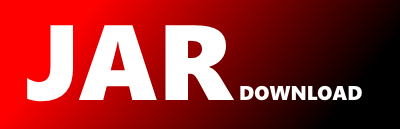
io.vertx.mutiny.ext.auth.oauth2.providers.AzureADAuth Maven / Gradle / Ivy
package io.vertx.mutiny.ext.auth.oauth2.providers;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import java.util.function.Consumer;
import org.reactivestreams.Subscriber;
import org.reactivestreams.Publisher;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.vertx.ext.auth.oauth2.OAuth2Options;
import io.vertx.core.http.HttpClientOptions;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
import io.vertx.core.Future;
/**
* Simplified factory to create an for Azure AD.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.auth.oauth2.providers.AzureADAuth original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.auth.oauth2.providers.AzureADAuth.class)
public class AzureADAuth extends io.vertx.mutiny.ext.auth.oauth2.providers.OpenIDConnectAuth {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new AzureADAuth((io.vertx.ext.auth.oauth2.providers.AzureADAuth) obj),
AzureADAuth::getDelegate
);
private final io.vertx.ext.auth.oauth2.providers.AzureADAuth delegate;
public AzureADAuth(io.vertx.ext.auth.oauth2.providers.AzureADAuth delegate) {
super(delegate);
this.delegate = delegate;
}
public AzureADAuth(Object delegate) {
super((io.vertx.ext.auth.oauth2.providers.AzureADAuth)delegate);
this.delegate = (io.vertx.ext.auth.oauth2.providers.AzureADAuth)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
AzureADAuth() {
super(null);
this.delegate = null;
}
public io.vertx.ext.auth.oauth2.providers.AzureADAuth getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
AzureADAuth that = (AzureADAuth) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* @param vertx
* @param clientId the client id given to you by Azure
* @param clientSecret the client secret given to you by Azure
* @param guid the guid of your application given to you by Azure
* @return
*/
public static io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth create(io.vertx.mutiny.core.Vertx vertx, String clientId, String clientSecret, String guid) {
io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth ret = io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth.newInstance((io.vertx.ext.auth.oauth2.OAuth2Auth)io.vertx.ext.auth.oauth2.providers.AzureADAuth.create(vertx.getDelegate(), clientId, clientSecret, guid));
return ret;
}
/**
* @param vertx
* @param clientId the client id given to you by Azure
* @param clientSecret the client secret given to you by Azure
* @param guid the guid of your application given to you by Azure
* @param httpClientOptions custom http client options
* @return
*/
public static io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth create(io.vertx.mutiny.core.Vertx vertx, String clientId, String clientSecret, String guid, io.vertx.core.http.HttpClientOptions httpClientOptions) {
io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth ret = io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth.newInstance((io.vertx.ext.auth.oauth2.OAuth2Auth)io.vertx.ext.auth.oauth2.providers.AzureADAuth.create(vertx.getDelegate(), clientId, clientSecret, guid, httpClientOptions));
return ret;
}
/**
* Create a OAuth2Auth provider for OpenID Connect Discovery. The discovery will use the default site in the
* configuration options and attempt to load the well known descriptor. If a site is provided (for example when
* running on a custom instance) that site will be used to do the lookup.
*
* If the discovered config includes a json web key url, it will be also fetched and the JWKs will be loaded
* into the OAuth provider so tokens can be decoded.
*
* With this provider, if the given configuration is using the flow type then
* the extra parameters object will include requested_token_use = on_behalf_of
as required by
* https://docs.microsoft.com/en-us/azure/active-directory.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param vertx the vertx instance
* @param config the initial config
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
public static io.smallrye.mutiny.Uni discover(io.vertx.mutiny.core.Vertx vertx, io.vertx.ext.auth.oauth2.OAuth2Options config) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
io.vertx.ext.auth.oauth2.providers.AzureADAuth.discover(vertx.getDelegate(), config, new Handler>() {
public void handle(AsyncResult ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth.newInstance((io.vertx.ext.auth.oauth2.OAuth2Auth)ar.result())));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.oauth2.providers.AzureADAuth#discover(io.vertx.mutiny.core.Vertx,OAuth2Options)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param vertx the vertx instance
* @param config the initial config
* @return the OAuth2Auth instance produced by the operation.
*/
public static io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth discoverAndAwait(io.vertx.mutiny.core.Vertx vertx, io.vertx.ext.auth.oauth2.OAuth2Options config) {
return (io.vertx.mutiny.ext.auth.oauth2.OAuth2Auth) discover(vertx, config).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.oauth2.providers.AzureADAuth#discover(io.vertx.mutiny.core.Vertx,OAuth2Options)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.oauth2.providers.AzureADAuth#discover(io.vertx.mutiny.core.Vertx,OAuth2Options)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.oauth2.providers.AzureADAuth#discover(io.vertx.mutiny.core.Vertx,OAuth2Options)} but you don't need to compose it with other operations.
* @param vertx the vertx instance
* @param config the initial config
*/
public static void discoverAndForget(io.vertx.mutiny.core.Vertx vertx, io.vertx.ext.auth.oauth2.OAuth2Options config) {
discover(vertx, config).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
}
public static AzureADAuth newInstance(io.vertx.ext.auth.oauth2.providers.AzureADAuth arg) {
return arg != null ? new AzureADAuth(arg) : null;
}
}