegalArgumentException("Cannot inject into final field " + field.getDeclaringClass().getSimpleName());
}
if (Modifier.isStatic(mods)) {
throw new IllegalArgumentException("Cannot inject into static field " + field.getDeclaringClass().getSimpleName());
}
return new FieldInjector<>(field, supplier);
}
static Injector forSetterMethod(Method method, BeanSupplier supplier) {
Assert.checkNotNullParam("method", method);
Assert.checkNotNullParam("supplier", supplier);
final int mods = method.getModifiers();
if (Modifier.isStatic(mods)) {
throw new IllegalArgumentException(
"Cannot inject into static method " + method.getDeclaringClass().getSimpleName());
}
if (method.getParameterCount() != 1) {
throw new IllegalArgumentException("Cannot inject into method with more than one argument");
}
return new MethodInjector<>(method, supplier);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy