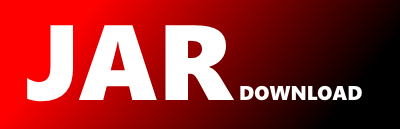
io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth Maven / Gradle / Ivy
package io.vertx.mutiny.ext.auth.oauth2;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import java.util.function.Consumer;
import org.reactivestreams.Subscriber;
import org.reactivestreams.Publisher;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.vertx.ext.auth.oauth2.OAuth2Options;
import io.vertx.ext.auth.oauth2.OAuth2FlowType;
import io.vertx.core.Future;
import io.vertx.core.json.JsonObject;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
/**
* Factory interface for creating OAuth2 based {@link io.vertx.mutiny.ext.auth.authentication.AuthenticationProvider} instances.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.auth.oauth2.OAuth2Auth original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.auth.oauth2.OAuth2Auth.class)
public class OAuth2Auth extends io.vertx.mutiny.ext.auth.authentication.AuthenticationProvider {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new OAuth2Auth((io.vertx.ext.auth.oauth2.OAuth2Auth) obj),
OAuth2Auth::getDelegate
);
private final io.vertx.ext.auth.oauth2.OAuth2Auth delegate;
public OAuth2Auth(io.vertx.ext.auth.oauth2.OAuth2Auth delegate) {
super(delegate);
this.delegate = delegate;
}
public OAuth2Auth(Object delegate) {
super((io.vertx.ext.auth.oauth2.OAuth2Auth)delegate);
this.delegate = (io.vertx.ext.auth.oauth2.OAuth2Auth)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
OAuth2Auth() {
super(null);
this.delegate = null;
}
public io.vertx.ext.auth.oauth2.OAuth2Auth getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
OAuth2Auth that = (OAuth2Auth) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* @param vertx the Vertx instance
* @return the auth provider
*/
public static io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth create(io.vertx.mutiny.core.Vertx vertx) {
io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth ret = io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth.newInstance((io.vertx.ext.auth.oauth2.OAuth2Auth)io.vertx.ext.auth.oauth2.OAuth2Auth.create(vertx.getDelegate()));
return ret;
}
/**
* @param vertx the Vertx instance
* @param config the config
* @return the auth provider
*/
public static io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth create(io.vertx.mutiny.core.Vertx vertx, io.vertx.ext.auth.oauth2.OAuth2Options config) {
io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth ret = io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth.newInstance((io.vertx.ext.auth.oauth2.OAuth2Auth)io.vertx.ext.auth.oauth2.OAuth2Auth.create(vertx.getDelegate(), config));
return ret;
}
/**
* Retrieve the public server JSON Web Key (JWK) required to verify the authenticity
* of issued ID and access tokens. The provider will refresh the keys according to:
* https://openid.net/specs/openid-connect-core-1_0.html#RotateEncKeys
*
* This means that the provider will look at the cache headers and will refresh when
* the max-age is reached. If the server does not return any cache headers it shall
* be up to the end user to call this method to refresh.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni jWKSet() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.jWKSet(handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#jWKSet}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Void instance produced by the operation.
*/
public Void jWKSetAndAwait() {
return (Void) jWKSet().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#jWKSet} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#jWKSet}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#jWKSet} but you don't need to compose it with other operations.
* @return the instance of OAuth2Auth to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth jWKSetAndForget() {
jWKSet().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* @param handler
* @return Future result.
*/
@Fluent
private io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth __missingKeyHandler(Handler handler) {
delegate.missingKeyHandler(handler);
return this;
}
/**
* @param handler
* @return
*/
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth missingKeyHandler(java.util.function.Consumer handler) {
return __missingKeyHandler(handler != null ? handler::accept : null);
}
/**
* @param params extra params to be included in the final URL.
* @return the url to be used to authorize the user.
*/
public String authorizeURL(JsonObject params) {
String ret = delegate.authorizeURL(params);
return ret;
}
/**
* Refresh the current User (access token).
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param user the user (access token) to be refreshed.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni refresh(io.vertx.mutiny.ext.auth.User user) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.refresh(user.getDelegate(), new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.mutiny.ext.auth.User.newInstance((io.vertx.ext.auth.User)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#refresh(io.vertx.mutiny.ext.auth.User)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param user the user (access token) to be refreshed.
* @return the User instance produced by the operation.
*/
public io.vertx.mutiny.ext.auth.User refreshAndAwait(io.vertx.mutiny.ext.auth.User user) {
return (io.vertx.mutiny.ext.auth.User) refresh(user).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#refresh(io.vertx.mutiny.ext.auth.User)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#refresh(io.vertx.mutiny.ext.auth.User)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#refresh(io.vertx.mutiny.ext.auth.User)} but you don't need to compose it with other operations.
* @param user the user (access token) to be refreshed.
* @return the instance of OAuth2Auth to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth refreshAndForget(io.vertx.mutiny.ext.auth.User user) {
refresh(user).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Revoke an obtained access or refresh token. More info https://tools.ietf.org/html/rfc7009.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param user the user (access token) to revoke.
* @param tokenType the token type (either access_token or refresh_token).
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni revoke(io.vertx.mutiny.ext.auth.User user, String tokenType) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.revoke(user.getDelegate(), tokenType, handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#revoke(io.vertx.mutiny.ext.auth.User,String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param user the user (access token) to revoke.
* @param tokenType the token type (either access_token or refresh_token).
* @return the Void instance produced by the operation.
*/
public Void revokeAndAwait(io.vertx.mutiny.ext.auth.User user, String tokenType) {
return (Void) revoke(user, tokenType).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#revoke(io.vertx.mutiny.ext.auth.User,String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#revoke(io.vertx.mutiny.ext.auth.User,String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#revoke(io.vertx.mutiny.ext.auth.User,String)} but you don't need to compose it with other operations.
* @param user the user (access token) to revoke.
* @param tokenType the token type (either access_token or refresh_token).
* @return the instance of OAuth2Auth to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth revokeAndForget(io.vertx.mutiny.ext.auth.User user, String tokenType) {
revoke(user, tokenType).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Revoke an obtained access token. More info https://tools.ietf.org/html/rfc7009.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param user the user (access token) to revoke.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni revoke(io.vertx.mutiny.ext.auth.User user) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.revoke(user.getDelegate(), handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#revoke(io.vertx.mutiny.ext.auth.User)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param user the user (access token) to revoke.
* @return the Void instance produced by the operation.
*/
public Void revokeAndAwait(io.vertx.mutiny.ext.auth.User user) {
return (Void) revoke(user).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#revoke(io.vertx.mutiny.ext.auth.User)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#revoke(io.vertx.mutiny.ext.auth.User)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#revoke(io.vertx.mutiny.ext.auth.User)} but you don't need to compose it with other operations.
* @param user the user (access token) to revoke.
* @return the instance of OAuth2Auth to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth revokeAndForget(io.vertx.mutiny.ext.auth.User user) {
revoke(user).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Retrieve profile information and other attributes for a logged-in end-user. More info https://openid.net/specs/openid-connect-core-1_0.html#UserInfo
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param user the user (access token) to fetch the user info.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public io.smallrye.mutiny.Uni userInfo(io.vertx.mutiny.ext.auth.User user) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.userInfo(user.getDelegate(), handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#userInfo(io.vertx.mutiny.ext.auth.User)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param user the user (access token) to fetch the user info.
* @return the JsonObject instance produced by the operation.
*/
public JsonObject userInfoAndAwait(io.vertx.mutiny.ext.auth.User user) {
return (JsonObject) userInfo(user).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#userInfo(io.vertx.mutiny.ext.auth.User)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#userInfo(io.vertx.mutiny.ext.auth.User)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#userInfo(io.vertx.mutiny.ext.auth.User)} but you don't need to compose it with other operations.
* @param user the user (access token) to fetch the user info.
* @return the instance of OAuth2Auth to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth userInfoAndForget(io.vertx.mutiny.ext.auth.User user) {
userInfo(user).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* @param user the user to generate the url for
* @param params extra parameters to apply to the url
* @return the url to end the session.
*/
public String endSessionURL(io.vertx.mutiny.ext.auth.User user, JsonObject params) {
String ret = delegate.endSessionURL(user.getDelegate(), params);
return ret;
}
/**
* @param user the user to generate the url for
* @return the url to end the session.
*/
public String endSessionURL(io.vertx.mutiny.ext.auth.User user) {
String ret = delegate.endSessionURL(user.getDelegate());
return ret;
}
/**
* Decode a token to a {@link io.vertx.mutiny.ext.auth.oauth2.AccessToken} object. This is useful to handle bearer JWT tokens.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param token the access token (base64 string)
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
* @deprecated */
@Deprecated
public io.smallrye.mutiny.Uni decodeToken(String token) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.decodeToken(token, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.mutiny.ext.auth.oauth2.AccessToken.newInstance((io.vertx.ext.auth.oauth2.AccessToken)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#decodeToken(String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param token the access token (base64 string)
* @return the AccessToken instance produced by the operation.
* @deprecated */
@Deprecated
public io.vertx.mutiny.ext.auth.oauth2.AccessToken decodeTokenAndAwait(String token) {
return (io.vertx.mutiny.ext.auth.oauth2.AccessToken) decodeToken(token).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#decodeToken(String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#decodeToken(String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#decodeToken(String)} but you don't need to compose it with other operations.
* @param token the access token (base64 string)
* @return the instance of OAuth2Auth to chain method calls.
* @deprecated */
@Deprecated
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth decodeTokenAndForget(String token) {
decodeToken(token).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Query an OAuth 2.0 authorization server to determine the active state of an OAuth 2.0 token and to determine
* meta-information about this token.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param token the access token (base64 string)
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
* @deprecated */
@Deprecated
public io.smallrye.mutiny.Uni introspectToken(String token) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.introspectToken(token, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.mutiny.ext.auth.oauth2.AccessToken.newInstance((io.vertx.ext.auth.oauth2.AccessToken)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#introspectToken(String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param token the access token (base64 string)
* @return the AccessToken instance produced by the operation.
* @deprecated */
@Deprecated
public io.vertx.mutiny.ext.auth.oauth2.AccessToken introspectTokenAndAwait(String token) {
return (io.vertx.mutiny.ext.auth.oauth2.AccessToken) introspectToken(token).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#introspectToken(String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#introspectToken(String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#introspectToken(String)} but you don't need to compose it with other operations.
* @param token the access token (base64 string)
* @return the instance of OAuth2Auth to chain method calls.
* @deprecated */
@Deprecated
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth introspectTokenAndForget(String token) {
introspectToken(token).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Query an OAuth 2.0 authorization server to determine the active state of an OAuth 2.0 token and to determine
* meta-information about this token.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param token the access token (base64 string)
* @param tokenType hint to the token type e.g.: `access_token`
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
* @deprecated */
@Deprecated
public io.smallrye.mutiny.Uni introspectToken(String token, String tokenType) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.introspectToken(token, tokenType, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.mutiny.ext.auth.oauth2.AccessToken.newInstance((io.vertx.ext.auth.oauth2.AccessToken)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#introspectToken(String,String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param token the access token (base64 string)
* @param tokenType hint to the token type e.g.: `access_token`
* @return the AccessToken instance produced by the operation.
* @deprecated */
@Deprecated
public io.vertx.mutiny.ext.auth.oauth2.AccessToken introspectTokenAndAwait(String token, String tokenType) {
return (io.vertx.mutiny.ext.auth.oauth2.AccessToken) introspectToken(token, tokenType).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#introspectToken(String,String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#introspectToken(String,String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#introspectToken(String,String)} but you don't need to compose it with other operations.
* @param token the access token (base64 string)
* @param tokenType hint to the token type e.g.: `access_token`
* @return the instance of OAuth2Auth to chain method calls.
* @deprecated */
@Deprecated
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth introspectTokenAndForget(String token, String tokenType) {
introspectToken(token, tokenType).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* @return the flow type.
* @deprecated */
@Deprecated
public io.vertx.ext.auth.oauth2.OAuth2FlowType getFlowType() {
io.vertx.ext.auth.oauth2.OAuth2FlowType ret = delegate.getFlowType();
return ret;
}
/**
* Loads a JWK Set from the remote provider.
*
* When calling this method several times, the loaded JWKs are updated in the underlying JWT object.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
* @deprecated Use {@link #jWKSet(Handler)} */
@Deprecated
public io.smallrye.mutiny.Uni loadJWK() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.loadJWK(handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#loadJWK}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Void instance produced by the operation.
* @deprecated Use {@link #jWKSet(Handler)} */
@Deprecated
public Void loadJWKAndAwait() {
return (Void) loadJWK().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#loadJWK} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#loadJWK}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth#loadJWK} but you don't need to compose it with other operations.
* @return the instance of OAuth2Auth to chain method calls.
* @deprecated Use {@link #jWKSet(Handler)} */
@Deprecated
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth loadJWKAndForget() {
loadJWK().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
@Deprecated
@Fluent
public io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth rbacHandler(io.vertx.mutiny.ext.auth.oauth2.OAuth2RBAC rbac) {
delegate.rbacHandler(rbac.getDelegate());
return this;
}
public static OAuth2Auth newInstance(io.vertx.ext.auth.oauth2.OAuth2Auth arg) {
return arg != null ? new OAuth2Auth(arg) : null;
}
}