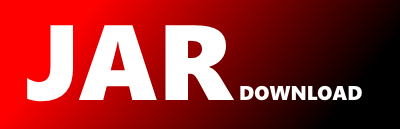
io.smallrye.mutiny.vertx.UniHelper Maven / Gradle / Ivy
package io.smallrye.mutiny.vertx;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.function.Consumer;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.subscription.UniSubscriber;
import io.smallrye.mutiny.subscription.UniSubscription;
import io.vertx.core.AsyncResult;
import io.vertx.core.Future;
import io.vertx.core.Handler;
import io.vertx.core.Promise;
public class UniHelper {
@SuppressWarnings({ "rawtypes", "Convert2Lambda" })
public static Consumer NOOP = new Consumer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy