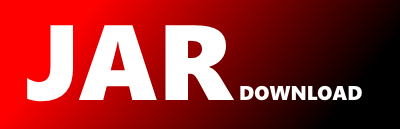
io.smallrye.reactive.messaging.ce.OutgoingCloudEventMetadataBuilder Maven / Gradle / Ivy
The newest version!
package io.smallrye.reactive.messaging.ce;
import java.net.URI;
import java.time.ZonedDateTime;
import java.util.Map;
import java.util.UUID;
import io.smallrye.reactive.messaging.ce.impl.DefaultOutgoingCloudEventMetadata;
public final class OutgoingCloudEventMetadataBuilder {
private final DefaultCloudEventMetadataBuilder builder = new DefaultCloudEventMetadataBuilder<>();
/**
* The Cloud Event Id.
* We store it to inject a random UUID if not set before the construction.
*/
private String id;
public OutgoingCloudEventMetadataBuilder() {
// Do nothing by default
}
public OutgoingCloudEventMetadataBuilder(OutgoingCloudEventMetadata existing) {
builder.withSpecVersion(existing.getSpecVersion());
this.id = existing.getId();
builder.withId(existing.getId());
builder.withSource(existing.getSource());
builder.withType(existing.getType());
builder.withData(existing.getData());
builder.withExtensions(existing.getExtensions());
builder.withTimestamp(existing.getTimeStamp().orElse(null));
builder.withSubject(existing.getSubject().orElse(null));
builder.withDataSchema(existing.getDataSchema().orElse(null));
builder.withDataContentType(existing.getDataContentType().orElse(null));
}
public OutgoingCloudEventMetadataBuilder withId(String id) {
this.id = id;
builder.withId(id);
return this;
}
public OutgoingCloudEventMetadataBuilder withSpecVersion(String specVersion) {
builder.withSpecVersion(specVersion);
return this;
}
public OutgoingCloudEventMetadataBuilder withSource(URI source) {
builder.withSource(source);
return this;
}
public OutgoingCloudEventMetadataBuilder withType(String type) {
builder.withType(type);
return this;
}
public OutgoingCloudEventMetadataBuilder withDataContentType(String dataContentType) {
builder.withDataContentType(dataContentType);
return this;
}
public OutgoingCloudEventMetadataBuilder withDataSchema(URI dataSchema) {
builder.withDataSchema(dataSchema);
return this;
}
public OutgoingCloudEventMetadataBuilder withSubject(String subject) {
builder.withSubject(subject);
return this;
}
public OutgoingCloudEventMetadataBuilder withTimestamp(ZonedDateTime timestamp) {
builder.withTimestamp(timestamp);
return this;
}
public OutgoingCloudEventMetadataBuilder withExtensions(Map extensions) {
builder.withExtensions(extensions);
return this;
}
public OutgoingCloudEventMetadataBuilder withExtension(String name, Object value) {
builder.withExtension(name, value);
return this;
}
public OutgoingCloudEventMetadataBuilder withoutExtension(String name) {
builder.withoutExtension(name);
return this;
}
public OutgoingCloudEventMetadata build() {
if (id == null) {
builder.withId(UUID.randomUUID().toString());
}
return new DefaultOutgoingCloudEventMetadata<>(builder.build());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy