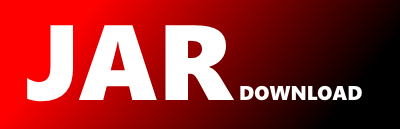
io.vertx.mutiny.ext.web.Router Maven / Gradle / Ivy
The newest version!
package io.vertx.mutiny.ext.web;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import java.util.List;
import io.vertx.core.http.HttpMethod;
import io.vertx.ext.web.AllowForwardHeaders;
import java.util.Map;
import io.vertx.core.Handler;
/**
* A router receives request from an {@link io.vertx.mutiny.core.http.HttpServer} and routes it to the first matching
* {@link io.vertx.mutiny.ext.web.Route} that it contains. A router can contain many routes.
*
* Routers are also used for routing failures.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.Router original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.Router.class)
public class Router implements io.vertx.core.Handler, java.util.function.Consumer {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new Router((io.vertx.ext.web.Router) obj),
Router::getDelegate
);
private final io.vertx.ext.web.Router delegate;
public Router(io.vertx.ext.web.Router delegate) {
this.delegate = delegate;
}
public Router(Object delegate) {
this.delegate = (io.vertx.ext.web.Router)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
Router() {
this.delegate = null;
}
public io.vertx.ext.web.Router getDelegate() {
return delegate;
}
static final io.smallrye.mutiny.vertx.TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)o1), o1 -> o1.getDelegate());
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Router that = (Router) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public void handle(io.vertx.mutiny.core.http.HttpServerRequest arg0) {
delegate.handle(arg0.getDelegate());
}
/**
* @param key the metadata of key
* @param value the metadata of value
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Router putMetadata(String key, java.lang.Object value) {
delegate.putMetadata(key, value);
return this;
}
/**
* @return the metadata of this router, never returns null.
*/
public java.util.Map metadata() {
java.util.Map ret = delegate.metadata();
return ret;
}
/**
* @param key the key for the metadata
* @return the data
*/
public T getMetadata(String key) {
T ret = (T) delegate.getMetadata(key);
return ret;
}
/**
* @param vertx the Vert.x instance
* @return the router
*/
public static io.vertx.mutiny.ext.web.Router router(io.vertx.mutiny.core.Vertx vertx) {
io.vertx.mutiny.ext.web.Router ret = io.vertx.mutiny.ext.web.Router.newInstance((io.vertx.ext.web.Router)io.vertx.ext.web.Router.router(vertx.getDelegate()));
return ret;
}
/**
* @return the route
*/
public io.vertx.mutiny.ext.web.Route route() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.route());
return ret;
}
/**
* @param method the HTTP method to match
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route route(io.vertx.core.http.HttpMethod method, String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.route(method, path));
return ret;
}
/**
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route route(String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.route(path));
return ret;
}
/**
* @param method the HTTP method to match
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route routeWithRegex(io.vertx.core.http.HttpMethod method, String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.routeWithRegex(method, regex));
return ret;
}
/**
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route routeWithRegex(String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.routeWithRegex(regex));
return ret;
}
/**
* @return the route
*/
public io.vertx.mutiny.ext.web.Route get() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.get());
return ret;
}
/**
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route get(String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.get(path));
return ret;
}
/**
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route getWithRegex(String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.getWithRegex(regex));
return ret;
}
/**
* @return the route
*/
public io.vertx.mutiny.ext.web.Route head() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.head());
return ret;
}
/**
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route head(String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.head(path));
return ret;
}
/**
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route headWithRegex(String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.headWithRegex(regex));
return ret;
}
/**
* @return the route
*/
public io.vertx.mutiny.ext.web.Route options() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.options());
return ret;
}
/**
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route options(String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.options(path));
return ret;
}
/**
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route optionsWithRegex(String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.optionsWithRegex(regex));
return ret;
}
/**
* @return the route
*/
public io.vertx.mutiny.ext.web.Route put() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.put());
return ret;
}
/**
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route put(String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.put(path));
return ret;
}
/**
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route putWithRegex(String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.putWithRegex(regex));
return ret;
}
/**
* @return the route
*/
public io.vertx.mutiny.ext.web.Route post() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.post());
return ret;
}
/**
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route post(String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.post(path));
return ret;
}
/**
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route postWithRegex(String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.postWithRegex(regex));
return ret;
}
/**
* @return the route
*/
public io.vertx.mutiny.ext.web.Route delete() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.delete());
return ret;
}
/**
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route delete(String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.delete(path));
return ret;
}
/**
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route deleteWithRegex(String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.deleteWithRegex(regex));
return ret;
}
/**
* @return the route
*/
public io.vertx.mutiny.ext.web.Route trace() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.trace());
return ret;
}
/**
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route trace(String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.trace(path));
return ret;
}
/**
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route traceWithRegex(String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.traceWithRegex(regex));
return ret;
}
/**
* @return the route
*/
public io.vertx.mutiny.ext.web.Route connect() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.connect());
return ret;
}
/**
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route connect(String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.connect(path));
return ret;
}
/**
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route connectWithRegex(String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.connectWithRegex(regex));
return ret;
}
/**
* @return the route
*/
public io.vertx.mutiny.ext.web.Route patch() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.patch());
return ret;
}
/**
* @param path URI paths that begin with this path will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route patch(String path) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.patch(path));
return ret;
}
/**
* @param regex URI paths that begin with a match for this regex will match
* @return the route
*/
public io.vertx.mutiny.ext.web.Route patchWithRegex(String regex) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.patchWithRegex(regex));
return ret;
}
/**
* @return a list of all the routes on this router
*/
public List getRoutes() {
List ret = delegate.getRoutes().stream().map(elt -> io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)elt)).collect(java.util.stream.Collectors.toList());
return ret;
}
/**
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Router clear() {
delegate.clear();
return this;
}
/**
* @param mountPoint the mount point (path prefix) to mount it on
* @param subRouter the router to mount as a sub router
* @return a reference to this, so the API can be used fluently
* @deprecated This method duplicates the sub router functionality from {@link Route#subRouter(Router)}. Mount a sub router on this router */
@Deprecated
public io.vertx.mutiny.ext.web.Route mountSubRouter(String mountPoint, io.vertx.mutiny.ext.web.Router subRouter) {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.mountSubRouter(mountPoint, subRouter.getDelegate()));
return ret;
}
/**
* @param statusCode status code the errorHandler is capable of handle
* @param errorHandler error handler. Note: You must not use {@link io.vertx.mutiny.ext.web.RoutingContext#next} inside the provided handler
* @return a reference to this, so the API can be used fluently
*/
@Fluent
private io.vertx.mutiny.ext.web.Router __errorHandler(int statusCode, Handler errorHandler) {
delegate.errorHandler(statusCode, new io.smallrye.mutiny.vertx.DelegatingHandler<>(errorHandler, event -> io.vertx.mutiny.ext.web.RoutingContext.newInstance((io.vertx.ext.web.RoutingContext)event)));
return this;
}
/**
* @param statusCode status code the errorHandler is capable of handle
* @param errorHandler error handler. Note: You must not use {@link io.vertx.mutiny.ext.web.RoutingContext#next} inside the provided handler
* @return
*/
public io.vertx.mutiny.ext.web.Router errorHandler(int statusCode, java.util.function.Consumer errorHandler) {
return __errorHandler(statusCode, errorHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(errorHandler) : null);
}
/**
* @param context the routing context
*/
public void handleContext(io.vertx.mutiny.ext.web.RoutingContext context) {
delegate.handleContext(context.getDelegate());
}
/**
* @param context the routing context
*/
public void handleFailure(io.vertx.mutiny.ext.web.RoutingContext context) {
delegate.handleFailure(context.getDelegate());
}
/**
* @param handler a notification handler that will receive this router as argument
* @return a reference to this, so the API can be used fluently
*/
@Fluent
private io.vertx.mutiny.ext.web.Router __modifiedHandler(Handler handler) {
delegate.modifiedHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(handler, event -> io.vertx.mutiny.ext.web.Router.newInstance((io.vertx.ext.web.Router)event)));
return this;
}
/**
* @param handler a notification handler that will receive this router as argument
* @return
*/
public io.vertx.mutiny.ext.web.Router modifiedHandler(java.util.function.Consumer handler) {
return __modifiedHandler(handler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(handler) : null);
}
/**
* @param allowForwardHeaders to enable parsing of "forwarded"-type headers
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Router allowForward(io.vertx.ext.web.AllowForwardHeaders allowForwardHeaders) {
delegate.allowForward(allowForwardHeaders);
return this;
}
public void accept(io.vertx.mutiny.core.http.HttpServerRequest item) {
handle(item);
}
public static Router newInstance(io.vertx.ext.web.Router arg) {
return arg != null ? new Router(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy