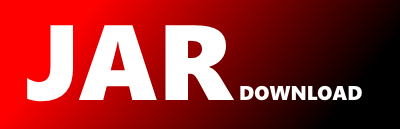
io.vertx.mutiny.ext.web.handler.SessionHandler Maven / Gradle / Ivy
package io.vertx.mutiny.ext.web.handler;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import io.vertx.core.http.CookieSameSite;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
import io.vertx.core.Future;
/**
* A handler that maintains a {@link io.vertx.mutiny.ext.web.Session} for each browser
* session.
*
* It looks up the session for each request based on a session cookie which
* contains a session ID. It stores the session when the response is ended in
* the session store.
*
* The session is available on the routing context with
* .
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.handler.SessionHandler original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.handler.SessionHandler.class)
public class SessionHandler implements io.vertx.mutiny.ext.web.handler.PlatformHandler, io.vertx.core.Handler, java.util.function.Consumer {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new SessionHandler((io.vertx.ext.web.handler.SessionHandler) obj),
SessionHandler::getDelegate
);
private final io.vertx.ext.web.handler.SessionHandler delegate;
public SessionHandler(io.vertx.ext.web.handler.SessionHandler delegate) {
this.delegate = delegate;
}
public SessionHandler(Object delegate) {
this.delegate = (io.vertx.ext.web.handler.SessionHandler)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
SessionHandler() {
this.delegate = null;
}
public io.vertx.ext.web.handler.SessionHandler getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SessionHandler that = (SessionHandler) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public void handle(io.vertx.mutiny.ext.web.RoutingContext arg0) {
delegate.handle(arg0.getDelegate());
}
/**
* @param sessionStore the session store
* @return the handler
*/
public static io.vertx.mutiny.ext.web.handler.SessionHandler create(io.vertx.mutiny.ext.web.sstore.SessionStore sessionStore) {
io.vertx.mutiny.ext.web.handler.SessionHandler ret = io.vertx.mutiny.ext.web.handler.SessionHandler.newInstance((io.vertx.ext.web.handler.SessionHandler)io.vertx.ext.web.handler.SessionHandler.create(sessionStore.getDelegate()));
return ret;
}
/**
* @param timeout the timeout, in ms.
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setSessionTimeout(long timeout) {
delegate.setSessionTimeout(timeout);
return this;
}
/**
* @param nag true to nag
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setNagHttps(boolean nag) {
delegate.setNagHttps(nag);
return this;
}
/**
* @param secure true to set the secure flag on the cookie
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setCookieSecureFlag(boolean secure) {
delegate.setCookieSecureFlag(secure);
return this;
}
/**
* @param httpOnly true to set the HttpOnly flag on the cookie
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setCookieHttpOnlyFlag(boolean httpOnly) {
delegate.setCookieHttpOnlyFlag(httpOnly);
return this;
}
/**
* @param sessionCookieName the session cookie name
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setSessionCookieName(String sessionCookieName) {
delegate.setSessionCookieName(sessionCookieName);
return this;
}
/**
* @param sessionCookiePath the session cookie path
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setSessionCookiePath(String sessionCookiePath) {
delegate.setSessionCookiePath(sessionCookiePath);
return this;
}
/**
* @param minLength the session id minimal length
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setMinLength(int minLength) {
delegate.setMinLength(minLength);
return this;
}
/**
* @param policy to use, null
for no policy.
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setCookieSameSite(io.vertx.core.http.CookieSameSite policy) {
delegate.setCookieSameSite(policy);
return this;
}
/**
* @param lazySession true to have a lazy session creation.
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setLazySession(boolean lazySession) {
delegate.setLazySession(lazySession);
return this;
}
/**
* @param cookieMaxAge a non negative max-age, note that 0 means expire now.
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setCookieMaxAge(long cookieMaxAge) {
delegate.setCookieMaxAge(cookieMaxAge);
return this;
}
/**
* Flush a context session earlier to the store, this will allow the end user to have full control on the event of
* a failure at the store level. Once a session is flushed no automatic save will be performed at end of request.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param ctx the current context
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni flush(io.vertx.mutiny.ext.web.RoutingContext ctx) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.flush(ctx.getDelegate(), handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.handler.SessionHandler#flush(io.vertx.mutiny.ext.web.RoutingContext)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param ctx the current context
* @return the Void instance produced by the operation.
*/
public Void flushAndAwait(io.vertx.mutiny.ext.web.RoutingContext ctx) {
return (Void) flush(ctx).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.handler.SessionHandler#flush(io.vertx.mutiny.ext.web.RoutingContext)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.handler.SessionHandler#flush(io.vertx.mutiny.ext.web.RoutingContext)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.handler.SessionHandler#flush(io.vertx.mutiny.ext.web.RoutingContext)} but you don't need to compose it with other operations.
* @param ctx the current context
* @return the instance of SessionHandler to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler flushAndForget(io.vertx.mutiny.ext.web.RoutingContext ctx) {
flush(ctx).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Flush a context session earlier to the store, this will allow the end user to have full control on the event of
* a failure at the store level. Once a session is flushed no automatic save will be performed at end of request.
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param ctx the current context
* @param ignoreStatus flush regardless of response status code
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni flush(io.vertx.mutiny.ext.web.RoutingContext ctx, boolean ignoreStatus) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.flush(ctx.getDelegate(), ignoreStatus, handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.handler.SessionHandler#flush(io.vertx.mutiny.ext.web.RoutingContext,boolean)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param ctx the current context
* @param ignoreStatus flush regardless of response status code
* @return the Void instance produced by the operation.
*/
public Void flushAndAwait(io.vertx.mutiny.ext.web.RoutingContext ctx, boolean ignoreStatus) {
return (Void) flush(ctx, ignoreStatus).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.handler.SessionHandler#flush(io.vertx.mutiny.ext.web.RoutingContext,boolean)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.handler.SessionHandler#flush(io.vertx.mutiny.ext.web.RoutingContext,boolean)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.handler.SessionHandler#flush(io.vertx.mutiny.ext.web.RoutingContext,boolean)} but you don't need to compose it with other operations.
* @param ctx the current context
* @param ignoreStatus flush regardless of response status code
* @return the instance of SessionHandler to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler flushAndForget(io.vertx.mutiny.ext.web.RoutingContext ctx, boolean ignoreStatus) {
flush(ctx, ignoreStatus).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* @param cookieless true if a cookieless session should be used
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setCookieless(boolean cookieless) {
delegate.setCookieless(cookieless);
return this;
}
/**
* @param context the routing context
* @return the session
*/
public io.vertx.mutiny.ext.web.Session newSession(io.vertx.mutiny.ext.web.RoutingContext context) {
io.vertx.mutiny.ext.web.Session ret = io.vertx.mutiny.ext.web.Session.newInstance((io.vertx.ext.web.Session)delegate.newSession(context.getDelegate()));
return ret;
}
/**
* Set the user for the session
*
* Unlike the bare Vert.x variant, this method returns a {@link io.smallrye.mutiny.Uni Uni}.
* Don't forget to subscribe on it to trigger the operation.
* @param context the routing context
* @param user the user
* @return the {@link io.smallrye.mutiny.Uni uni} firing the result of the operation when completed, or a failure if the operation failed.
*/
@CheckReturnValue
public io.smallrye.mutiny.Uni setUser(io.vertx.mutiny.ext.web.RoutingContext context, io.vertx.mutiny.ext.auth.User user) {
return io.smallrye.mutiny.vertx.AsyncResultUni.toUni(handler -> {
delegate.setUser(context.getDelegate(), user.getDelegate(), handler);
});
}
/**
* Blocking variant of {@link io.vertx.mutiny.ext.web.handler.SessionHandler#setUser(io.vertx.mutiny.ext.web.RoutingContext,io.vertx.mutiny.ext.auth.User)}.
*
* This method waits for the completion of the underlying asynchronous operation.
* If the operation completes successfully, the result is returned, otherwise the failure is thrown (potentially wrapped in a RuntimeException).
* @param context the routing context
* @param user the user
* @return the Void instance produced by the operation.
*/
public Void setUserAndAwait(io.vertx.mutiny.ext.web.RoutingContext context, io.vertx.mutiny.ext.auth.User user) {
return (Void) setUser(context, user).await().indefinitely();
}
/**
* Variant of {@link io.vertx.mutiny.ext.web.handler.SessionHandler#setUser(io.vertx.mutiny.ext.web.RoutingContext,io.vertx.mutiny.ext.auth.User)} that ignores the result of the operation.
*
* This method subscribes on the result of {@link io.vertx.mutiny.ext.web.handler.SessionHandler#setUser(io.vertx.mutiny.ext.web.RoutingContext,io.vertx.mutiny.ext.auth.User)}, but discards the outcome (item or failure).
* This method is useful to trigger the asynchronous operation from {@link io.vertx.mutiny.ext.web.handler.SessionHandler#setUser(io.vertx.mutiny.ext.web.RoutingContext,io.vertx.mutiny.ext.auth.User)} but you don't need to compose it with other operations.
* @param context the routing context
* @param user the user
* @return the instance of SessionHandler to chain method calls.
*/
@Fluent
public io.vertx.mutiny.ext.web.handler.SessionHandler setUserAndForget(io.vertx.mutiny.ext.web.RoutingContext context, io.vertx.mutiny.ext.auth.User user) {
setUser(context, user).subscribe().with(io.smallrye.mutiny.vertx.UniHelper.NOOP);
return this;
}
/**
* Default name of session cookie
*/
public static final String DEFAULT_SESSION_COOKIE_NAME = io.vertx.ext.web.handler.SessionHandler.DEFAULT_SESSION_COOKIE_NAME;
/**
* Default path of session cookie
*/
public static final String DEFAULT_SESSION_COOKIE_PATH = io.vertx.ext.web.handler.SessionHandler.DEFAULT_SESSION_COOKIE_PATH;
/**
* Default time, in ms, that a session lasts for without being accessed before
* expiring.
*/
public static final long DEFAULT_SESSION_TIMEOUT = io.vertx.ext.web.handler.SessionHandler.DEFAULT_SESSION_TIMEOUT;
/**
* Default of whether a nagging log warning should be written if the session
* handler is accessed over HTTP, not HTTPS
*/
public static final boolean DEFAULT_NAG_HTTPS = io.vertx.ext.web.handler.SessionHandler.DEFAULT_NAG_HTTPS;
/**
* Default of whether the cookie has the HttpOnly flag set More info:
* https://www.owasp.org/index.php/HttpOnly
*/
public static final boolean DEFAULT_COOKIE_HTTP_ONLY_FLAG = io.vertx.ext.web.handler.SessionHandler.DEFAULT_COOKIE_HTTP_ONLY_FLAG;
/**
* Default of whether the cookie has the 'secure' flag set to allow transmission
* over https only. More info: https://www.owasp.org/index.php/SecureFlag
*/
public static final boolean DEFAULT_COOKIE_SECURE_FLAG = io.vertx.ext.web.handler.SessionHandler.DEFAULT_COOKIE_SECURE_FLAG;
/**
* Default min length for a session id. More info:
* https://www.owasp.org/index.php/Session_Management_Cheat_Sheet
*/
public static final int DEFAULT_SESSIONID_MIN_LENGTH = io.vertx.ext.web.handler.SessionHandler.DEFAULT_SESSIONID_MIN_LENGTH;
/**
* Default of whether the session should be created lazily.
*/
public static final boolean DEFAULT_LAZY_SESSION = io.vertx.ext.web.handler.SessionHandler.DEFAULT_LAZY_SESSION;
public void accept(io.vertx.mutiny.ext.web.RoutingContext item) {
handle(item);
}
public static SessionHandler newInstance(io.vertx.ext.web.handler.SessionHandler arg) {
return arg != null ? new SessionHandler(arg) : null;
}
}