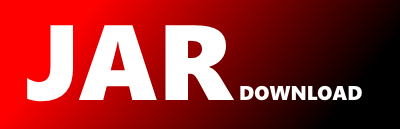
io.vertx.mutiny.ext.auth.webauthn.MetaDataService Maven / Gradle / Ivy
package io.vertx.mutiny.ext.auth.webauthn;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import io.vertx.core.json.JsonObject;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
import io.vertx.ext.auth.webauthn.Authenticator;
import io.vertx.core.Future;
/**
* Factory interface for creating FIDO2 MetaDataService.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.auth.webauthn.MetaDataService original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.auth.webauthn.MetaDataService.class)
public class MetaDataService {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new MetaDataService((io.vertx.ext.auth.webauthn.MetaDataService) obj),
MetaDataService::getDelegate
);
private final io.vertx.ext.auth.webauthn.MetaDataService delegate;
public MetaDataService(io.vertx.ext.auth.webauthn.MetaDataService delegate) {
this.delegate = delegate;
}
public MetaDataService(Object delegate) {
this.delegate = (io.vertx.ext.auth.webauthn.MetaDataService)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
MetaDataService() {
this.delegate = null;
}
public io.vertx.ext.auth.webauthn.MetaDataService getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
MetaDataService that = (MetaDataService) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* Fetches the FIDO2 TOC for the given URL and process the entries to the metadata store.
* Only valid entries will be stored. The operation will return true
only if all
* entries have been added. false
if they have been processed but at least one was
* invalid.
*
* The operation will only fail on network problems.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param url the url to the TOC
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni fetchTOC(String url) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.fetchTOC(url, handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.webauthn.MetaDataService#fetchTOC(String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param url the url to the TOC
* @return the Boolean instance produced by the operation.
*/
public Boolean fetchTOCAndAwait(String url) {
return (Boolean) fetchTOC(url).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.webauthn.MetaDataService#fetchTOC(String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.webauthn.MetaDataService#fetchTOC(String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.webauthn.MetaDataService#fetchTOC(String)} but you don't need to compose it with other operations.
* @param url the url to the TOC
* @return the instance of MetaDataService to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.webauthn.MetaDataService fetchTOCAndForget(String url) {
fetchTOC(url).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Fetches the FIDO2 MDS3 TOC and process the entries to the metadata store.
* Only valid entries will be stored. The operation will return true
only if all
* entries have been added. false
if they have been processed but at least one was
* invalid.
*
* The operation will only fail on network problems.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni fetchTOC() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.fetchTOC(handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.auth.webauthn.MetaDataService#fetchTOC}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Boolean instance produced by the operation.
*/
public Boolean fetchTOCAndAwait() {
return (Boolean) fetchTOC().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.auth.webauthn.MetaDataService#fetchTOC} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.auth.webauthn.MetaDataService#fetchTOC}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.auth.webauthn.MetaDataService#fetchTOC} but you don't need to compose it with other operations.
* @return the instance of MetaDataService to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.auth.webauthn.MetaDataService fetchTOCAndForget() {
fetchTOC().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* @param statement the json statement
* @return fluent self
*/
@Fluent
public io.vertx.mutiny.ext.auth.webauthn.MetaDataService addStatement(JsonObject statement) {
delegate.addStatement(statement);
return this;
}
/**
* @return fluent self
*/
@Fluent
public io.vertx.mutiny.ext.auth.webauthn.MetaDataService flush() {
delegate.flush();
return this;
}
/**
* @param authenticator authenticator to verify
* @return an MDS statement for this authenticator or null
.
*/
public JsonObject verify(io.vertx.ext.auth.webauthn.Authenticator authenticator) {
JsonObject ret = delegate.verify(authenticator);
return ret;
}
public static MetaDataService newInstance(io.vertx.ext.auth.webauthn.MetaDataService arg) {
return arg != null ? new MetaDataService(arg) : null;
}
}