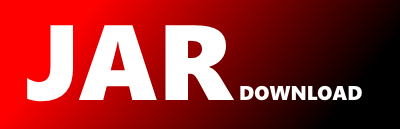
io.vertx.mutiny.ext.web.RoutingContext Maven / Gradle / Ivy
package io.vertx.mutiny.ext.web;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import java.nio.charset.Charset;
import java.util.Map;
import io.vertx.core.json.JsonObject;
import io.vertx.core.AsyncResult;
import java.time.Instant;
import io.vertx.core.Future;
import io.vertx.core.json.JsonArray;
import java.util.List;
import io.vertx.core.http.HttpMethod;
import io.vertx.core.Handler;
/**
* Represents the context for the handling of a request in Vert.x-Web.
*
* A new instance is created for each HTTP request that is received in the
* {@link io.vertx.core.Handler} of the router.
*
* The same instance is passed to any matching request or failure handlers during the routing of the request or
* failure.
*
* The context provides access to the and
* and allows you to maintain arbitrary data that lives for the lifetime of the context. Contexts are discarded once they
* have been routed to the handler for the request.
*
* The context also provides access to the {@link io.vertx.mutiny.ext.web.Session}, cookies and body for the request, given the correct handlers
* in the application.
*
* If you use the internal error handler
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.RoutingContext original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.RoutingContext.class)
public class RoutingContext {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new RoutingContext((io.vertx.ext.web.RoutingContext) obj),
RoutingContext::getDelegate
);
private final io.vertx.ext.web.RoutingContext delegate;
public RoutingContext(io.vertx.ext.web.RoutingContext delegate) {
this.delegate = delegate;
}
public RoutingContext(Object delegate) {
this.delegate = (io.vertx.ext.web.RoutingContext)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
RoutingContext() {
this.delegate = null;
}
public io.vertx.ext.web.RoutingContext getDelegate() {
return delegate;
}
static final io.smallrye.mutiny.vertx.TypeArg TYPE_ARG_1 = new TypeArg(o1 -> io.vertx.mutiny.ext.web.FileUpload.newInstance((io.vertx.ext.web.FileUpload)o1), o1 -> o1.getDelegate());
static final io.smallrye.mutiny.vertx.TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.mutiny.core.http.Cookie.newInstance((io.vertx.core.http.Cookie)o1), o1 -> o1.getDelegate());
static final io.smallrye.mutiny.vertx.TypeArg TYPE_ARG_2 = new TypeArg(o1 -> io.vertx.mutiny.ext.web.LanguageHeader.newInstance((io.vertx.ext.web.LanguageHeader)o1), o1 -> o1.getDelegate());
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
RoutingContext that = (RoutingContext) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* @return the HTTP request object
*/
public io.vertx.mutiny.core.http.HttpServerRequest request() {
if (cached_0 != null) {
return cached_0;
}
io.vertx.mutiny.core.http.HttpServerRequest ret = io.vertx.mutiny.core.http.HttpServerRequest.newInstance((io.vertx.core.http.HttpServerRequest)delegate.request());
cached_0 = ret;
return ret;
}
/**
* @return the HTTP response object
*/
public io.vertx.mutiny.core.http.HttpServerResponse response() {
if (cached_1 != null) {
return cached_1;
}
io.vertx.mutiny.core.http.HttpServerResponse ret = io.vertx.mutiny.core.http.HttpServerResponse.newInstance((io.vertx.core.http.HttpServerResponse)delegate.response());
cached_1 = ret;
return ret;
}
/**
*/
public void next() {
delegate.next();
}
/**
* @param statusCode the HTTP status code
*/
public void fail(int statusCode) {
delegate.fail(statusCode);
}
/**
* @param throwable a throwable representing the failure
*/
public void fail(java.lang.Throwable throwable) {
delegate.fail(throwable);
}
/**
* @param statusCode the HTTP status code
* @param throwable a throwable representing the failure
*/
public void fail(int statusCode, java.lang.Throwable throwable) {
delegate.fail(statusCode, throwable);
}
/**
* @param key the key for the data
* @param obj the data
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext put(String key, java.lang.Object obj) {
delegate.put(key, obj);
return this;
}
/**
* @param key the key for the data
* @return the data
*/
public T get(String key) {
T ret = (T) delegate.get(key);
return ret;
}
/**
* @param key the key for the data
* @param defaultValue when the underlying data doesn't contain the key this will be the return value.
* @return the data
*/
public T get(String key, T defaultValue) {
T ret = (T) delegate.get(key, defaultValue);
return ret;
}
/**
* @param key the key for the data
* @return the previous data associated with the key
*/
public T remove(String key) {
T ret = (T) delegate.remove(key);
return ret;
}
/**
* @return the Vert.x instance associated to the initiating {@link io.vertx.mutiny.ext.web.Router} for this context
*/
public io.vertx.mutiny.core.Vertx vertx() {
if (cached_2 != null) {
return cached_2;
}
io.vertx.mutiny.core.Vertx ret = io.vertx.mutiny.core.Vertx.newInstance((io.vertx.core.Vertx)delegate.vertx());
cached_2 = ret;
return ret;
}
/**
* @return the mount point for this router. It will be null for a top level router. For a sub-router it will be the path at which the subrouter was mounted.
*/
public String mountPoint() {
String ret = delegate.mountPoint();
return ret;
}
/**
* @return the current route this context is being routed through.
*/
public io.vertx.mutiny.ext.web.Route currentRoute() {
io.vertx.mutiny.ext.web.Route ret = io.vertx.mutiny.ext.web.Route.newInstance((io.vertx.ext.web.Route)delegate.currentRoute());
return ret;
}
/**
* @return
* @deprecated */
@Deprecated
public String normalisedPath() {
String ret = delegate.normalisedPath();
return ret;
}
/**
* @return the normalized path
*/
public String normalizedPath() {
String ret = delegate.normalizedPath();
return ret;
}
/**
* @param name the cookie name
* @return the cookie
* @deprecated Use {@link HttpServerRequest#getCookie(String)} Get the cookie with the specified name. */
@Deprecated
public io.vertx.mutiny.core.http.Cookie getCookie(String name) {
io.vertx.mutiny.core.http.Cookie ret = io.vertx.mutiny.core.http.Cookie.newInstance((io.vertx.core.http.Cookie)delegate.getCookie(name));
return ret;
}
/**
* @param cookie the cookie
* @return a reference to this, so the API can be used fluently
* @deprecated Use {@link HttpServerResponse#addCookie(Cookie)} Add a cookie. This will be sent back to the client in the response. */
@Deprecated
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext addCookie(io.vertx.mutiny.core.http.Cookie cookie) {
delegate.addCookie(cookie.getDelegate());
return this;
}
/**
* @param name the name of the cookie
* @return the cookie, if it existed, or null
* @deprecated Use {@link HttpServerResponse#removeCookie(String)} Expire a cookie, notifying a User Agent to remove it from its cookie jar. */
@Deprecated
public io.vertx.mutiny.core.http.Cookie removeCookie(String name) {
io.vertx.mutiny.core.http.Cookie ret = io.vertx.mutiny.core.http.Cookie.newInstance((io.vertx.core.http.Cookie)delegate.removeCookie(name));
return ret;
}
/**
* @param name the name of the cookie
* @param invalidate
* @return the cookie, if it existed, or null
* @deprecated Use {@link HttpServerResponse#removeCookie(String, boolean)} Remove a cookie from the cookie set. If invalidate is true then it will expire a cookie, notifying a User Agent to remove it from its cookie jar. */
@Deprecated
public io.vertx.mutiny.core.http.Cookie removeCookie(String name, boolean invalidate) {
io.vertx.mutiny.core.http.Cookie ret = io.vertx.mutiny.core.http.Cookie.newInstance((io.vertx.core.http.Cookie)delegate.removeCookie(name, invalidate));
return ret;
}
/**
* @return the number of cookies.
* @deprecated Use {@link HttpServerRequest#cookieCount()} */
@Deprecated
public int cookieCount() {
int ret = delegate.cookieCount();
return ret;
}
/**
* @return a map of all the cookies.
* @deprecated Use {@link HttpServerRequest#cookieMap()} */
@Deprecated
public java.util.Map cookieMap() {
java.util.Map ret = delegate.cookieMap().entrySet().stream().collect(Collectors.toMap(_e -> _e.getKey(), _e -> io.vertx.mutiny.core.http.Cookie.newInstance((io.vertx.core.http.Cookie)_e.getValue())));
return ret;
}
/**
* @return the entire HTTP request body as a string, assuming UTF-8 encoding if the request does not provide the content type charset attribute. If a charset is provided in the request that it shall be respected. The context must have first been routed to a {@link io.vertx.mutiny.ext.web.handler.BodyHandler} for this to be populated.
* @deprecated Use {@link #body()} instead. */
@Deprecated
public String getBodyAsString() {
String ret = delegate.getBodyAsString();
return ret;
}
/**
* @param encoding the encoding, e.g. "UTF-16"
* @return the body
* @deprecated Use {@link #body()} instead. Get the entire HTTP request body as a string, assuming the specified encoding. The context must have first been routed to a {@link io.vertx.ext.web.handler.BodyHandler} for this to be populated. */
@Deprecated
public String getBodyAsString(String encoding) {
String ret = delegate.getBodyAsString(encoding);
return ret;
}
/**
* @param maxAllowedLength if the current buffer length is greater than the limit an {@link java.lang.IllegalStateException} is thrown. This can be used to avoid DDoS attacks on very long JSON payloads that could take over the CPU while attempting to parse the data.
* @return Get the entire HTTP request body as a . The context must have first been routed to a {@link io.vertx.mutiny.ext.web.handler.BodyHandler} for this to be populated.
When the body is null
or the "null"
JSON literal then null
is returned.
* @deprecated Use {@link #body()} instead. Gets the current body buffer as a {@link JsonObject}. If a positive limit is provided the parsing will only happen if the buffer length is smaller or equal to the limit. Otherwise an {@link IllegalStateException} is thrown. When the application is only handling uploads in JSON format, it is recommended to set a limit on {@link io.vertx.ext.web.handler.BodyHandler#setBodyLimit(long)} as this will avoid the upload to be parsed and loaded into the application memory. */
@Deprecated
public JsonObject getBodyAsJson(int maxAllowedLength) {
JsonObject ret = delegate.getBodyAsJson(maxAllowedLength);
return ret;
}
/**
* @param maxAllowedLength if the current buffer length is greater than the limit an {@link java.lang.IllegalStateException} is thrown. This can be used to avoid DDoS attacks on very long JSON payloads that could take over the CPU while attempting to parse the data.
* @return Get the entire HTTP request body as a . The context must have first been routed to a {@link io.vertx.mutiny.ext.web.handler.BodyHandler} for this to be populated.
When the body is null
or the "null"
JSON literal then null
is returned.
* @deprecated Use {@link #body()} instead. Gets the current body buffer as a {@link JsonArray}. If a positive limit is provided the parsing will only happen if the buffer length is smaller or equal to the limit. Otherwise an {@link IllegalStateException} is thrown. When the application is only handling uploads in JSON format, it is recommended to set a limit on {@link io.vertx.ext.web.handler.BodyHandler#setBodyLimit(long)} as this will avoid the upload to be parsed and loaded into the application memory. */
@Deprecated
public JsonArray getBodyAsJsonArray(int maxAllowedLength) {
JsonArray ret = delegate.getBodyAsJsonArray(maxAllowedLength);
return ret;
}
/**
* @return Get the entire HTTP request body as a . The context must have first been routed to a {@link io.vertx.mutiny.ext.web.handler.BodyHandler} for this to be populated.
When the body is null
or the "null"
JSON literal then null
is returned.
* @deprecated Use {@link #body()} instead. */
@Deprecated
public JsonObject getBodyAsJson() {
JsonObject ret = delegate.getBodyAsJson();
return ret;
}
/**
* @return Get the entire HTTP request body as a . The context must have first been routed to a {@link io.vertx.mutiny.ext.web.handler.BodyHandler} for this to be populated.
When the body is null
or the "null"
JSON literal then null
is returned.
* @deprecated Use {@link #body()} instead. */
@Deprecated
public JsonArray getBodyAsJsonArray() {
JsonArray ret = delegate.getBodyAsJsonArray();
return ret;
}
/**
* @return Get the entire HTTP request body as a . The context must have first been routed to a {@link io.vertx.mutiny.ext.web.handler.BodyHandler} for this to be populated.
* @deprecated Use {@link #body()} instead. */
@Deprecated
public io.vertx.mutiny.core.buffer.Buffer getBody() {
io.vertx.mutiny.core.buffer.Buffer ret = io.vertx.mutiny.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)delegate.getBody());
return ret;
}
public io.vertx.mutiny.ext.web.RequestBody body() {
io.vertx.mutiny.ext.web.RequestBody ret = io.vertx.mutiny.ext.web.RequestBody.newInstance((io.vertx.ext.web.RequestBody)delegate.body());
return ret;
}
/**
* @return a list of {@link io.vertx.mutiny.ext.web.FileUpload} (if any) for the request. The context must have first been routed to a {@link io.vertx.mutiny.ext.web.handler.BodyHandler} for this to work.
*/
public List fileUploads() {
List ret = delegate.fileUploads().stream().map(elt -> io.vertx.mutiny.ext.web.FileUpload.newInstance((io.vertx.ext.web.FileUpload)elt)).collect(java.util.stream.Collectors.toList());
return ret;
}
/**
*/
public void cancelAndCleanupFileUploads() {
delegate.cancelAndCleanupFileUploads();
}
/**
* @return the session.
*/
public io.vertx.mutiny.ext.web.Session session() {
io.vertx.mutiny.ext.web.Session ret = io.vertx.mutiny.ext.web.Session.newInstance((io.vertx.ext.web.Session)delegate.session());
return ret;
}
/**
* @return true if the session has been accessed.
*/
public boolean isSessionAccessed() {
boolean ret = delegate.isSessionAccessed();
return ret;
}
/**
* @return the user, or null if the current user is not authenticated.
*/
public io.vertx.mutiny.ext.auth.User user() {
io.vertx.mutiny.ext.auth.User ret = io.vertx.mutiny.ext.auth.User.newInstance((io.vertx.ext.auth.User)delegate.user());
return ret;
}
/**
* @return the throwable used when signalling failure
*/
public java.lang.Throwable failure() {
if (cached_3 != null) {
return cached_3;
}
java.lang.Throwable ret = delegate.failure();
cached_3 = ret;
return ret;
}
/**
* @return the status code used when signalling failure
*/
public int statusCode() {
if (cached_4 != null) {
return cached_4;
}
int ret = delegate.statusCode();
cached_4 = ret;
return ret;
}
/**
* @return the most acceptable content type.
*/
public String getAcceptableContentType() {
String ret = delegate.getAcceptableContentType();
return ret;
}
/**
* @return A container with the parsed headers.
*/
public io.vertx.mutiny.ext.web.ParsedHeaderValues parsedHeaders() {
if (cached_5 != null) {
return cached_5;
}
io.vertx.mutiny.ext.web.ParsedHeaderValues ret = io.vertx.mutiny.ext.web.ParsedHeaderValues.newInstance((io.vertx.ext.web.ParsedHeaderValues)delegate.parsedHeaders());
cached_5 = ret;
return ret;
}
/**
* @param handler the handler
* @return the id of the handler. This can be used if you later want to remove the handler.
*/
private int __addHeadersEndHandler(Handler handler) {
int ret = delegate.addHeadersEndHandler(handler);
return ret;
}
/**
* @param handler the handler
* @return
*/
public int addHeadersEndHandler(java.lang.Runnable handler) {
return __addHeadersEndHandler(ignored -> handler.run()
);
}
/**
* @param handlerID the id as returned from {@link io.vertx.mutiny.ext.web.RoutingContext#addHeadersEndHandler}.
* @return true if the handler existed and was removed, false otherwise
*/
public boolean removeHeadersEndHandler(int handlerID) {
boolean ret = delegate.removeHeadersEndHandler(handlerID);
return ret;
}
/**
* @param handler the handler
* @return the id of the handler. This can be used if you later want to remove the handler.
*/
private int __addBodyEndHandler(Handler handler) {
int ret = delegate.addBodyEndHandler(handler);
return ret;
}
/**
* @param handler the handler
* @return
*/
public int addBodyEndHandler(java.lang.Runnable handler) {
return __addBodyEndHandler(ignored -> handler.run()
);
}
/**
* @param handlerID the id as returned from {@link io.vertx.mutiny.ext.web.RoutingContext#addBodyEndHandler}.
* @return true if the handler existed and was removed, false otherwise
*/
public boolean removeBodyEndHandler(int handlerID) {
boolean ret = delegate.removeBodyEndHandler(handlerID);
return ret;
}
/**
* Add an end handler for the request/response context. This will be called when the response is disposed or an
* exception has been encountered to allow consistent cleanup. The handler is called asynchronously of when the
* response has been received by the client.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni addEndHandler() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.addEndHandler(handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.RoutingContext#addEndHandler}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Void instance produced by the operation.
*/
public Void addEndHandlerAndAwait() {
return (Void) addEndHandler().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.RoutingContext#addEndHandler} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.RoutingContext#addEndHandler}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.RoutingContext#addEndHandler} but you don't need to compose it with other operations.
*/
public void addEndHandlerAndForget() {
addEndHandler().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
}
/**
* @param handlerID the id as returned from {@link io.vertx.mutiny.ext.web.RoutingContext#addEndHandler}.
* @return true if the handler existed and was removed, false otherwise
*/
public boolean removeEndHandler(int handlerID) {
boolean ret = delegate.removeEndHandler(handlerID);
return ret;
}
/**
* @return true if the context is being routed to failure handlers.
*/
public boolean failed() {
boolean ret = delegate.failed();
return ret;
}
/**
* @param body the body
* @deprecated This method is internal. Users that really need to use it should refer to {@link io.vertx.ext.web.impl.RoutingContextInternal#setBody(Buffer)} Set the body. Used by the {@link io.vertx.ext.web.handler.BodyHandler}. You will not normally call this method. */
@Deprecated
public void setBody(io.vertx.mutiny.core.buffer.Buffer body) {
delegate.setBody(body.getDelegate());
}
/**
* @param session the session
* @deprecated This method is internal. Users that really need to use it should refer to {@link io.vertx.ext.web.impl.RoutingContextInternal#setSession(Session)} Set the session. Used by the {@link io.vertx.ext.web.handler.SessionHandler}. You will not normally call this method. */
@Deprecated
public void setSession(io.vertx.mutiny.ext.web.Session session) {
delegate.setSession(session.getDelegate());
}
/**
* @param user the user
*/
public void setUser(io.vertx.mutiny.ext.auth.User user) {
delegate.setUser(user.getDelegate());
}
/**
*/
public void clearUser() {
delegate.clearUser();
}
/**
* @param contentType the content type
*/
public void setAcceptableContentType(String contentType) {
delegate.setAcceptableContentType(contentType);
}
/**
* @param path the new http path.
*/
public void reroute(String path) {
delegate.reroute(path);
}
/**
* @param method the new http request
* @param path the new http path.
*/
public void reroute(io.vertx.core.http.HttpMethod method, String path) {
delegate.reroute(method, path);
}
/**
* @return The best matched language for the request
*/
public List acceptableLanguages() {
if (cached_6 != null) {
return cached_6;
}
List ret = delegate.acceptableLanguages().stream().map(elt -> io.vertx.mutiny.ext.web.LanguageHeader.newInstance((io.vertx.ext.web.LanguageHeader)elt)).collect(java.util.stream.Collectors.toList());
cached_6 = ret;
return ret;
}
/**
* @return the users preferred locale.
*/
public io.vertx.mutiny.ext.web.LanguageHeader preferredLanguage() {
if (cached_7 != null) {
return cached_7;
}
io.vertx.mutiny.ext.web.LanguageHeader ret = io.vertx.mutiny.ext.web.LanguageHeader.newInstance((io.vertx.ext.web.LanguageHeader)delegate.preferredLanguage());
cached_7 = ret;
return ret;
}
/**
* @return the map of named parameters
*/
public java.util.Map pathParams() {
java.util.Map ret = delegate.pathParams();
return ret;
}
/**
* @param name the name of parameter as defined in path declaration
* @return the actual value of the parameter or null if it doesn't exist
*/
public String pathParam(String name) {
String ret = delegate.pathParam(name);
return ret;
}
/**
* @return the multimap of query parameters
*/
public io.vertx.mutiny.core.MultiMap queryParams() {
io.vertx.mutiny.core.MultiMap ret = io.vertx.mutiny.core.MultiMap.newInstance((io.vertx.core.MultiMap)delegate.queryParams());
return ret;
}
/**
* @param name The name of query parameter
* @return The list of all parameters matching the parameter name. It returns an empty list if no query parameter with name
was found
*/
public List queryParam(String name) {
List ret = delegate.queryParam(name);
return ret;
}
/**
* @param filename the filename for the attachment
* @return the instance of RoutingContext to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext attachment(String filename) {
delegate.attachment(filename);
return this;
}
/**
* See {@link io.vertx.mutiny.ext.web.RoutingContext#redirect}.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param url
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni redirect(String url) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.redirect(url, handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.RoutingContext#redirect(String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param url
* @return the Void instance produced by the operation.
*/
public Void redirectAndAwait(String url) {
return (Void) redirect(url).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.RoutingContext#redirect(String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.RoutingContext#redirect(String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.RoutingContext#redirect(String)} but you don't need to compose it with other operations.
* @param url
* @return the instance of RoutingContext to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext redirectAndForget(String url) {
redirect(url).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* See {@link io.vertx.mutiny.ext.web.RoutingContext#json}.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param json
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni json(java.lang.Object json) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.json(json, handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.RoutingContext#json(Object)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param json
* @return the Void instance produced by the operation.
*/
public Void jsonAndAwait(java.lang.Object json) {
return (Void) json(json).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.RoutingContext#json(Object)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.RoutingContext#json(Object)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.RoutingContext#json(Object)} but you don't need to compose it with other operations.
* @param json
* @return the instance of RoutingContext to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext jsonAndForget(java.lang.Object json) {
json(json).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* @param type content type
* @return The most close value
*/
public boolean is(String type) {
if (cached_8 != null) {
return cached_8;
}
boolean ret = delegate.is(type);
cached_8 = ret;
return ret;
}
/**
* @return true if content is fresh according to the cache.
*/
public boolean isFresh() {
boolean ret = delegate.isFresh();
return ret;
}
/**
* @param etag the etag value
* @return the instance of RoutingContext to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext etag(String etag) {
delegate.etag(etag);
return this;
}
/**
* @param instant the last modified instant
* @return the instance of RoutingContext to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext lastModified(String instant) {
delegate.lastModified(instant);
return this;
}
/**
* See {@link io.vertx.mutiny.ext.web.RoutingContext#end}
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param chunk
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni end(String chunk) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.end(chunk, handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.RoutingContext#end(String)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param chunk
* @return the Void instance produced by the operation.
*/
public Void endAndAwait(String chunk) {
return (Void) end(chunk).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.RoutingContext#end(String)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.RoutingContext#end(String)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.RoutingContext#end(String)} but you don't need to compose it with other operations.
* @param chunk
* @return the instance of RoutingContext to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext endAndForget(String chunk) {
end(chunk).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* See {@link io.vertx.mutiny.ext.web.RoutingContext#end}
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param buffer
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni end(io.vertx.mutiny.core.buffer.Buffer buffer) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.end(buffer.getDelegate(), handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.RoutingContext#end(io.vertx.mutiny.core.buffer.Buffer)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param buffer
* @return the Void instance produced by the operation.
*/
public Void endAndAwait(io.vertx.mutiny.core.buffer.Buffer buffer) {
return (Void) end(buffer).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.RoutingContext#end(io.vertx.mutiny.core.buffer.Buffer)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.RoutingContext#end(io.vertx.mutiny.core.buffer.Buffer)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.RoutingContext#end(io.vertx.mutiny.core.buffer.Buffer)} but you don't need to compose it with other operations.
* @param buffer
* @return the instance of RoutingContext to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext endAndForget(io.vertx.mutiny.core.buffer.Buffer buffer) {
end(buffer).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* See {@link io.vertx.mutiny.ext.web.RoutingContext#end}
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni end() {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.end(handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.RoutingContext#end}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @return the Void instance produced by the operation.
*/
public Void endAndAwait() {
return (Void) end().await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.RoutingContext#end} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.RoutingContext#end}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.RoutingContext#end} but you don't need to compose it with other operations.
* @return the instance of RoutingContext to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext endAndForget() {
end().subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* @return all the context data as a map
*/
public java.util.Map data() {
java.util.Map ret = delegate.data();
return ret;
}
/**
* @param encoding a non null character set.
* @return the multimap of query parameters
*/
public io.vertx.mutiny.core.MultiMap queryParams(java.nio.charset.Charset encoding) {
io.vertx.mutiny.core.MultiMap ret = io.vertx.mutiny.core.MultiMap.newInstance((io.vertx.core.MultiMap)delegate.queryParams(encoding));
return ret;
}
/**
* @param instant the last modified instant
* @return the instance of RoutingContext to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.RoutingContext lastModified(java.time.Instant instant) {
delegate.lastModified(instant);
return this;
}
private io.vertx.mutiny.core.http.HttpServerRequest cached_0;
private io.vertx.mutiny.core.http.HttpServerResponse cached_1;
private io.vertx.mutiny.core.Vertx cached_2;
private java.lang.Throwable cached_3;
private java.lang.Integer cached_4;
private io.vertx.mutiny.ext.web.ParsedHeaderValues cached_5;
private List cached_6;
private io.vertx.mutiny.ext.web.LanguageHeader cached_7;
private java.lang.Boolean cached_8;
public static RoutingContext newInstance(io.vertx.ext.web.RoutingContext arg) {
return arg != null ? new RoutingContext(arg) : null;
}
}