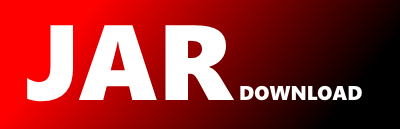
io.vertx.mutiny.ext.web.MIMEHeader Maven / Gradle / Ivy
The newest version!
package io.vertx.mutiny.ext.web;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import java.util.Map;
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.MIMEHeader.class)
public class MIMEHeader implements io.vertx.mutiny.ext.web.ParsedHeaderValue {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new MIMEHeader((io.vertx.ext.web.MIMEHeader) obj),
MIMEHeader::getDelegate
);
private final io.vertx.ext.web.MIMEHeader delegate;
public MIMEHeader(io.vertx.ext.web.MIMEHeader delegate) {
this.delegate = delegate;
}
public MIMEHeader(Object delegate) {
this.delegate = (io.vertx.ext.web.MIMEHeader)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
MIMEHeader() {
this.delegate = null;
}
public io.vertx.ext.web.MIMEHeader getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
MIMEHeader that = (MIMEHeader) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* @return
*/
public String rawValue() {
String ret = delegate.rawValue();
return ret;
}
/**
* @return
*/
public String value() {
String ret = delegate.value();
return ret;
}
/**
* @return
*/
public float weight() {
float ret = delegate.weight();
return ret;
}
/**
* @param key
* @return
*/
public String parameter(String key) {
String ret = delegate.parameter(key);
return ret;
}
/**
* @return Unmodifiable Map of parameters of this header value
*/
public java.util.Map parameters() {
java.util.Map ret = delegate.parameters();
return ret;
}
/**
* @return
*/
public boolean isPermitted() {
boolean ret = delegate.isPermitted();
return ret;
}
/**
* @param matchTry The header to be matched from
* @return true if this header represents a subset of matchTry, otherwise, false
*/
public boolean isMatchedBy(io.vertx.mutiny.ext.web.ParsedHeaderValue matchTry) {
boolean ret = delegate.isMatchedBy(matchTry.getDelegate());
return ret;
}
/**
* @return
*/
public int weightedOrder() {
int ret = delegate.weightedOrder();
return ret;
}
/**
* @return The component of the MIME this represents
*/
public String component() {
String ret = delegate.component();
return ret;
}
/**
* @return The subcomponent of the MIME this represents
*/
public String subComponent() {
String ret = delegate.subComponent();
return ret;
}
/**
* If no "q" parameter is present, the default weight is 1.
*/
public static final float DEFAULT_WEIGHT = io.vertx.ext.web.MIMEHeader.DEFAULT_WEIGHT;
public static MIMEHeader newInstance(io.vertx.ext.web.MIMEHeader arg) {
return arg != null ? new MIMEHeader(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy