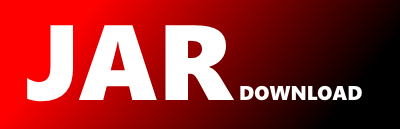
io.smallrye.reactive.messaging.amqp.AmqpConnectorCommonConfiguration Maven / Gradle / Ivy
The newest version!
package io.smallrye.reactive.messaging.amqp;
import java.util.Optional;
import org.eclipse.microprofile.config.Config;
import org.eclipse.microprofile.config.ConfigProvider;
import org.eclipse.microprofile.reactive.messaging.spi.ConnectorFactory;
/**
* Extracts the common configuration for the {@code smallrye-amqp} connector.
*/
public class AmqpConnectorCommonConfiguration {
protected final Config config;
/**
* Creates a new AmqpConnectorCommonConfiguration.
*/
public AmqpConnectorCommonConfiguration(Config config) {
this.config = config;
}
/**
* @return the connector configuration
*/
public Config config() {
return this.config;
}
/**
* Retrieves the value stored for the given alias.
* @param alias the attribute alias, must not be {@code null} or blank
* @param type the targeted type
* @param the targeted type
* @return the configuration value for the given alias, empty if not set
*/
protected Optional getFromAlias(String alias, Class type) {
return ConfigProvider.getConfig().getOptionalValue(alias, type);
}
/**
* Retrieves the value stored for the given alias. Returns the default value if not present.
* @param alias the attribute alias, must not be {@code null} or blank
* @param type the targeted type
* @param defaultValue the default value
* @param the targeted type
* @return the configuration value for the given alias, empty if not set
*/
protected T getFromAliasWithDefaultValue(String alias, Class type, T defaultValue) {
return getFromAlias(alias, type).orElse(defaultValue);
}
/**
* @return the channel name
*/
public String getChannel() {
return config.getValue(ConnectorFactory.CHANNEL_NAME_ATTRIBUTE, String.class);
}
/**
* Gets the username value from the configuration.
* Attribute Name: username
* Description: The username used to authenticate to the broker
* MicroProfile Config Alias: amqp-username
* @return the username
*/
public Optional getUsername() {
Optional maybe = config.getOptionalValue("username", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("amqp-username", String.class);
}
/**
* Gets the password value from the configuration.
* Attribute Name: password
* Description: The password used to authenticate to the broker
* MicroProfile Config Alias: amqp-password
* @return the password
*/
public Optional getPassword() {
Optional maybe = config.getOptionalValue("password", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("amqp-password", String.class);
}
/**
* Gets the host value from the configuration.
* Attribute Name: host
* Description: The broker hostname
* MicroProfile Config Alias: amqp-host
* Default Value: localhost
* @return the host
*/
public String getHost() {
return config.getOptionalValue("host", String.class)
.orElseGet(() -> getFromAliasWithDefaultValue("amqp-host", String.class, "localhost"));
}
/**
* Gets the port value from the configuration.
* Attribute Name: port
* Description: The broker port
* MicroProfile Config Alias: amqp-port
* Default Value: 5672
* @return the port
*/
public Integer getPort() {
return config.getOptionalValue("port", Integer.class)
.orElseGet(() -> getFromAliasWithDefaultValue("amqp-port", Integer.class, Integer.valueOf("5672")));
}
/**
* Gets the use-ssl value from the configuration.
* Attribute Name: use-ssl
* Description: Whether the AMQP connection uses SSL/TLS
* MicroProfile Config Alias: amqp-use-ssl
* Default Value: false
* @return the use-ssl
*/
public Boolean getUseSsl() {
return config.getOptionalValue("use-ssl", Boolean.class)
.orElseGet(() -> getFromAliasWithDefaultValue("amqp-use-ssl", Boolean.class, Boolean.valueOf("false")));
}
/**
* Gets the virtual-host value from the configuration.
* Attribute Name: virtual-host
* Description: If set, configure the hostname value used for the connection AMQP Open frame and TLS SNI server name (if TLS is in use)
* MicroProfile Config Alias: amqp-virtual-host
* @return the virtual-host
*/
public Optional getVirtualHost() {
Optional maybe = config.getOptionalValue("virtual-host", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("amqp-virtual-host", String.class);
}
/**
* Gets the sni-server-name value from the configuration.
* Attribute Name: sni-server-name
* Description: If set, explicitly override the hostname to use for the TLS SNI server name
* MicroProfile Config Alias: amqp-sni-server-name
* @return the sni-server-name
*/
public Optional getSniServerName() {
Optional maybe = config.getOptionalValue("sni-server-name", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("amqp-sni-server-name", String.class);
}
/**
* Gets the reconnect-attempts value from the configuration.
* Attribute Name: reconnect-attempts
* Description: The number of reconnection attempts
* MicroProfile Config Alias: amqp-reconnect-attempts
* Default Value: 100
* @return the reconnect-attempts
*/
public Integer getReconnectAttempts() {
return config.getOptionalValue("reconnect-attempts", Integer.class)
.orElseGet(() -> getFromAliasWithDefaultValue("amqp-reconnect-attempts", Integer.class, Integer.valueOf("100")));
}
/**
* Gets the reconnect-interval value from the configuration.
* Attribute Name: reconnect-interval
* Description: The interval in second between two reconnection attempts
* MicroProfile Config Alias: amqp-reconnect-interval
* Default Value: 10
* @return the reconnect-interval
*/
public Integer getReconnectInterval() {
return config.getOptionalValue("reconnect-interval", Integer.class)
.orElseGet(() -> getFromAliasWithDefaultValue("amqp-reconnect-interval", Integer.class, Integer.valueOf("10")));
}
/**
* Gets the connect-timeout value from the configuration.
* Attribute Name: connect-timeout
* Description: The connection timeout in milliseconds
* MicroProfile Config Alias: amqp-connect-timeout
* Default Value: 1000
* @return the connect-timeout
*/
public Integer getConnectTimeout() {
return config.getOptionalValue("connect-timeout", Integer.class)
.orElseGet(() -> getFromAliasWithDefaultValue("amqp-connect-timeout", Integer.class, Integer.valueOf("1000")));
}
/**
* Gets the container-id value from the configuration.
* Attribute Name: container-id
* Description: The AMQP container id
* @return the container-id
*/
public Optional getContainerId() {
return config.getOptionalValue("container-id", String.class);
}
/**
* Gets the address value from the configuration.
* Attribute Name: address
* Description: The AMQP address. If not set, the channel name is used
* @return the address
*/
public Optional getAddress() {
return config.getOptionalValue("address", String.class);
}
/**
* Gets the link-name value from the configuration.
* Attribute Name: link-name
* Description: The name of the link. If not set, the channel name is used.
* @return the link-name
*/
public Optional getLinkName() {
return config.getOptionalValue("link-name", String.class);
}
/**
* Gets the client-options-name value from the configuration.
* Attribute Name: client-options-name
* Description: The name of the AMQP Client Option bean used to customize the AMQP client configuration
* MicroProfile Config Alias: amqp-client-options-name
* @return the client-options-name
*/
public Optional getClientOptionsName() {
Optional maybe = config.getOptionalValue("client-options-name", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("amqp-client-options-name", String.class);
}
/**
* Gets the client-ssl-context-name value from the configuration.
* Attribute Name: client-ssl-context-name
* Description: The name of an SSLContext bean to use for connecting to AMQP when SSL is used
* MicroProfile Config Alias: amqp-client-ssl-context-name
* @return the client-ssl-context-name
*/
public Optional getClientSslContextName() {
Optional maybe = config.getOptionalValue("client-ssl-context-name", String.class);
if (maybe.isPresent()) { return maybe; }
return getFromAlias("amqp-client-ssl-context-name", String.class);
}
/**
* Gets the tracing-enabled value from the configuration.
* Attribute Name: tracing-enabled
* Description: Whether tracing is enabled (default) or disabled
* Default Value: true
* @return the tracing-enabled
*/
public Boolean getTracingEnabled() {
return config.getOptionalValue("tracing-enabled", Boolean.class)
.orElse(Boolean.valueOf("true"));
}
/**
* Gets the health-timeout value from the configuration.
* Attribute Name: health-timeout
* Description: The max number of seconds to wait to determine if the connection with the broker is still established for the readiness check. After that threshold, the check is considered as failed.
* Default Value: 3
* @return the health-timeout
*/
public Integer getHealthTimeout() {
return config.getOptionalValue("health-timeout", Integer.class)
.orElse(Integer.valueOf("3"));
}
/**
* Gets the cloud-events value from the configuration.
* Attribute Name: cloud-events
* Description: Enables (default) or disables the Cloud Event support. If enabled on an _incoming_ channel, the connector analyzes the incoming records and try to create Cloud Event metadata. If enabled on an _outgoing_, the connector sends the outgoing messages as Cloud Event if the message includes Cloud Event Metadata.
* Default Value: true
* @return the cloud-events
*/
public Boolean getCloudEvents() {
return config.getOptionalValue("cloud-events", Boolean.class)
.orElse(Boolean.valueOf("true"));
}
/**
* Gets the capabilities value from the configuration.
* Attribute Name: capabilities
* Description: A comma-separated list of capabilities proposed by the sender or receiver client.
* @return the capabilities
*/
public Optional getCapabilities() {
return config.getOptionalValue("capabilities", String.class);
}
public void validate() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy