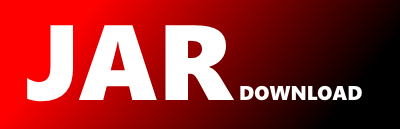
io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler Maven / Gradle / Ivy
package io.vertx.mutiny.ext.web.handler.graphql.ws;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import graphql.GraphQL;
import io.vertx.ext.web.handler.graphql.ws.GraphQLWSOptions;
import io.vertx.core.Handler;
/**
* A handler for the GraphQL over WebSocket Protocol.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler.class)
public class GraphQLWSHandler implements io.vertx.mutiny.ext.web.handler.ProtocolUpgradeHandler, io.vertx.core.Handler, java.util.function.Consumer {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new GraphQLWSHandler((io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler) obj),
GraphQLWSHandler::getDelegate
);
private final io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler delegate;
public GraphQLWSHandler(io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler delegate) {
this.delegate = delegate;
}
public GraphQLWSHandler(Object delegate) {
this.delegate = (io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
GraphQLWSHandler() {
this.delegate = null;
}
public io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
GraphQLWSHandler that = (GraphQLWSHandler) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public void handle(io.vertx.mutiny.ext.web.RoutingContext arg0) {
delegate.handle(arg0.getDelegate());
}
/**
* @param connectionInitHandler
* @return a reference to this, so the API can be used fluently
* @deprecated as of 4.5.1, use {@link #builder(GraphQL)} instead */
@Deprecated
@Fluent
private io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler __connectionInitHandler(Handler connectionInitHandler) {
delegate.connectionInitHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(connectionInitHandler, event -> io.vertx.mutiny.ext.web.handler.graphql.ws.ConnectionInitEvent.newInstance((io.vertx.ext.web.handler.graphql.ws.ConnectionInitEvent)event)));
return this;
}
/**
* @param connectionInitHandler
* @return
* @deprecated as of 4.5.1, use {@link #builder(GraphQL)} instead */
@Deprecated
public io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler connectionInitHandler(java.util.function.Consumer connectionInitHandler) {
return __connectionInitHandler(connectionInitHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(connectionInitHandler) : null);
}
/**
* @param config the callback to invoke
* @return a reference to this, so the API can be used fluently
* @deprecated as of 4.5.1, use {@link #builder(GraphQL)} instead */
@Deprecated
@Fluent
private io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler __beforeExecute(Handler> config) {
delegate.beforeExecute(new io.smallrye.mutiny.vertx.DelegatingHandler<>(config, event -> io.vertx.mutiny.ext.web.handler.graphql.ExecutionInputBuilderWithContext.newInstance((io.vertx.ext.web.handler.graphql.ExecutionInputBuilderWithContext)event, new TypeArg(o0 -> io.vertx.mutiny.ext.web.handler.graphql.ws.Message.newInstance((io.vertx.ext.web.handler.graphql.ws.Message)o0), o0 -> o0.getDelegate()))));
return this;
}
/**
* @param config the callback to invoke
* @return
* @deprecated as of 4.5.1, use {@link #builder(GraphQL)} instead */
@Deprecated
public io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler beforeExecute(java.util.function.Consumer> config) {
return __beforeExecute(config != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(config) : null);
}
/**
* @param messageHandler
* @return a reference to this, so the API can be used fluently
* @deprecated as of 4.5.1, use {@link #builder(GraphQL)} instead */
@Deprecated
@Fluent
private io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler __messageHandler(Handler messageHandler) {
delegate.messageHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(messageHandler, event -> io.vertx.mutiny.ext.web.handler.graphql.ws.Message.newInstance((io.vertx.ext.web.handler.graphql.ws.Message)event)));
return this;
}
/**
* @param messageHandler
* @return
* @deprecated as of 4.5.1, use {@link #builder(GraphQL)} instead */
@Deprecated
public io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler messageHandler(java.util.function.Consumer messageHandler) {
return __messageHandler(messageHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(messageHandler) : null);
}
/**
* @param endHandler
* @return a reference to this, so the API can be used fluently
* @deprecated as of 4.5.1, use {@link #builder(GraphQL)} instead */
@Deprecated
@Fluent
private io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler __endHandler(Handler endHandler) {
delegate.endHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(endHandler, event -> io.vertx.mutiny.core.http.ServerWebSocket.newInstance((io.vertx.core.http.ServerWebSocket)event)));
return this;
}
/**
* @param endHandler
* @return
* @deprecated as of 4.5.1, use {@link #builder(GraphQL)} instead */
@Deprecated
public io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler endHandler(java.util.function.Consumer endHandler) {
return __endHandler(endHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(endHandler) : null);
}
/**
* @param graphQL
* @return
*/
public static io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandlerBuilder builder(graphql.GraphQL graphQL) {
io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandlerBuilder ret = io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandlerBuilder.newInstance((io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandlerBuilder)io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler.builder(graphQL));
return ret;
}
/**
* @param graphQL
* @return
*/
public static io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler create(graphql.GraphQL graphQL) {
io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler ret = io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler.newInstance((io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler)io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler.create(graphQL));
return ret;
}
/**
* @param graphQL
* @param options options for configuring the {@link io.vertx.ext.web.handler.graphql.ws.GraphQLWSOptions}
* @return
*/
public static io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler create(graphql.GraphQL graphQL, io.vertx.ext.web.handler.graphql.ws.GraphQLWSOptions options) {
io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler ret = io.vertx.mutiny.ext.web.handler.graphql.ws.GraphQLWSHandler.newInstance((io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler)io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler.create(graphQL, options));
return ret;
}
public void accept(io.vertx.mutiny.ext.web.RoutingContext item) {
handle(item);
}
public static GraphQLWSHandler newInstance(io.vertx.ext.web.handler.graphql.ws.GraphQLWSHandler arg) {
return arg != null ? new GraphQLWSHandler(arg) : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy