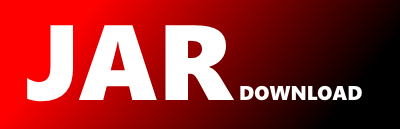
io.vertx.mutiny.ext.web.Route Maven / Gradle / Ivy
package io.vertx.mutiny.ext.web;
import java.util.Map;
import java.util.stream.Collectors;
import io.smallrye.mutiny.Multi;
import io.smallrye.mutiny.Uni;
import io.smallrye.mutiny.vertx.TypeArg;
import io.vertx.codegen.annotations.Fluent;
import io.smallrye.common.annotation.CheckReturnValue;
import java.util.List;
import io.vertx.core.http.HttpMethod;
import java.util.Map;
import java.util.Set;
import io.vertx.core.Handler;
import java.util.function.Function;
import io.vertx.core.Future;
/**
* A route is a holder for a set of criteria which determine whether an HTTP request or failure should be routed
* to a handler.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.Route original} non Mutiny-ified interface using Vert.x codegen.
*/
@io.smallrye.mutiny.vertx.MutinyGen(io.vertx.ext.web.Route.class)
public class Route {
public static final io.smallrye.mutiny.vertx.TypeArg __TYPE_ARG = new io.smallrye.mutiny.vertx.TypeArg<>( obj -> new Route((io.vertx.ext.web.Route) obj),
Route::getDelegate
);
private final io.vertx.ext.web.Route delegate;
public Route(io.vertx.ext.web.Route delegate) {
this.delegate = delegate;
}
public Route(Object delegate) {
this.delegate = (io.vertx.ext.web.Route)delegate;
}
/**
* Empty constructor used by CDI, do not use this constructor directly.
**/
Route() {
this.delegate = null;
}
public io.vertx.ext.web.Route getDelegate() {
return delegate;
}
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Route that = (Route) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
/**
* @param method the HTTP method to add
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route method(io.vertx.core.http.HttpMethod method) {
delegate.method(method);
return this;
}
/**
* @param path the path prefix
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route path(String path) {
delegate.path(path);
return this;
}
/**
* @param path the path regex
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route pathRegex(String path) {
delegate.pathRegex(path);
return this;
}
/**
* @param contentType the content type
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route produces(String contentType) {
delegate.produces(contentType);
return this;
}
/**
* @param contentType the content type
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route consumes(String contentType) {
delegate.consumes(contentType);
return this;
}
/**
* @param hostnamePattern the hostname pattern that should match Host
header of the requests
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route virtualHost(String hostnamePattern) {
delegate.virtualHost(hostnamePattern);
return this;
}
/**
* @param order the order
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route order(int order) {
delegate.order(order);
return this;
}
/**
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route last() {
delegate.last();
return this;
}
/**
* @param requestHandler the request handler
* @return a reference to this, so the API can be used fluently
*/
@Fluent
private io.vertx.mutiny.ext.web.Route __handler(Handler requestHandler) {
delegate.handler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(requestHandler, event -> io.vertx.mutiny.ext.web.RoutingContext.newInstance((io.vertx.ext.web.RoutingContext)event)));
return this;
}
/**
* @param requestHandler the request handler
* @return
*/
public io.vertx.mutiny.ext.web.Route handler(java.util.function.Consumer requestHandler) {
return __handler(requestHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(requestHandler) : null);
}
/**
* @param requestHandler
* @return the instance of Route to chain method calls.
*/
@Fluent
private io.vertx.mutiny.ext.web.Route __blockingHandler(Handler requestHandler) {
delegate.blockingHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(requestHandler, event -> io.vertx.mutiny.ext.web.RoutingContext.newInstance((io.vertx.ext.web.RoutingContext)event)));
return this;
}
/**
* @param requestHandler
* @return
*/
public io.vertx.mutiny.ext.web.Route blockingHandler(java.util.function.Consumer requestHandler) {
return __blockingHandler(requestHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(requestHandler) : null);
}
/**
* @param subRouter the router to add
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route subRouter(io.vertx.mutiny.ext.web.Router subRouter) {
delegate.subRouter(subRouter.getDelegate());
return this;
}
/**
* @param requestHandler the blocking request handler
* @param ordered if true handlers are executed in sequence, otherwise are run in parallel
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route blockingHandler(Handler requestHandler, boolean ordered) {
delegate.blockingHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(requestHandler, event -> io.vertx.mutiny.ext.web.RoutingContext.newInstance((io.vertx.ext.web.RoutingContext)event)), ordered);
return this;
}
/**
* @param failureHandler the request handler
* @return a reference to this, so the API can be used fluently
*/
@Fluent
private io.vertx.mutiny.ext.web.Route __failureHandler(Handler failureHandler) {
delegate.failureHandler(new io.smallrye.mutiny.vertx.DelegatingHandler<>(failureHandler, event -> io.vertx.mutiny.ext.web.RoutingContext.newInstance((io.vertx.ext.web.RoutingContext)event)));
return this;
}
/**
* @param failureHandler the request handler
* @return
*/
public io.vertx.mutiny.ext.web.Route failureHandler(java.util.function.Consumer failureHandler) {
return __failureHandler(failureHandler != null ? new io.smallrye.mutiny.vertx.DelegatingConsumerHandler(failureHandler) : null);
}
/**
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route remove() {
delegate.remove();
return this;
}
/**
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route disable() {
delegate.disable();
return this;
}
/**
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route enable() {
delegate.enable();
return this;
}
/**
* @param useNormalizedPath
* @return the instance of Route to chain method calls.
* @deprecated */
@Deprecated
@Fluent
public io.vertx.mutiny.ext.web.Route useNormalisedPath(boolean useNormalizedPath) {
delegate.useNormalisedPath(useNormalizedPath);
return this;
}
/**
* @param useNormalizedPath use normalized path for routing?
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route useNormalizedPath(boolean useNormalizedPath) {
delegate.useNormalizedPath(useNormalizedPath);
return this;
}
/**
* @param key the key for the metadata
* @return the data
*/
public T getMetadata(String key) {
T ret = (T) delegate.getMetadata(key);
return ret;
}
/**
* @return the path prefix (if any) for this route
*/
public String getPath() {
String ret = delegate.getPath();
return ret;
}
/**
* @return true if backed by a pattern.
*/
public boolean isRegexPath() {
boolean ret = delegate.isRegexPath();
return ret;
}
/**
* @return true if the path is exact.
*/
public boolean isExactPath() {
boolean ret = delegate.isExactPath();
return ret;
}
/**
* @return the http methods accepted by this route
*/
public Set methods() {
Set ret = delegate.methods();
return ret;
}
/**
* @param groups group names
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route setRegexGroupsNames(List groups) {
delegate.setRegexGroupsNames(groups);
return this;
}
/**
* @param name The name of the route.
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route setName(String name) {
delegate.setName(name);
return this;
}
/**
* @return the name of the route. If not given explicitly, the path or the pattern or null is returned (in that order)
*/
public String getName() {
String ret = delegate.getName();
return ret;
}
/**
* @param function the request handler function
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route respond(Function> function) {
delegate.respond(new java.util.function.Function>() {
public io.vertx.core.Future apply(io.vertx.ext.web.RoutingContext arg) {
return io.smallrye.mutiny.vertx.UniHelper.toFuture(
function.apply(io.vertx.mutiny.ext.web.RoutingContext.newInstance((io.vertx.ext.web.RoutingContext)arg))
);
}
});
return this;
}
/**
* @param key the metadata of key
* @param value the metadata of value
* @return a reference to this, so the API can be used fluently
*/
@Fluent
public io.vertx.mutiny.ext.web.Route putMetadata(String key, java.lang.Object value) {
delegate.putMetadata(key, value);
return this;
}
/**
* @return the metadata of this route, never returns null.
*/
public java.util.Map metadata() {
java.util.Map ret = delegate.metadata();
return ret;
}
public static Route newInstance(io.vertx.ext.web.Route arg) {
return arg != null ? new Route(arg) : null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy