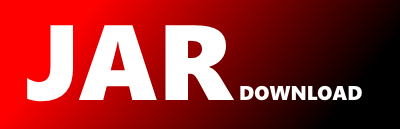
io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler Maven / Gradle / Ivy
package io.vertx.mutiny.ext.web.handler.graphql;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import org.dataloader.DataLoaderRegistry;
import graphql.GraphQL;
import java.util.function.Function;
import io.vertx.ext.web.handler.graphql.ApolloWSOptions;
import graphql.GraphQLContext;
import java.util.Locale;
import io.vertx.core.Handler;
/**
* A handler for GraphQL requests sent over Apollo's subscriptions-transport-ws
transport.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.handler.graphql.ApolloWSHandler original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.handler.graphql.ApolloWSHandler.class)
public class ApolloWSHandler implements io.vertx.mutiny.ext.web.handler.ProtocolUpgradeHandler, io.vertx.core.Handler, java.util.function.Consumer {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new ApolloWSHandler((io.vertx.ext.web.handler.graphql.ApolloWSHandler) obj),
ApolloWSHandler::getDelegate
);
private final io.vertx.ext.web.handler.graphql.ApolloWSHandler delegate;
public ApolloWSHandler(io.vertx.ext.web.handler.graphql.ApolloWSHandler delegate) {
this.delegate = delegate;
}
public ApolloWSHandler(Object delegate) {
this.delegate = (io.vertx.ext.web.handler.graphql.ApolloWSHandler)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
ApolloWSHandler() {
this.delegate = null;
}
public io.vertx.ext.web.handler.graphql.ApolloWSHandler getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ApolloWSHandler that = (ApolloWSHandler) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
@Deprecated
public void handle(io.vertx.mutiny.ext.web.RoutingContext arg0) {
delegate.handle(arg0.getDelegate());
}
/**
* @param connectionHandler
* @return a reference to this, so the API can be used fluently
* @deprecated */
@Deprecated
@Fluent
private io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler __connectionHandler(Handler connectionHandler) {
delegate.connectionHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(connectionHandler, event -> io.vertx.mutiny.core.http.ServerWebSocket.newInstance((io.vertx.core.http.ServerWebSocket)event)));
return this;
}
/**
* @param connectionHandler
* @return
* @deprecated */
@Deprecated
public io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler connectionHandler(java.util.function.Consumer connectionHandler) {
return __connectionHandler(connectionHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(connectionHandler) : null);
}
/**
* @param connectionInitHandler
* @return a reference to this, so the API can be used fluently
* @deprecated */
@Deprecated
@Fluent
private io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler __connectionInitHandler(Handler connectionInitHandler) {
delegate.connectionInitHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(connectionInitHandler, event -> io.vertx.mutiny.ext.web.handler.graphql.ApolloWSConnectionInitEvent.newInstance((io.vertx.ext.web.handler.graphql.ApolloWSConnectionInitEvent)event)));
return this;
}
/**
* @param connectionInitHandler
* @return
* @deprecated */
@Deprecated
public io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler connectionInitHandler(java.util.function.Consumer connectionInitHandler) {
return __connectionInitHandler(connectionInitHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(connectionInitHandler) : null);
}
/**
* @param messageHandler
* @return a reference to this, so the API can be used fluently
* @deprecated */
@Deprecated
@Fluent
private io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler __messageHandler(Handler messageHandler) {
delegate.messageHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(messageHandler, event -> io.vertx.mutiny.ext.web.handler.graphql.ApolloWSMessage.newInstance((io.vertx.ext.web.handler.graphql.ApolloWSMessage)event)));
return this;
}
/**
* @param messageHandler
* @return
* @deprecated */
@Deprecated
public io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler messageHandler(java.util.function.Consumer messageHandler) {
return __messageHandler(messageHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(messageHandler) : null);
}
/**
* @param endHandler
* @return a reference to this, so the API can be used fluently
* @deprecated */
@Deprecated
@Fluent
private io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler __endHandler(Handler endHandler) {
delegate.endHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(endHandler, event -> io.vertx.mutiny.core.http.ServerWebSocket.newInstance((io.vertx.core.http.ServerWebSocket)event)));
return this;
}
/**
* @param endHandler
* @return
* @deprecated */
@Deprecated
public io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler endHandler(java.util.function.Consumer endHandler) {
return __endHandler(endHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(endHandler) : null);
}
/**
* @param factory
* @return a reference to this, so the API can be used fluently
* @deprecated as of 4.2, use {@link #beforeExecute(Handler)} instead */
@Deprecated
@Fluent
public io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler queryContext(Function factory) {
delegate.queryContext(new java.util.function.Function() {
public java.lang.Object apply(io.vertx.ext.web.handler.graphql.ApolloWSMessage arg) {
java.lang.Object ret = factory.apply(io.vertx.mutiny.ext.web.handler.graphql.ApolloWSMessage.newInstance((io.vertx.ext.web.handler.graphql.ApolloWSMessage)arg));
return ret;
}
});
return this;
}
/**
* @param config the callback to invoke
* @return a reference to this, so the API can be used fluently
* @deprecated */
@Deprecated
@Fluent
private io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler __beforeExecute(Handler> config) {
delegate.beforeExecute(new io.smallrye.mutiny.vertx.DelegatingHandler<>(config, event -> io.vertx.mutiny.ext.web.handler.graphql.ExecutionInputBuilderWithContext.newInstance((io.vertx.ext.web.handler.graphql.ExecutionInputBuilderWithContext)event, new TypeArg(o0 -> io.vertx.mutiny.ext.web.handler.graphql.ApolloWSMessage.newInstance((io.vertx.ext.web.handler.graphql.ApolloWSMessage)o0), o0 -> o0.getDelegate()))));
return this;
}
/**
* @param config the callback to invoke
* @return
* @deprecated */
@Deprecated
public io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler beforeExecute(java.util.function.Consumer> config) {
return __beforeExecute(config != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(config) : null);
}
/**
* @param graphQL
* @return
* @deprecated */
@Deprecated
public static io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler create(graphql.GraphQL graphQL) {
io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler ret = io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler.newInstance((io.vertx.ext.web.handler.graphql.ApolloWSHandler)io.vertx.ext.web.handler.graphql.ApolloWSHandler.create(graphQL));
return ret;
}
/**
* @param graphQL
* @param options options for configuring the {@link io.vertx.ext.web.handler.graphql.ApolloWSOptions}
* @return
* @deprecated */
@Deprecated
public static io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler create(graphql.GraphQL graphQL, io.vertx.ext.web.handler.graphql.ApolloWSOptions options) {
io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler ret = io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler.newInstance((io.vertx.ext.web.handler.graphql.ApolloWSHandler)io.vertx.ext.web.handler.graphql.ApolloWSHandler.create(graphQL, options));
return ret;
}
/**
* @param graphQlContext the GraphQL context object
* @return the {@link io.vertx.mutiny.ext.web.handler.graphql.ApolloWSMessage}
* @deprecated */
@Deprecated
public static io.vertx.mutiny.ext.web.handler.graphql.ApolloWSMessage getMessage(graphql.GraphQLContext graphQlContext) {
io.vertx.mutiny.ext.web.handler.graphql.ApolloWSMessage ret = io.vertx.mutiny.ext.web.handler.graphql.ApolloWSMessage.newInstance((io.vertx.ext.web.handler.graphql.ApolloWSMessage)io.vertx.ext.web.handler.graphql.ApolloWSHandler.getMessage(graphQlContext));
return ret;
}
/**
* @param factory
* @return a reference to this, so the API can be used fluently
* @deprecated as of 4.2, use {@link #beforeExecute(Handler)} instead */
@Deprecated
@Fluent
public io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler dataLoaderRegistry(Function factory) {
delegate.dataLoaderRegistry(new java.util.function.Function() {
public org.dataloader.DataLoaderRegistry apply(io.vertx.ext.web.handler.graphql.ApolloWSMessage arg) {
org.dataloader.DataLoaderRegistry ret = factory.apply(io.vertx.mutiny.ext.web.handler.graphql.ApolloWSMessage.newInstance((io.vertx.ext.web.handler.graphql.ApolloWSMessage)arg));
return ret;
}
});
return this;
}
/**
* @param factory
* @return a reference to this, so the API can be used fluently
* @deprecated as of 4.2, use {@link #beforeExecute(Handler)} instead */
@Deprecated
@Fluent
public io.vertx.mutiny.ext.web.handler.graphql.ApolloWSHandler locale(Function factory) {
delegate.locale(new java.util.function.Function() {
public java.util.Locale apply(io.vertx.ext.web.handler.graphql.ApolloWSMessage arg) {
java.util.Locale ret = factory.apply(io.vertx.mutiny.ext.web.handler.graphql.ApolloWSMessage.newInstance((io.vertx.ext.web.handler.graphql.ApolloWSMessage)arg));
return ret;
}
});
return this;
}
public void accept(io.vertx.mutiny.ext.web.RoutingContext item) {
handle(item);
}
public static ApolloWSHandler newInstance(io.vertx.ext.web.handler.graphql.ApolloWSHandler arg) {
return arg != null ? new ApolloWSHandler(arg) : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy