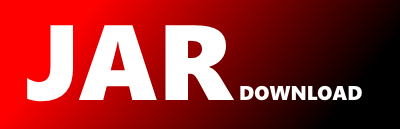
io.smallrye.mutiny.vertx.WriteStreamSubscriber Maven / Gradle / Ivy
package io.smallrye.mutiny.vertx;
import java.util.function.Consumer;
import org.reactivestreams.Subscriber;
/**
* A {@link io.vertx.core.streams.WriteStream} to {@link org.reactivestreams.Subscriber} adapter.
*
* @param the type of item.
*/
@SuppressWarnings("SubscriberImplementation")
public interface WriteStreamSubscriber extends Subscriber {
/**
* Sets the handler to invoke on failure events.
*
* The underlying {@link io.vertx.core.streams.WriteStream#end()} method is not invoked in this case.
*
* @param callback the callback invoked with the failure
* @return a reference to this, so the API can be used fluently
*/
WriteStreamSubscriber onFailure(Consumer super Throwable> callback);
/**
* Sets the handler to invoke on completion events.
*
* The underlying {@link io.vertx.core.streams.WriteStream#end()} method is invoked before the
* given {@code callback}.
*
* @param callback the callback invoked when the completion event is received
* @return a reference to this, so the API can be used fluently
*/
WriteStreamSubscriber onComplete(Runnable callback);
/**
* Sets the handler to invoke if the adapted {@link io.vertx.core.streams.WriteStream} fails.
*
* The underlying {@link io.vertx.core.streams.WriteStream#end()} method is not invoked in this case.
*
* @param callback the callback invoked with the failure
* @return a reference to this, so the API can be used fluently
*/
WriteStreamSubscriber onWriteStreamError(Consumer super Throwable> callback);
}