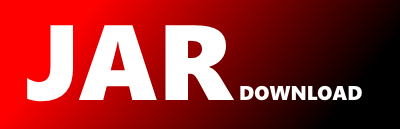
io.vertx.mutiny.ext.web.ParsedHeaderValue Maven / Gradle / Ivy
The newest version!
package io.vertx.mutiny.ext.web;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import java.util.Map;
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.ParsedHeaderValue.class)
public interface ParsedHeaderValue {
io.vertx.ext.web.ParsedHeaderValue getDelegate();
/**
* @return
*/
public String rawValue();
/**
* @return
*/
public String value();
/**
* @return
*/
public float weight();
/**
* @param key
* @return
*/
public String parameter(String key);
/**
* @return Unmodifiable Map of parameters of this header value
*/
public java.util.Map parameters();
/**
* @return
*/
public boolean isPermitted();
/**
* @param matchTry The header to be matched from
* @return true if this header represents a subset of matchTry, otherwise, false
*/
public boolean isMatchedBy(io.vertx.mutiny.ext.web.ParsedHeaderValue matchTry);
/**
* @return
*/
public int weightedOrder();
public static ParsedHeaderValue newInstance(io.vertx.ext.web.ParsedHeaderValue arg) {
return arg != null ? new ParsedHeaderValueImpl(arg) : null;
}
}
class ParsedHeaderValueImpl implements ParsedHeaderValue {
private final io.vertx.ext.web.ParsedHeaderValue delegate;
public io.vertx.ext.web.ParsedHeaderValue getDelegate() {
return delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
ParsedHeaderValueImpl() {
this.delegate = null;
}
public ParsedHeaderValueImpl(io.vertx.ext.web.ParsedHeaderValue delegate) {
this.delegate = delegate;
}
/**
* @return
*/
public String rawValue() {
String ret = delegate.rawValue();
return ret;
}
/**
* @return
*/
public String value() {
String ret = delegate.value();
return ret;
}
/**
* @return
*/
public float weight() {
float ret = delegate.weight();
return ret;
}
/**
* @param key
* @return
*/
public String parameter(String key) {
String ret = delegate.parameter(key);
return ret;
}
/**
* @return Unmodifiable Map of parameters of this header value
*/
public java.util.Map parameters() {
java.util.Map ret = delegate.parameters();
return ret;
}
/**
* @return
*/
public boolean isPermitted() {
boolean ret = delegate.isPermitted();
return ret;
}
/**
* @param matchTry The header to be matched from
* @return true if this header represents a subset of matchTry, otherwise, false
*/
public boolean isMatchedBy(io.vertx.mutiny.ext.web.ParsedHeaderValue matchTry) {
boolean ret = delegate.isMatchedBy(matchTry.getDelegate());
return ret;
}
/**
* @return
*/
public int weightedOrder() {
int ret = delegate.weightedOrder();
return ret;
}
/**
* If no "q" parameter is present, the default weight is 1.
*/
float DEFAULT_WEIGHT = io.vertx.ext.web.ParsedHeaderValue.DEFAULT_WEIGHT;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy