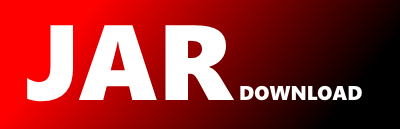
io.smallrye.reactive.messaging.amqp.AmqpConnectorOutgoingConfiguration Maven / Gradle / Ivy
package io.smallrye.reactive.messaging.amqp;
import java.util.Optional;
import org.eclipse.microprofile.config.Config;
/**
* Extract the outgoing configuration for the {@code smallrye-amqp} connector.
*/
public class AmqpConnectorOutgoingConfiguration extends AmqpConnectorCommonConfiguration {
/**
* Creates a new AmqpConnectorOutgoingConfiguration.
*/
public AmqpConnectorOutgoingConfiguration(Config config) {
super(config);
validate();
}
/**
* Gets the durable value from the configuration.
* Attribute Name: durable
* Description: Whether sent AMQP messages are marked durable
* Default Value: false
* @return the durable
*/
public Boolean getDurable() {
return config.getOptionalValue("durable", Boolean.class)
.orElse(Boolean.valueOf("false"));
}
/**
* Gets the ttl value from the configuration.
* Attribute Name: ttl
* Description: The time-to-live of the send AMQP messages. 0 to disable the TTL
* Default Value: 0
* @return the ttl
*/
public Long getTtl() {
return config.getOptionalValue("ttl", Long.class)
.orElse(Long.valueOf("0"));
}
/**
* Gets the credit-retrieval-period value from the configuration.
* Attribute Name: credit-retrieval-period
* Description: The period (in milliseconds) between two attempts to retrieve the credits granted by the broker. This time is used when the sender run out of credits.
* Default Value: 2000
* @return the credit-retrieval-period
*/
public Integer getCreditRetrievalPeriod() {
return config.getOptionalValue("credit-retrieval-period", Integer.class)
.orElse(Integer.valueOf("2000"));
}
/**
* Gets the max-inflight-messages value from the configuration.
* Attribute Name: max-inflight-messages
* Description: The maximum number of messages to be written to the broker concurrently. The number of sent messages waiting to be acknowledged by the broker are limited by this value and credits granted by the broker. The default value `0` means only credits apply.
* Default Value: 0
* @return the max-inflight-messages
*/
public Long getMaxInflightMessages() {
return config.getOptionalValue("max-inflight-messages", Long.class)
.orElse(Long.valueOf("0"));
}
/**
* Gets the use-anonymous-sender value from the configuration.
* Attribute Name: use-anonymous-sender
* Description: Whether or not the connector should use an anonymous sender. Default value is `true` if the broker supports it, `false` otherwise. If not supported, it is not possible to dynamically change the destination address.
* @return the use-anonymous-sender
*/
public Optional getUseAnonymousSender() {
return config.getOptionalValue("use-anonymous-sender", Boolean.class);
}
/**
* Gets the merge value from the configuration.
* Attribute Name: merge
* Description: Whether the connector should allow multiple upstreams
* Default Value: false
* @return the merge
*/
public Boolean getMerge() {
return config.getOptionalValue("merge", Boolean.class)
.orElse(Boolean.valueOf("false"));
}
/**
* Gets the cloud-events-source value from the configuration.
* Attribute Name: cloud-events-source
* Description: Configure the default `source` attribute of the outgoing Cloud Event. Requires `cloud-events` to be set to `true`. This value is used if the message does not configure the `source` attribute itself
* MicroProfile Config Alias: cloud-events-default-source
* @return the cloud-events-source
*/
public Optional getCloudEventsSource() {
Optional maybe = config.getOptionalValue("cloud-events-source", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("cloud-events-default-source", String.class);
}
/**
* Gets the cloud-events-type value from the configuration.
* Attribute Name: cloud-events-type
* Description: Configure the default `type` attribute of the outgoing Cloud Event. Requires `cloud-events` to be set to `true`. This value is used if the message does not configure the `type` attribute itself
* MicroProfile Config Alias: cloud-events-default-type
* @return the cloud-events-type
*/
public Optional getCloudEventsType() {
Optional maybe = config.getOptionalValue("cloud-events-type", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("cloud-events-default-type", String.class);
}
/**
* Gets the cloud-events-subject value from the configuration.
* Attribute Name: cloud-events-subject
* Description: Configure the default `subject` attribute of the outgoing Cloud Event. Requires `cloud-events` to be set to `true`. This value is used if the message does not configure the `subject` attribute itself
* MicroProfile Config Alias: cloud-events-default-subject
* @return the cloud-events-subject
*/
public Optional getCloudEventsSubject() {
Optional maybe = config.getOptionalValue("cloud-events-subject", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("cloud-events-default-subject", String.class);
}
/**
* Gets the cloud-events-data-content-type value from the configuration.
* Attribute Name: cloud-events-data-content-type
* Description: Configure the default `datacontenttype` attribute of the outgoing Cloud Event. Requires `cloud-events` to be set to `true`. This value is used if the message does not configure the `datacontenttype` attribute itself
* MicroProfile Config Alias: cloud-events-default-data-content-type
* @return the cloud-events-data-content-type
*/
public Optional getCloudEventsDataContentType() {
Optional maybe = config.getOptionalValue("cloud-events-data-content-type", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("cloud-events-default-data-content-type", String.class);
}
/**
* Gets the cloud-events-data-schema value from the configuration.
* Attribute Name: cloud-events-data-schema
* Description: Configure the default `dataschema` attribute of the outgoing Cloud Event. Requires `cloud-events` to be set to `true`. This value is used if the message does not configure the `dataschema` attribute itself
* MicroProfile Config Alias: cloud-events-default-data-schema
* @return the cloud-events-data-schema
*/
public Optional getCloudEventsDataSchema() {
Optional maybe = config.getOptionalValue("cloud-events-data-schema", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("cloud-events-default-data-schema", String.class);
}
/**
* Gets the cloud-events-insert-timestamp value from the configuration.
* Attribute Name: cloud-events-insert-timestamp
* Description: Whether or not the connector should insert automatically the `time` attribute into the outgoing Cloud Event. Requires `cloud-events` to be set to `true`. This value is used if the message does not configure the `time` attribute itself
* MicroProfile Config Alias: cloud-events-default-timestamp
* Default Value: true
* @return the cloud-events-insert-timestamp
*/
public Boolean getCloudEventsInsertTimestamp() {
return config.getOptionalValue("cloud-events-insert-timestamp", Boolean.class)
.orElseGet(() -> getFromAliasWithDefaultValue("cloud-events-default-timestamp", Boolean.class, Boolean.valueOf("true")));
}
/**
* Gets the cloud-events-mode value from the configuration.
* Attribute Name: cloud-events-mode
* Description: The Cloud Event mode (`structured` or `binary` (default)). Indicates how are written the cloud events in the outgoing record
* Default Value: binary
* @return the cloud-events-mode
*/
public String getCloudEventsMode() {
return config.getOptionalValue("cloud-events-mode", String.class)
.orElse("binary");
}
public void validate() {
super.validate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy