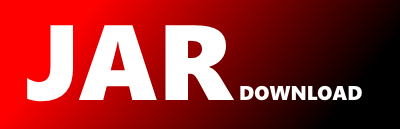
io.vertx.mutiny.ext.auth.oauth2.AccessToken Maven / Gradle / Ivy
package io.vertx.mutiny.ext.auth.oauth2;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import java.util.function.Consumer;
import org.reactivestreams.Subscriber;
import org.reactivestreams.Publisher;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.vertx.core.http.HttpMethod;
import io.vertx.core.json.JsonObject;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
import io.vertx.core.Future;
/**
* AccessToken extension to the User interface
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.auth.oauth2.AccessToken original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.auth.oauth2.AccessToken.class)
public class AccessToken extends io.vertx.mutiny.ext.auth.User {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new AccessToken((io.vertx.ext.auth.oauth2.AccessToken) obj),
AccessToken::getDelegate
);
private final io.vertx.ext.auth.oauth2.AccessToken delegate;
public AccessToken(io.vertx.ext.auth.oauth2.AccessToken delegate) {
super(delegate);
this.delegate = delegate;
}
public AccessToken(Object delegate) {
super((io.vertx.ext.auth.oauth2.AccessToken)delegate);
this.delegate = (io.vertx.ext.auth.oauth2.AccessToken)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
AccessToken() {
super(null);
this.delegate = null;
}
public io.vertx.ext.auth.oauth2.AccessToken getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AccessToken that = (AccessToken) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* @return
*/
public boolean isScopeGranted() {
boolean ret = delegate.isScopeGranted();
return ret;
}
/**
* @return JSON
*/
public JsonObject accessToken() {
if (cached_0 != null) {
return cached_0;
}
JsonObject ret = delegate.accessToken();
cached_0 = ret;
return ret;
}
/**
* @return JSON
*/
public JsonObject idToken() {
if (cached_1 != null) {
return cached_1;
}
JsonObject ret = delegate.idToken();
cached_1 = ret;
return ret;
}
/**
* @return String
*/
public String opaqueAccessToken() {
String ret = delegate.opaqueAccessToken();
return ret;
}
/**
* @return String
*/
public String opaqueRefreshToken() {
String ret = delegate.opaqueRefreshToken();
return ret;
}
/**
* @return String
*/
public String opaqueIdToken() {
String ret = delegate.opaqueIdToken();
return ret;
}
public String tokenType() {
String ret = delegate.tokenType();
return ret;
}
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.AccessToken setTrustJWT(boolean trust) {
delegate.setTrustJWT(trust);
return this;
}
/**
* Refresh the access token
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni refresh() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(callback -> {
delegate.refresh(callback);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#refresh}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Void instance produced by the operation.
*/
public Void refreshAndAwait() {
return (Void) refresh().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#refresh} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#refresh}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#refresh} but you don't need to compose it with other operations.
* @return the instance of AccessToken to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.AccessToken refreshAndForget() {
refresh().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Revoke access or refresh token
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param token_type - A String containing the type of token to revoke. Should be either "access_token" or "refresh_token".
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni revoke(String token_type) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(callback -> {
delegate.revoke(token_type, callback);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#revoke(String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param token_type - A String containing the type of token to revoke. Should be either "access_token" or "refresh_token".
* @return the Void instance produced by the operation.
*/
public Void revokeAndAwait(String token_type) {
return (Void) revoke(token_type).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#revoke(String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#revoke(String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#revoke(String)} but you don't need to compose it with other operations.
* @param token_type - A String containing the type of token to revoke. Should be either "access_token" or "refresh_token".
* @return the instance of AccessToken to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.AccessToken revokeAndForget(String token_type) {
revoke(token_type).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Revoke refresh token and calls the logout endpoint. This is a openid-connect extension and might not be
* available on all providers.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni logout() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(callback -> {
delegate.logout(callback);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#logout}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Void instance produced by the operation.
*/
public Void logoutAndAwait() {
return (Void) logout().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#logout} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#logout}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#logout} but you don't need to compose it with other operations.
* @return the instance of AccessToken to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.AccessToken logoutAndForget() {
logout().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Introspect access token. This is an OAuth2 extension that allow to verify if an access token is still valid.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni introspect() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(callback -> {
delegate.introspect(callback);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#introspect}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Void instance produced by the operation.
*/
public Void introspectAndAwait() {
return (Void) introspect().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#introspect} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#introspect}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#introspect} but you don't need to compose it with other operations.
* @return the instance of AccessToken to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.AccessToken introspectAndForget() {
introspect().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Introspect access token. This is an OAuth2 extension that allow to verify if an access token is still valid.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param tokenType - A String containing the type of token to revoke. Should be either "access_token" or "refresh_token".
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni introspect(String tokenType) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(callback -> {
delegate.introspect(tokenType, callback);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#introspect(String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param tokenType - A String containing the type of token to revoke. Should be either "access_token" or "refresh_token".
* @return the Void instance produced by the operation.
*/
public Void introspectAndAwait(String tokenType) {
return (Void) introspect(tokenType).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#introspect(String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#introspect(String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#introspect(String)} but you don't need to compose it with other operations.
* @param tokenType - A String containing the type of token to revoke. Should be either "access_token" or "refresh_token".
* @return the instance of AccessToken to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.AccessToken introspectAndForget(String tokenType) {
introspect(tokenType).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Load the user info as per OIDC spec.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni userInfo() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(callback -> {
delegate.userInfo(callback);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#userInfo}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the JsonObject instance produced by the operation.
*/
public JsonObject userInfoAndAwait() {
return (JsonObject) userInfo().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#userInfo} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#userInfo}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#userInfo} but you don't need to compose it with other operations.
* @return the instance of AccessToken to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.AccessToken userInfoAndForget() {
userInfo().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Fetches a JSON resource using this Access Token.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param resource - the resource to fetch.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni fetch(String resource) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(callback -> {
delegate.fetch(resource, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
callback.handle(io.vertx.core.Future.succeededFuture(io.vertx.mutiny.ext.auth.oauth2.OAuth2Response.newInstance((io.vertx.ext.auth.oauth2.OAuth2Response)ar.result())));
} else {
callback.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#fetch(String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param resource - the resource to fetch.
* @return the OAuth2Response instance produced by the operation.
*/
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Response fetchAndAwait(String resource) {
return (io.vertx.mutiny.ext.auth.oauth2.OAuth2Response) fetch(resource).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#fetch(String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#fetch(String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#fetch(String)} but you don't need to compose it with other operations.
* @param resource - the resource to fetch.
* @return the instance of AccessToken to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.AccessToken fetchAndForget(String resource) {
fetch(resource).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Fetches a JSON resource using this Access Token.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param method - the HTTP method to user.
* @param resource - the resource to fetch.
* @param headers - extra headers to pass to the request.
* @param payload - payload to send to the server.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni fetch(io.vertx.core.http.HttpMethod method, String resource, JsonObject headers, io.vertx.mutiny.core.buffer.Buffer payload) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(callback -> {
delegate.fetch(method, resource, headers, payload.getDelegate(), new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
callback.handle(io.vertx.core.Future.succeededFuture(io.vertx.mutiny.ext.auth.oauth2.OAuth2Response.newInstance((io.vertx.ext.auth.oauth2.OAuth2Response)ar.result())));
} else {
callback.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#fetch(HttpMethod,String,JsonObject,io.vertx.mutiny.core.buffer.Buffer)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param method - the HTTP method to user.
* @param resource - the resource to fetch.
* @param headers - extra headers to pass to the request.
* @param payload - payload to send to the server.
* @return the OAuth2Response instance produced by the operation.
*/
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Response fetchAndAwait(io.vertx.core.http.HttpMethod method, String resource, JsonObject headers, io.vertx.mutiny.core.buffer.Buffer payload) {
return (io.vertx.mutiny.ext.auth.oauth2.OAuth2Response) fetch(method, resource, headers, payload).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#fetch(HttpMethod,String,JsonObject,io.vertx.mutiny.core.buffer.Buffer)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#fetch(HttpMethod,String,JsonObject,io.vertx.mutiny.core.buffer.Buffer)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken#fetch(HttpMethod,String,JsonObject,io.vertx.mutiny.core.buffer.Buffer)} but you don't need to compose it with other operations.
* @param method - the HTTP method to user.
* @param resource - the resource to fetch.
* @param headers - extra headers to pass to the request.
* @param payload - payload to send to the server.
* @return the instance of AccessToken to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.AccessToken fetchAndForget(io.vertx.core.http.HttpMethod method, String resource, JsonObject headers, io.vertx.mutiny.core.buffer.Buffer payload) {
fetch(method, resource, headers, payload).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
private JsonObject cached_0;
private JsonObject cached_1;
public static AccessToken newInstance(io.vertx.ext.auth.oauth2.AccessToken arg) {
return arg != null ? new AccessToken(arg) : null;
}
}