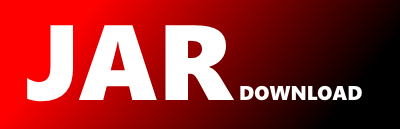
mutiny.zero.operators.Select Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mutiny-zero Show documentation
Show all versions of mutiny-zero Show documentation
Mutiny Zero is a minimal API for creating reactive-streams compliant publishers
package mutiny.zero.operators;
import static java.util.Objects.requireNonNull;
import java.util.concurrent.Flow;
import java.util.function.Predicate;
/**
* A {@link java.util.concurrent.Flow.Publisher} that selects elements matching a {@link Predicate}.
*
* @param the elements type
*/
public class Select implements Flow.Publisher {
private final Flow.Publisher upstream;
private final Predicate predicate;
/**
* Build a new selection publisher.
*
* @param upstream the upstream publisher
* @param predicate the predicate to select the elements forwarded to subscribers, must not throw exceptions
*/
public Select(Flow.Publisher upstream, Predicate predicate) {
this.upstream = requireNonNull(upstream, "The upstream cannot be null");
this.predicate = requireNonNull(predicate, "The predicate cannot be null");
}
@Override
public void subscribe(Flow.Subscriber super T> subscriber) {
requireNonNull(subscriber, "The subscriber cannot be null");
Processor processor = new Processor();
processor.subscribe(subscriber);
upstream.subscribe(processor);
}
private class Processor extends ProcessorBase {
@Override
public void onNext(T item) {
if (!cancelled()) {
try {
if (predicate.test(item)) {
downstream().onNext(item);
}
} catch (Throwable failure) {
cancel();
downstream().onError(failure);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy