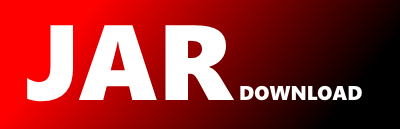
io.vertx.mutiny.ext.web.handler.sockjs.SockJSHandler Maven / Gradle / Ivy
The newest version!
package io.vertx.mutiny.ext.web.handler.sockjs;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import io.vertx.ext.web.handler.sockjs.SockJSBridgeOptions;
import io.vertx.core.Handler;
import io.vertx.ext.web.handler.sockjs.SockJSHandlerOptions;
/**
*
* A handler that allows you to handle SockJS connections from clients.
*
* We currently support version 0.3.3 of the SockJS protocol, which can be found in
* this tag:
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.handler.sockjs.SockJSHandler original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.handler.sockjs.SockJSHandler.class)
public class SockJSHandler implements io.vertx.core.Handler, java.util.function.Consumer {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new SockJSHandler((io.vertx.ext.web.handler.sockjs.SockJSHandler) obj),
SockJSHandler::getDelegate
);
private final io.vertx.ext.web.handler.sockjs.SockJSHandler delegate;
public SockJSHandler(io.vertx.ext.web.handler.sockjs.SockJSHandler delegate) {
this.delegate = delegate;
}
public SockJSHandler(Object delegate) {
this.delegate = (io.vertx.ext.web.handler.sockjs.SockJSHandler)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
SockJSHandler() {
this.delegate = null;
}
public io.vertx.ext.web.handler.sockjs.SockJSHandler getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SockJSHandler that = (SockJSHandler) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* @param vertx the Vert.x instance
* @return the handler
*/
public static io.vertx.mutiny.ext.web.handler.sockjs.SockJSHandler create(io.vertx.mutiny.core.Vertx vertx) {
io.vertx.mutiny.ext.web.handler.sockjs.SockJSHandler ret = io.vertx.mutiny.ext.web.handler.sockjs.SockJSHandler.newInstance((io.vertx.ext.web.handler.sockjs.SockJSHandler)io.vertx.ext.web.handler.sockjs.SockJSHandler.create(vertx.getDelegate()));
return ret;
}
/**
* @param vertx the Vert.x instance
* @param options options to configure the handler
* @return the handler
*/
public static io.vertx.mutiny.ext.web.handler.sockjs.SockJSHandler create(io.vertx.mutiny.core.Vertx vertx, io.vertx.ext.web.handler.sockjs.SockJSHandlerOptions options) {
io.vertx.mutiny.ext.web.handler.sockjs.SockJSHandler ret = io.vertx.mutiny.ext.web.handler.sockjs.SockJSHandler.newInstance((io.vertx.ext.web.handler.sockjs.SockJSHandler)io.vertx.ext.web.handler.sockjs.SockJSHandler.create(vertx.getDelegate(), options));
return ret;
}
/**
* @param handler the handler
* @return a router to be mounted on an existing router
*/
private io.vertx.mutiny.ext.web.Router __socketHandler(Handler handler) {
io.vertx.mutiny.ext.web.Router ret = io.vertx.mutiny.ext.web.Router.newInstance((io.vertx.ext.web.Router)delegate.socketHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(handler, event -> io.vertx.mutiny.ext.web.handler.sockjs.SockJSSocket.newInstance((io.vertx.ext.web.handler.sockjs.SockJSSocket)event))));
return ret;
}
/**
* @param handler the handler
* @return
*/
public io.vertx.mutiny.ext.web.Router socketHandler(java.util.function.Consumer handler) {
return __socketHandler(handler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(handler) : null);
}
/**
* @param bridgeOptions options to configure the bridge with
* @return a router to be mounted on an existing router
*/
public io.vertx.mutiny.ext.web.Router bridge(io.vertx.ext.web.handler.sockjs.SockJSBridgeOptions bridgeOptions) {
io.vertx.mutiny.ext.web.Router ret = io.vertx.mutiny.ext.web.Router.newInstance((io.vertx.ext.web.Router)delegate.bridge(bridgeOptions));
return ret;
}
/**
* @param authorizationProvider authorization provider to be used on the bridge
* @param bridgeOptions options to configure the bridge with
* @param bridgeEventHandler handler to receive bridge events
* @return a router to be mounted on an existing router
*/
private io.vertx.mutiny.ext.web.Router __bridge(io.vertx.mutiny.ext.auth.authorization.AuthorizationProvider authorizationProvider, io.vertx.ext.web.handler.sockjs.SockJSBridgeOptions bridgeOptions, Handler bridgeEventHandler) {
io.vertx.mutiny.ext.web.Router ret = io.vertx.mutiny.ext.web.Router.newInstance((io.vertx.ext.web.Router)delegate.bridge(authorizationProvider.getDelegate(), bridgeOptions, new io.smallrye.mutiny.vertx.DelegatingHandler<>(bridgeEventHandler, event -> io.vertx.mutiny.ext.web.handler.sockjs.BridgeEvent.newInstance((io.vertx.ext.web.handler.sockjs.BridgeEvent)event))));
return ret;
}
/**
* @param authorizationProvider authorization provider to be used on the bridge
* @param bridgeOptions options to configure the bridge with
* @param bridgeEventHandler handler to receive bridge events
* @return
*/
public io.vertx.mutiny.ext.web.Router bridge(io.vertx.mutiny.ext.auth.authorization.AuthorizationProvider authorizationProvider, io.vertx.ext.web.handler.sockjs.SockJSBridgeOptions bridgeOptions, java.util.function.Consumer bridgeEventHandler) {
return __bridge(authorizationProvider, bridgeOptions, bridgeEventHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(bridgeEventHandler) : null);
}
/**
* @param bridgeOptions options to configure the bridge with
* @param bridgeEventHandler handler to receive bridge events
* @return a router to be mounted on an existing router
*/
private io.vertx.mutiny.ext.web.Router __bridge(io.vertx.ext.web.handler.sockjs.SockJSBridgeOptions bridgeOptions, Handler bridgeEventHandler) {
io.vertx.mutiny.ext.web.Router ret = io.vertx.mutiny.ext.web.Router.newInstance((io.vertx.ext.web.Router)delegate.bridge(bridgeOptions, new io.smallrye.mutiny.vertx.DelegatingHandler<>(bridgeEventHandler, event -> io.vertx.mutiny.ext.web.handler.sockjs.BridgeEvent.newInstance((io.vertx.ext.web.handler.sockjs.BridgeEvent)event))));
return ret;
}
/**
* @param bridgeOptions options to configure the bridge with
* @param bridgeEventHandler handler to receive bridge events
* @return
*/
public io.vertx.mutiny.ext.web.Router bridge(io.vertx.ext.web.handler.sockjs.SockJSBridgeOptions bridgeOptions, java.util.function.Consumer bridgeEventHandler) {
return __bridge(bridgeOptions, bridgeEventHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(bridgeEventHandler) : null);
}
/**
* @param routingContext the routing context
* @deprecated mount the router as a sub-router instead. This method will not properly handle errors. */
@Deprecated
public void handle(io.vertx.mutiny.ext.web.RoutingContext routingContext) {
delegate.handle(routingContext.getDelegate());
}
public void accept(io.vertx.mutiny.ext.web.RoutingContext item) {
handle(item);
}
public static SockJSHandler newInstance(io.vertx.ext.web.handler.sockjs.SockJSHandler arg) {
return arg != null ? new SockJSHandler(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy