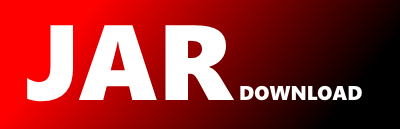
io.vertx.mutiny.ext.web.FileUpload Maven / Gradle / Ivy
package io.vertx.mutiny.ext.web;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import io.vertx.core.Future;
/**
* Represents a file-upload from an HTTP multipart form submission.
*
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.FileUpload original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.FileUpload.class)
public class FileUpload {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new FileUpload((io.vertx.ext.web.FileUpload) obj),
FileUpload::getDelegate
);
private final io.vertx.ext.web.FileUpload delegate;
public FileUpload(io.vertx.ext.web.FileUpload delegate) {
this.delegate = delegate;
}
public FileUpload(Object delegate) {
this.delegate = (io.vertx.ext.web.FileUpload)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
FileUpload() {
this.delegate = null;
}
public io.vertx.ext.web.FileUpload getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
FileUpload that = (FileUpload) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* @return the name of the upload as provided in the form submission
*/
public String name() {
String ret = delegate.name();
return ret;
}
/**
* @return the actual temporary file name on the server where the file was uploaded to.
*/
public String uploadedFileName() {
String ret = delegate.uploadedFileName();
return ret;
}
/**
* @return the file name of the upload as provided in the form submission
*/
public String fileName() {
String ret = delegate.fileName();
return ret;
}
/**
* @return the size of the upload, in bytes
*/
public long size() {
long ret = delegate.size();
return ret;
}
/**
* @return the content type (MIME type) of the upload
*/
public String contentType() {
String ret = delegate.contentType();
return ret;
}
/**
* @return the content transfer encoding of the upload - this describes how the upload was encoded in the form submission.
*/
public String contentTransferEncoding() {
String ret = delegate.contentTransferEncoding();
return ret;
}
/**
* @return the charset of the upload
*/
public String charSet() {
String ret = delegate.charSet();
return ret;
}
/**
* @return true
when the upload was cancelled, false
when the upload is finished and the file is available
*/
public boolean cancel() {
boolean ret = delegate.cancel();
return ret;
}
/**
* Delete the uploaded file on the disk.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni delete() {
return io.smallrye.mutiny.vertx.UniHelper.toUni(delegate.delete());}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.FileUpload#delete}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Void instance produced by the operation.
*/
public Void deleteAndAwait() {
return delete().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.FileUpload#delete} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.FileUpload#delete}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.FileUpload#delete} but you don't need to compose it with other operations.
*/
public void deleteAndForget() {
delete().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
}
public static FileUpload newInstance(io.vertx.ext.web.FileUpload arg) {
return arg != null ? new FileUpload(arg) : null;
}
}