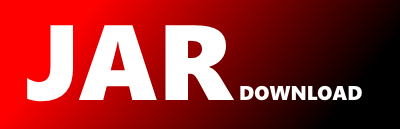
io.smallrye.reactive.messaging.aws.sqs.SqsConnectorIncomingConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smallrye-reactive-messaging-aws-sqs Show documentation
Show all versions of smallrye-reactive-messaging-aws-sqs Show documentation
A module for Smallrye reactive messaging integration with AWS SQS Service
The newest version!
package io.smallrye.reactive.messaging.aws.sqs;
import java.util.Optional;
import org.eclipse.microprofile.config.Config;
/**
* Extract the incoming configuration for the {@code smallrye-sqs} connector.
*/
public class SqsConnectorIncomingConfiguration extends SqsConnectorCommonConfiguration {
/**
* Creates a new SqsConnectorIncomingConfiguration.
*/
public SqsConnectorIncomingConfiguration(Config config) {
super(config);
validate();
}
/**
* Gets the wait-time-seconds value from the configuration.
* Attribute Name: wait-time-seconds
* Description: The maximum amount of time in seconds to wait for messages to be received
* Default Value: 1
* @return the wait-time-seconds
*/
public Integer getWaitTimeSeconds() {
return config.getOptionalValue("wait-time-seconds", Integer.class)
.orElse(Integer.valueOf("1"));
}
/**
* Gets the max-number-of-messages value from the configuration.
* Attribute Name: max-number-of-messages
* Description: The maximum number of messages to receive
* Default Value: 10
* @return the max-number-of-messages
*/
public Integer getMaxNumberOfMessages() {
return config.getOptionalValue("max-number-of-messages", Integer.class)
.orElse(Integer.valueOf("10"));
}
/**
* Gets the visibility-timeout value from the configuration.
* Attribute Name: visibility-timeout
* Description: The duration in seconds that the received messages are hidden from subsequent retrieve requests after being retrieved by a receive request
* @return the visibility-timeout
*/
public Optional getVisibilityTimeout() {
return config.getOptionalValue("visibility-timeout", Integer.class);
}
/**
* Gets the receive.request.message-attribute-names value from the configuration.
* Attribute Name: receive.request.message-attribute-names
* Description: The message attribute names to retrieve when receiving messages.
* @return the receive.request.message-attribute-names
*/
public Optional getReceiveRequestMessageAttributeNames() {
return config.getOptionalValue("receive.request.message-attribute-names", String.class);
}
/**
* Gets the receive.request.customizer value from the configuration.
* Attribute Name: receive.request.customizer
* Description: The identifier for the bean implementing a customizer to receive requests, defaults to channel name if not provided
* @return the receive.request.customizer
*/
public Optional getReceiveRequestCustomizer() {
return config.getOptionalValue("receive.request.customizer", String.class);
}
/**
* Gets the receive.request.retries value from the configuration.
* Attribute Name: receive.request.retries
* Description: If set to a positive number, the connector will try to retry the request that was not delivered successfully (with a potentially transient error) until the number of retries is reached. If set to 0, retries are disabled.
* Default Value: 2147483647
* @return the receive.request.retries
*/
public Long getReceiveRequestRetries() {
return config.getOptionalValue("receive.request.retries", Long.class)
.orElse(Long.valueOf("2147483647"));
}
/**
* Gets the receive.request.pause.resume value from the configuration.
* Attribute Name: receive.request.pause.resume
* Description: Whether the polling must be paused when the application does not request items and resume when it does. This allows implementing back-pressure based on the application capacity. Note that polling is not stopped, but will not retrieve any records when paused.
* Default Value: true
* @return the receive.request.pause.resume
*/
public Boolean getReceiveRequestPauseResume() {
return config.getOptionalValue("receive.request.pause.resume", Boolean.class)
.orElse(Boolean.valueOf("true"));
}
/**
* Gets the ack.delete value from the configuration.
* Attribute Name: ack.delete
* Description: Whether the acknowledgement deletes the message from the queue
* Default Value: true
* @return the ack.delete
*/
public Boolean getAckDelete() {
return config.getOptionalValue("ack.delete", Boolean.class)
.orElse(Boolean.valueOf("true"));
}
public void validate() {
super.validate();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy