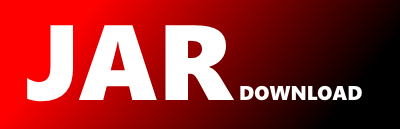
io.vertx.mutiny.ext.web.openapi.RouterBuilder Maven / Gradle / Ivy
package io.vertx.mutiny.ext.web.openapi;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import java.util.function.Function;
import io.vertx.core.Future;
import io.vertx.ext.web.openapi.OpenAPILoaderOptions;
import java.util.List;
import io.vertx.ext.web.openapi.RouterBuilderOptions;
import io.vertx.core.json.JsonObject;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
/**
* Interface to build a Vert.x Web from an OpenAPI 3 contract.
* To add an handler, use {@link io.vertx.mutiny.ext.web.openapi.RouterBuilder#operation} (String, Handler)}
* Usage example:
*
* RouterBuilder.create(vertx, "src/resources/spec.yaml", asyncResult -> {
if (!asyncResult.succeeded()) {
// IO failure or spec invalid
else {
* RouterBuilder routerBuilder = asyncResult.result();
* RouterBuilder.operation("operation_id").handler(routingContext -> {
* // Do something
* }, routingContext -> {
* // Do something with failure handler
* });
* Router router = routerBuilder.createRouter();
* }
* });
* }
*
*
* Handlers are loaded in this order:
*
* - Body handler (Customizable with
* - Custom global handlers configurable with
* - Global security handlers defined in upper spec level
* - Operation specific security handlers
* - Generated validation handler
* - User handlers or "Not implemented" handler
*
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.openapi.RouterBuilder original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.openapi.RouterBuilder.class)
public class RouterBuilder {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new RouterBuilder((io.vertx.ext.web.openapi.RouterBuilder) obj),
RouterBuilder::getDelegate
);
private final io.vertx.ext.web.openapi.RouterBuilder delegate;
public RouterBuilder(io.vertx.ext.web.openapi.RouterBuilder delegate) {
this.delegate = delegate;
}
public RouterBuilder(Object delegate) {
this.delegate = (io.vertx.ext.web.openapi.RouterBuilder)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
RouterBuilder() {
this.delegate = null;
}
public io.vertx.ext.web.openapi.RouterBuilder getDelegate() {
return delegate;
}
static final io.smallrye.mutiny.vertx.TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.mutiny.ext.web.openapi.Operation.newInstance((io.vertx.ext.web.openapi.Operation)o1), o1 -> o1.getDelegate());
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
RouterBuilder that = (RouterBuilder) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* @param operationId the id of the operation
* @return the requested operation
*/
public io.vertx.mutiny.ext.web.openapi.Operation operation(String operationId) {
io.vertx.mutiny.ext.web.openapi.Operation ret = io.vertx.mutiny.ext.web.openapi.Operation.newInstance((io.vertx.ext.web.openapi.Operation)delegate.operation(operationId));
return ret;
}
/**
* @return all operations defined in the contract
*/
public List operations() {
List ret = delegate.operations().stream().map(elt -> io.vertx.mutiny.ext.web.openapi.Operation.newInstance((io.vertx.ext.web.openapi.Operation)elt)).collect(java.util.stream.Collectors.toList());
return ret;
}
/**
* @param bodyHandler
* @return self
* @deprecated Use {@link #rootHandler(Handler)} instead. The order matters, so adding the body handler should happen after any {@code PLATFORM} or {@code SECURITY_POLICY} handler(s). */
@Deprecated
@Fluent
public io.vertx.mutiny.ext.web.openapi.RouterBuilder bodyHandler(io.vertx.mutiny.ext.web.handler.BodyHandler bodyHandler) {
delegate.bodyHandler(bodyHandler.getDelegate());
return this;
}
/**
* @param rootHandler
* @return self
*/
@Fluent
private io.vertx.mutiny.ext.web.openapi.RouterBuilder __rootHandler(Handler rootHandler) {
delegate.rootHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(rootHandler, event -> io.vertx.mutiny.ext.web.RoutingContext.newInstance((io.vertx.ext.web.RoutingContext)event)));
return this;
}
/**
* @param rootHandler
* @return
*/
public io.vertx.mutiny.ext.web.openapi.RouterBuilder rootHandler(java.util.function.Consumer rootHandler) {
return __rootHandler(rootHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(rootHandler) : null);
}
/**
* @return self
*/
@Fluent
public io.vertx.mutiny.ext.web.openapi.RouterBuilder mountServicesFromExtensions() {
delegate.mountServicesFromExtensions();
return this;
}
/**
* @param options
* @return self
*/
@Fluent
public io.vertx.mutiny.ext.web.openapi.RouterBuilder setOptions(io.vertx.ext.web.openapi.RouterBuilderOptions options) {
delegate.setOptions(options);
return this;
}
/**
* @return options of router builder. For more info {@link io.vertx.ext.web.openapi.RouterBuilderOptions}
*/
public io.vertx.ext.web.openapi.RouterBuilderOptions getOptions() {
io.vertx.ext.web.openapi.RouterBuilderOptions ret = delegate.getOptions();
return ret;
}
/**
* @return holder used by self to process the OpenAPI. You can use it to resolve $ref
s
*/
public io.vertx.mutiny.ext.web.openapi.OpenAPIHolder getOpenAPI() {
io.vertx.mutiny.ext.web.openapi.OpenAPIHolder ret = io.vertx.mutiny.ext.web.openapi.OpenAPIHolder.newInstance((io.vertx.ext.web.openapi.OpenAPIHolder)delegate.getOpenAPI());
return ret;
}
/**
* @return schema router used by self to internally manage all {@link io.vertx.mutiny.json.schema.Schema} instances
* @deprecated This method exposes the internal of the OpenAPI handler, it will be removed in the future. Users should configure the json schema module from the options. */
@Deprecated
public io.vertx.mutiny.json.schema.SchemaRouter getSchemaRouter() {
io.vertx.mutiny.json.schema.SchemaRouter ret = io.vertx.mutiny.json.schema.SchemaRouter.newInstance((io.vertx.json.schema.SchemaRouter)delegate.getSchemaRouter());
return ret;
}
/**
* @return schema parser used by self to parse all {@link io.vertx.mutiny.json.schema.Schema}
* @deprecated This method exposes the internal of the OpenAPI handler, it will be removed in the future. Users should configure the json schema module from the options. */
@Deprecated
public io.vertx.mutiny.json.schema.SchemaParser getSchemaParser() {
io.vertx.mutiny.json.schema.SchemaParser ret = io.vertx.mutiny.json.schema.SchemaParser.newInstance((io.vertx.json.schema.SchemaParser)delegate.getSchemaParser());
return ret;
}
/**
* @param serviceExtraPayloadMapper
* @return self
*/
@Fluent
public io.vertx.mutiny.ext.web.openapi.RouterBuilder serviceExtraPayloadMapper(Function serviceExtraPayloadMapper) {
delegate.serviceExtraPayloadMapper(new java.util.function.Function() {
public JsonObject apply(io.vertx.ext.web.RoutingContext arg) {
JsonObject ret = serviceExtraPayloadMapper.apply(io.vertx.mutiny.ext.web.RoutingContext.newInstance((io.vertx.ext.web.RoutingContext)arg));
return ret;
}
});
return this;
}
/**
* @param securitySchemeName
* @return a security scheme.
*/
public io.vertx.mutiny.ext.web.openapi.SecurityScheme securityHandler(String securitySchemeName) {
io.vertx.mutiny.ext.web.openapi.SecurityScheme ret = io.vertx.mutiny.ext.web.openapi.SecurityScheme.newInstance((io.vertx.ext.web.openapi.SecurityScheme)delegate.securityHandler(securitySchemeName));
return ret;
}
/**
* @return
*/
public io.vertx.mutiny.ext.web.Router createRouter() {
io.vertx.mutiny.ext.web.Router ret = io.vertx.mutiny.ext.web.Router.newInstance((io.vertx.ext.web.Router)delegate.createRouter());
return ret;
}
/**
* Like
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param vertx
* @param url
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public static io.smallrye.mutiny.Uni create(io.vertx.mutiny.core.Vertx vertx, String url) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
io.vertx.ext.web.openapi.RouterBuilder.create(vertx.getDelegate(), url, new io.smallrye.mutiny.vertx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.mutiny.ext.web.openapi.RouterBuilder.newInstance((io.vertx.ext.web.openapi.RouterBuilder)event))));
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.openapi.RouterBuilder#create(io.vertx.mutiny.core.Vertx,String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param vertx
* @param url
* @return the RouterBuilder instance produced by the operation.
*/
public static io.vertx.mutiny.ext.web.openapi.RouterBuilder createAndAwait(io.vertx.mutiny.core.Vertx vertx, String url) {
return (io.vertx.mutiny.ext.web.openapi.RouterBuilder) create(vertx, url).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.openapi.RouterBuilder#create(io.vertx.mutiny.core.Vertx,String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.openapi.RouterBuilder#create(io.vertx.mutiny.core.Vertx,String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.openapi.RouterBuilder#create(io.vertx.mutiny.core.Vertx,String)} but you don't need to compose it with other operations.
* @param vertx
* @param url
*/
public static void createAndForget(io.vertx.mutiny.core.Vertx vertx, String url) {
create(vertx, url).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
}
/**
* Like
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param vertx
* @param url
* @param options
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public static io.smallrye.mutiny.Uni create(io.vertx.mutiny.core.Vertx vertx, String url, io.vertx.ext.web.openapi.OpenAPILoaderOptions options) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
io.vertx.ext.web.openapi.RouterBuilder.create(vertx.getDelegate(), url, options, new io.smallrye.mutiny.vertx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.mutiny.ext.web.openapi.RouterBuilder.newInstance((io.vertx.ext.web.openapi.RouterBuilder)event))));
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.openapi.RouterBuilder#create(io.vertx.mutiny.core.Vertx,String,OpenAPILoaderOptions)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param vertx
* @param url
* @param options
* @return the RouterBuilder instance produced by the operation.
*/
public static io.vertx.mutiny.ext.web.openapi.RouterBuilder createAndAwait(io.vertx.mutiny.core.Vertx vertx, String url, io.vertx.ext.web.openapi.OpenAPILoaderOptions options) {
return (io.vertx.mutiny.ext.web.openapi.RouterBuilder) create(vertx, url, options).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.openapi.RouterBuilder#create(io.vertx.mutiny.core.Vertx,String,OpenAPILoaderOptions)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.openapi.RouterBuilder#create(io.vertx.mutiny.core.Vertx,String,OpenAPILoaderOptions)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.openapi.RouterBuilder#create(io.vertx.mutiny.core.Vertx,String,OpenAPILoaderOptions)} but you don't need to compose it with other operations.
* @param vertx
* @param url
* @param options
*/
public static void createAndForget(io.vertx.mutiny.core.Vertx vertx, String url, io.vertx.ext.web.openapi.OpenAPILoaderOptions options) {
create(vertx, url, options).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
}
/**
* @param securitySchemeName the components security scheme id
* @param handler the authentication handler
* @return self
*/
@Fluent
public io.vertx.mutiny.ext.web.openapi.RouterBuilder securityHandler(String securitySchemeName, io.vertx.mutiny.ext.web.handler.AuthenticationHandler handler) {
delegate.securityHandler(securitySchemeName, handler.getDelegate());
return this;
}
public static RouterBuilder newInstance(io.vertx.ext.web.openapi.RouterBuilder arg) {
return arg != null ? new RouterBuilder(arg) : null;
}
}