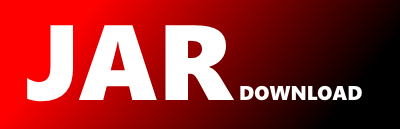
io.smallrye.reactive.messaging.amqp.AmqpConnectorIncomingConfiguration Maven / Gradle / Ivy
package io.smallrye.reactive.messaging.amqp;
import java.util.Optional;
import org.eclipse.microprofile.config.Config;
/**
* Extract the incoming configuration for the {@code smallrye-amqp} connector.
*/
public class AmqpConnectorIncomingConfiguration extends AmqpConnectorCommonConfiguration {
/**
* Creates a new AmqpConnectorIncomingConfiguration.
*/
public AmqpConnectorIncomingConfiguration(Config config) {
super(config);
validate();
}
/**
* Gets the broadcast value from the configuration.
* Attribute Name: broadcast
* Description: Whether the received AMQP messages must be dispatched to multiple _subscribers_
* Default Value: false
* @return the broadcast
*/
public Boolean getBroadcast() {
return config.getOptionalValue("broadcast", Boolean.class)
.orElse(Boolean.valueOf("false"));
}
/**
* Gets the durable value from the configuration.
* Attribute Name: durable
* Description: Whether AMQP subscription is durable
* Default Value: false
* @return the durable
*/
public Boolean getDurable() {
return config.getOptionalValue("durable", Boolean.class)
.orElse(Boolean.valueOf("false"));
}
/**
* Gets the auto-acknowledgement value from the configuration.
* Attribute Name: auto-acknowledgement
* Description: Whether the received AMQP messages must be acknowledged when received
* Default Value: false
* @return the auto-acknowledgement
*/
public Boolean getAutoAcknowledgement() {
return config.getOptionalValue("auto-acknowledgement", Boolean.class)
.orElse(Boolean.valueOf("false"));
}
/**
* Gets the failure-strategy value from the configuration.
* Attribute Name: failure-strategy
* Description: Specify the failure strategy to apply when a message produced from an AMQP message is nacked. Accepted values are `fail` (default), `accept`, `release`, `reject`, `modified-failed`, `modified-failed-undeliverable-here`
* Default Value: fail
* @return the failure-strategy
*/
public String getFailureStrategy() {
return config.getOptionalValue("failure-strategy", String.class)
.orElse("fail");
}
/**
* Gets the selector value from the configuration.
* Attribute Name: selector
* Description: Sets a message selector. This attribute is used to define an `apache.org:selector-filter:string` filter on the source terminus, using SQL-based syntax to request the server filters which messages are delivered to the receiver (if supported by the server in question). Precise functionality supported and syntax needed can vary depending on the server.
* @return the selector
*/
public Optional getSelector() {
return config.getOptionalValue("selector", String.class);
}
public void validate() {
super.validate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy