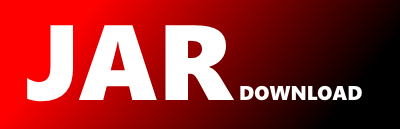
io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil Maven / Gradle / Ivy
package io.vertx.mutiny.ext.auth.sqlclient;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
import io.vertx.core.Future;
/**
* Utility to create users/roles/permissions. This is a helper class and not intended to be a full user
* management utility. While the standard authentication and authorization interfaces will require usually
* read only access to the database, in order to use this API a full read/write access must be granted.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.auth.sqlclient.SqlUserUtil original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.auth.sqlclient.SqlUserUtil.class)
public class SqlUserUtil {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new SqlUserUtil((io.vertx.ext.auth.sqlclient.SqlUserUtil) obj),
SqlUserUtil::getDelegate
);
private final io.vertx.ext.auth.sqlclient.SqlUserUtil delegate;
public SqlUserUtil(io.vertx.ext.auth.sqlclient.SqlUserUtil delegate) {
this.delegate = delegate;
}
public SqlUserUtil(Object delegate) {
this.delegate = (io.vertx.ext.auth.sqlclient.SqlUserUtil)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
SqlUserUtil() {
this.delegate = null;
}
public io.vertx.ext.auth.sqlclient.SqlUserUtil getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SqlUserUtil that = (SqlUserUtil) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* @param client the client with write rights to the database.
* @return the instance
*/
public static io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil create(io.vertx.mutiny.sqlclient.SqlClient client) {
io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil ret = io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil.newInstance((io.vertx.ext.auth.sqlclient.SqlUserUtil)io.vertx.ext.auth.sqlclient.SqlUserUtil.create(client.getDelegate()));
return ret;
}
/**
* @param client the client with write rights to the database.
* @param insertUserSQL
* @param insertUserRoleSQL
* @param insertRolePermissionSQL
* @return the instance
*/
public static io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil create(io.vertx.mutiny.sqlclient.SqlClient client, String insertUserSQL, String insertUserRoleSQL, String insertRolePermissionSQL) {
io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil ret = io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil.newInstance((io.vertx.ext.auth.sqlclient.SqlUserUtil)io.vertx.ext.auth.sqlclient.SqlUserUtil.create(client.getDelegate(), insertUserSQL, insertUserRoleSQL, insertRolePermissionSQL));
return ret;
}
/**
* Insert a user into a database.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param username the username to be set
* @param password the passsword in clear text, will be adapted following the definitions of the defined strategy
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni createUser(String username, String password) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(resultHandler -> {
delegate.createUser(username, password, resultHandler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createUser(String,String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param username the username to be set
* @param password the passsword in clear text, will be adapted following the definitions of the defined strategy
* @return the Void instance produced by the operation.
*/
public Void createUserAndAwait(String username, String password) {
return (Void) createUser(username, password).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createUser(String,String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createUser(String,String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createUser(String,String)} but you don't need to compose it with other operations.
* @param username the username to be set
* @param password the passsword in clear text, will be adapted following the definitions of the defined strategy
* @return the instance of SqlUserUtil to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil createUserAndForget(String username, String password) {
createUser(username, password).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Insert a user into a database.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param username the username to be set
* @param hash the password hash, as result of {@link io.vertx.mutiny.ext.auth.HashingStrategy#hash}
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni createHashedUser(String username, String hash) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(resultHandler -> {
delegate.createHashedUser(username, hash, resultHandler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createHashedUser(String,String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param username the username to be set
* @param hash the password hash, as result of {@link io.vertx.mutiny.ext.auth.HashingStrategy#hash}
* @return the Void instance produced by the operation.
*/
public Void createHashedUserAndAwait(String username, String hash) {
return (Void) createHashedUser(username, hash).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createHashedUser(String,String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createHashedUser(String,String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createHashedUser(String,String)} but you don't need to compose it with other operations.
* @param username the username to be set
* @param hash the password hash, as result of {@link io.vertx.mutiny.ext.auth.HashingStrategy#hash}
* @return the instance of SqlUserUtil to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil createHashedUserAndForget(String username, String hash) {
createHashedUser(username, hash).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Insert a user role into a database.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param username the username to be set
* @param role a to be set
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni createUserRole(String username, String role) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(resultHandler -> {
delegate.createUserRole(username, role, resultHandler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createUserRole(String,String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param username the username to be set
* @param role a to be set
* @return the Void instance produced by the operation.
*/
public Void createUserRoleAndAwait(String username, String role) {
return (Void) createUserRole(username, role).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createUserRole(String,String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createUserRole(String,String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createUserRole(String,String)} but you don't need to compose it with other operations.
* @param username the username to be set
* @param role a to be set
* @return the instance of SqlUserUtil to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil createUserRoleAndForget(String username, String role) {
createUserRole(username, role).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Insert a role permission into a database.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param role a to be set
* @param permission the permission to be set
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni createRolePermission(String role, String permission) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(resultHandler -> {
delegate.createRolePermission(role, permission, resultHandler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createRolePermission(String,String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param role a to be set
* @param permission the permission to be set
* @return the Void instance produced by the operation.
*/
public Void createRolePermissionAndAwait(String role, String permission) {
return (Void) createRolePermission(role, permission).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createRolePermission(String,String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createRolePermission(String,String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil#createRolePermission(String,String)} but you don't need to compose it with other operations.
* @param role a to be set
* @param permission the permission to be set
* @return the instance of SqlUserUtil to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.sqlclient.SqlUserUtil createRolePermissionAndForget(String role, String permission) {
createRolePermission(role, permission).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
public static SqlUserUtil newInstance(io.vertx.ext.auth.sqlclient.SqlUserUtil arg) {
return arg != null ? new SqlUserUtil(arg) : null;
}
}