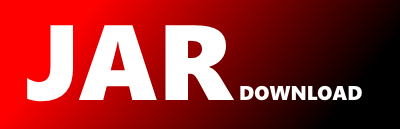
io.smallrye.mutiny.tuples.Tuple2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mutiny Show documentation
Show all versions of mutiny Show documentation
Intuitive Event-Driven Reactive Programming Library for Java
package io.smallrye.mutiny.tuples;
import java.util.Arrays;
import java.util.List;
import java.util.Objects;
import java.util.function.Function;
/**
* A tuple containing two items.
*
* @param The type of the first item
* @param The type of the second item
*/
public class Tuple2 implements Tuple {
final L item1;
final R item2;
protected Tuple2(L left, R right) {
this.item1 = left;
this.item2 = right;
}
public static Tuple2 of(L l, R r) {
return new Tuple2<>(l, r);
}
/**
* Gets the first item.
*
* @return The first item, can be {@code null}
*/
public L getItem1() {
return item1;
}
/**
* Gets the second item.
*
* @return The second item, can be {@code null}
*/
public R getItem2() {
return item2;
}
/**
* Applies a mapper function to the left (item1) part of this {@link Tuple2} to produce a new {@link Tuple2}.
* The right part (item2) is not modified.
*
* @param mapper the mapping {@link Function} for the left item
* @param the new type for the left item
* @return the new {@link Tuple2}
*/
public Tuple2 mapItem1(Function mapper) {
return Tuple2.of(mapper.apply(item1), item2);
}
/**
* Applies a mapper function to the right part (item2) of this {@link Tuple2} to produce a new {@link Tuple2}.
* The left (item1) part is not modified.
*
* @param mapper the mapping {@link Function} for the right item
* @param the new type for the right item
* @return the new {@link Tuple2}
*/
public Tuple2 mapItem2(Function mapper) {
return Tuple2.of(item1, mapper.apply(item2));
}
@Override
public Object nth(int index) {
assertIndexInBounds(index);
if (index == 0) {
return item1;
}
return item2;
}
protected void assertIndexInBounds(int index) {
if (index < 0 || index >= size()) {
throw new IndexOutOfBoundsException(
"Cannot retrieve item at position " + index + ", size is " + size());
}
}
@Override
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy