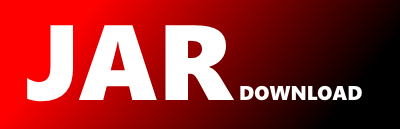
io.vertx.mutiny.db2client.DB2Connection Maven / Gradle / Ivy
package io.vertx.mutiny.db2client;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import io.vertx.db2client.DB2ConnectOptions;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
import io.vertx.core.Future;
/**
* A connection to DB2 server.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.db2client.DB2Connection original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.db2client.DB2Connection.class)
public class DB2Connection extends io.vertx.mutiny.sqlclient.SqlConnection {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new DB2Connection((io.vertx.db2client.DB2Connection) obj),
DB2Connection::getDelegate
);
private final io.vertx.db2client.DB2Connection delegate;
public DB2Connection(io.vertx.db2client.DB2Connection delegate) {
super(delegate);
this.delegate = delegate;
}
public DB2Connection(Object delegate) {
super((io.vertx.db2client.DB2Connection)delegate);
this.delegate = (io.vertx.db2client.DB2Connection)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
DB2Connection() {
super(null);
this.delegate = null;
}
public io.vertx.db2client.DB2Connection getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DB2Connection that = (DB2Connection) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* Create a connection to DB2 server with the given connectOptions
.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param vertx the vertx instance
* @param connectOptions the options for the connection
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public static io.smallrye.mutiny.Uni connect(io.vertx.mutiny.core.Vertx vertx, io.vertx.db2client.DB2ConnectOptions connectOptions) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
io.vertx.db2client.DB2Connection.connect(vertx.getDelegate(), connectOptions, new io.smallrye.mutiny.vertx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.mutiny.db2client.DB2Connection.newInstance((io.vertx.db2client.DB2Connection)event))));
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.db2client.DB2Connection#connect(io.vertx.mutiny.core.Vertx,DB2ConnectOptions)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param vertx the vertx instance
* @param connectOptions the options for the connection
* @return the DB2Connection instance produced by the operation.
*/
public static io.vertx.mutiny.db2client.DB2Connection connectAndAwait(io.vertx.mutiny.core.Vertx vertx, io.vertx.db2client.DB2ConnectOptions connectOptions) {
return (io.vertx.mutiny.db2client.DB2Connection) connect(vertx, connectOptions).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.db2client.DB2Connection#connect(io.vertx.mutiny.core.Vertx,DB2ConnectOptions)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.db2client.DB2Connection#connect(io.vertx.mutiny.core.Vertx,DB2ConnectOptions)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.db2client.DB2Connection#connect(io.vertx.mutiny.core.Vertx,DB2ConnectOptions)} but you don't need to compose it with other operations.
* @param vertx the vertx instance
* @param connectOptions the options for the connection
*/
public static void connectAndForget(io.vertx.mutiny.core.Vertx vertx, io.vertx.db2client.DB2ConnectOptions connectOptions) {
connect(vertx, connectOptions).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
}
/**
* Like {@link io.vertx.mutiny.db2client.DB2Connection#connect} with options build
* from connectionUri
.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param vertx
* @param connectionUri
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public static io.smallrye.mutiny.Uni connect(io.vertx.mutiny.core.Vertx vertx, String connectionUri) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
io.vertx.db2client.DB2Connection.connect(vertx.getDelegate(), connectionUri, new io.smallrye.mutiny.vertx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.mutiny.db2client.DB2Connection.newInstance((io.vertx.db2client.DB2Connection)event))));
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.db2client.DB2Connection#connect(io.vertx.mutiny.core.Vertx,String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param vertx
* @param connectionUri
* @return the DB2Connection instance produced by the operation.
*/
public static io.vertx.mutiny.db2client.DB2Connection connectAndAwait(io.vertx.mutiny.core.Vertx vertx, String connectionUri) {
return (io.vertx.mutiny.db2client.DB2Connection) connect(vertx, connectionUri).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.db2client.DB2Connection#connect(io.vertx.mutiny.core.Vertx,String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.db2client.DB2Connection#connect(io.vertx.mutiny.core.Vertx,String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.db2client.DB2Connection#connect(io.vertx.mutiny.core.Vertx,String)} but you don't need to compose it with other operations.
* @param vertx
* @param connectionUri
*/
public static void connectAndForget(io.vertx.mutiny.core.Vertx vertx, String connectionUri) {
connect(vertx, connectionUri).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
}
@CheckReturnValue
public io.smallrye.mutiny.Uni prepare(String sql) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.prepare(sql, new io.smallrye.mutiny.vertx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.mutiny.sqlclient.PreparedStatement.newInstance((io.vertx.sqlclient.PreparedStatement)event))));
});
}
public io.vertx.mutiny.sqlclient.PreparedStatement prepareAndAwait(String sql) {
return (io.vertx.mutiny.sqlclient.PreparedStatement) prepare(sql).await().indefinitely();
}
@Fluent
public io.vertx.mutiny.db2client.DB2Connection prepareAndForget(String sql) {
prepare(sql).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
@Fluent
private io.vertx.mutiny.db2client.DB2Connection __exceptionHandler(Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
public io.vertx.mutiny.db2client.DB2Connection exceptionHandler(java.util.function.Consumer handler) {
return __exceptionHandler(handler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(handler) : null);
}
@Fluent
private io.vertx.mutiny.db2client.DB2Connection __closeHandler(Handler handler) {
delegate.closeHandler(handler);
return this;
}
public io.vertx.mutiny.db2client.DB2Connection closeHandler(java.lang.Runnable handler) {
return __closeHandler(ignored -> handler.run()
);
}
/**
* Send a PING command to check if the server is alive.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni ping() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.ping(handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.db2client.DB2Connection#ping}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Void instance produced by the operation.
*/
public Void pingAndAwait() {
return (Void) ping().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.db2client.DB2Connection#ping} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.db2client.DB2Connection#ping}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.db2client.DB2Connection#ping} but you don't need to compose it with other operations.
* @return the instance of DB2Connection to chain method calls.
*/
@Fluent
public io.vertx.mutiny.db2client.DB2Connection pingAndForget() {
ping().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Send a DEBUG command to dump debug information to the server's stdout.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni debug() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.debug(handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.db2client.DB2Connection#debug}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Void instance produced by the operation.
*/
public Void debugAndAwait() {
return (Void) debug().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.db2client.DB2Connection#debug} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.db2client.DB2Connection#debug}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.db2client.DB2Connection#debug} but you don't need to compose it with other operations.
* @return the instance of DB2Connection to chain method calls.
*/
@Fluent
public io.vertx.mutiny.db2client.DB2Connection debugAndForget() {
debug().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* @param sqlConnection the connection to cast
* @return a {@link io.vertx.mutiny.db2client.DB2Connection instance}
*/
public static io.vertx.mutiny.db2client.DB2Connection cast(io.vertx.mutiny.sqlclient.SqlConnection sqlConnection) {
io.vertx.mutiny.db2client.DB2Connection ret = io.vertx.mutiny.db2client.DB2Connection.newInstance((io.vertx.db2client.DB2Connection)io.vertx.db2client.DB2Connection.cast(sqlConnection.getDelegate()));
return ret;
}
public static DB2Connection newInstance(io.vertx.db2client.DB2Connection arg) {
return arg != null ? new DB2Connection(arg) : null;
}
}