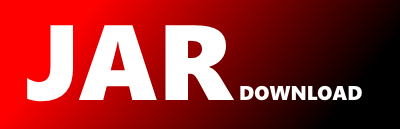
io.smallrye.jwt.build.impl.ImplMessages_$bundle Maven / Gradle / Ivy
The newest version!
package io.smallrye.jwt.build.impl;
import java.util.Locale;
import java.io.Serializable;
import java.lang.NullPointerException;
import java.lang.Throwable;
import io.smallrye.jwt.build.JwtException;
import java.lang.String;
import io.smallrye.jwt.build.JwtSignatureException;
import java.util.Arrays;
import io.smallrye.jwt.build.JwtEncryptionException;
import java.lang.IllegalArgumentException;
/**
* Warning this class consists of generated code.
*/
public class ImplMessages_$bundle implements ImplMessages, Serializable {
private static final long serialVersionUID = 1L;
protected ImplMessages_$bundle() {}
public static final ImplMessages_$bundle INSTANCE = new ImplMessages_$bundle();
protected Object readResolve() {
return INSTANCE;
}
private static final Locale LOCALE = Locale.ROOT;
protected Locale getLoggingLocale() {
return LOCALE;
}
protected String unsupportedSignatureAlgorithm$str() {
return "SRJWT05000: Unsupported signature algorithm: %s";
}
@Override
public final JwtSignatureException unsupportedSignatureAlgorithm(final String algorithmName, final Throwable throwable) {
final JwtSignatureException result = new JwtSignatureException(String.format(getLoggingLocale(), unsupportedSignatureAlgorithm$str(), algorithmName), throwable);
_copyStackTraceMinusOne(result);
return result;
}
private static void _copyStackTraceMinusOne(final Throwable e) {
final StackTraceElement[] st = e.getStackTrace();
e.setStackTrace(Arrays.copyOfRange(st, 1, st.length));
}
@Override
public final JwtSignatureException unsupportedSignatureAlgorithm(final String algorithmName) {
final JwtSignatureException result = new JwtSignatureException(String.format(getLoggingLocale(), unsupportedSignatureAlgorithm$str(), algorithmName));
_copyStackTraceMinusOne(result);
return result;
}
protected String joseSerializationError$str() {
return "SRJWT05003: %s";
}
@Override
public final JwtEncryptionException joseSerializationError(final String errorMessage, final Throwable t) {
final JwtEncryptionException result = new JwtEncryptionException(String.format(getLoggingLocale(), joseSerializationError$str(), errorMessage), t);
_copyStackTraceMinusOne(result);
return result;
}
protected String unsupportedKeyEncryptionAlgorithm$str() {
return "SRJWT05005: Unsupported key encryption algorithm: %s";
}
@Override
public final JwtEncryptionException unsupportedKeyEncryptionAlgorithm(final String algorithmName) {
final JwtEncryptionException result = new JwtEncryptionException(String.format(getLoggingLocale(), unsupportedKeyEncryptionAlgorithm$str(), algorithmName));
_copyStackTraceMinusOne(result);
return result;
}
protected String unsupportedContentEncryptionAlgorithm$str() {
return "SRJWT05006: Unsupported content encryption algorithm: %s";
}
@Override
public final JwtEncryptionException unsupportedContentEncryptionAlgorithm(final String algorithmName) {
final JwtEncryptionException result = new JwtEncryptionException(String.format(getLoggingLocale(), unsupportedContentEncryptionAlgorithm$str(), algorithmName));
_copyStackTraceMinusOne(result);
return result;
}
protected String encryptionKeyCanNotBeLoadedFromLocation$str() {
return "SRJWT05007: Key encryption key can not be loaded from: %s";
}
@Override
public final IllegalArgumentException encryptionKeyCanNotBeLoadedFromLocation(final String keyLocation) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), encryptionKeyCanNotBeLoadedFromLocation$str(), keyLocation));
_copyStackTraceMinusOne(result);
return result;
}
protected String encryptionKeyNotConfigured$str() {
return "SRJWT05008: Please set 'smallrye.jwt.encrypt.key.location' or 'smallrye.jwt.encrypt.key' property";
}
@Override
public final IllegalArgumentException encryptionKeyNotConfigured() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), encryptionKeyNotConfigured$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String signatureException$str() {
return "SRJWT05009: ";
}
@Override
public final JwtSignatureException signatureException(final Throwable throwable) {
final JwtSignatureException result = new JwtSignatureException(String.format(getLoggingLocale(), signatureException$str()), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String signKeyPropertyRequired$str() {
return "SRJWT05010: Inner JWT can not be created, 'smallrye.jwt.sign.key.location' is not set but the 'alg' header is: %s";
}
@Override
public final JwtSignatureException signKeyPropertyRequired(final String algorithmName) {
final JwtSignatureException result = new JwtSignatureException(String.format(getLoggingLocale(), signKeyPropertyRequired$str(), algorithmName));
_copyStackTraceMinusOne(result);
return result;
}
protected String noneSignatureAlgorithmUnsupported$str() {
return "SRJWT05011: None signature algorithm is currently not supported";
}
@Override
public final JwtSignatureException noneSignatureAlgorithmUnsupported() {
final JwtSignatureException result = new JwtSignatureException(String.format(getLoggingLocale(), noneSignatureAlgorithmUnsupported$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String signJwtTokenFailed$str() {
return "SRJWT05012: Failure to create a signed JWT token: %s";
}
@Override
public final JwtSignatureException signJwtTokenFailed(final String exceptionMessage, final Throwable throwable) {
final JwtSignatureException result = new JwtSignatureException(String.format(getLoggingLocale(), signJwtTokenFailed$str(), exceptionMessage), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String algDoesNotMatchKeyType$str() {
return "SRJWT05013: JWK algorithm 'alg' value does not match a key type";
}
@Override
public final IllegalArgumentException algDoesNotMatchKeyType() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), algDoesNotMatchKeyType$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String publicKeyBeingUsedForSign$str() {
return "SRJWT05014: Only PrivateKey or SecretKey can be be used to sign a token";
}
@Override
public final IllegalArgumentException publicKeyBeingUsedForSign() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), publicKeyBeingUsedForSign$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String readJsonFailure$str() {
return "SRJWT05015: Failure to read the json content: %s";
}
@Override
public final JwtException readJsonFailure(final String exceptionMessage, final Throwable throwable) {
final JwtException result = new JwtException(String.format(getLoggingLocale(), readJsonFailure$str(), exceptionMessage), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String failureToParseJWK$str() {
return "SRJWT05016: Failure to parse JWK: %s";
}
@Override
public final JwtException failureToParseJWK(final String exceptionMessage, final Throwable throwable) {
final JwtException result = new JwtException(String.format(getLoggingLocale(), failureToParseJWK$str(), exceptionMessage), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String failureToParseJWKS$str() {
return "SRJWT05017: Failure to parse JWK Set: %s";
}
@Override
public final JwtException failureToParseJWKS(final String exceptionMessage, final Throwable throwable) {
final JwtException result = new JwtException(String.format(getLoggingLocale(), failureToParseJWKS$str(), exceptionMessage), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String kidRequired$str() {
return "SRJWT05018: Key id 'kid' header value must be provided";
}
@Override
public final IllegalArgumentException kidRequired() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), kidRequired$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String keyWithKidNotFound$str() {
return "SRJWT05019: JWK set has no key with a key id 'kid' header '%s'";
}
@Override
public final IllegalArgumentException keyWithKidNotFound(final String keyId) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), keyWithKidNotFound$str(), keyId));
_copyStackTraceMinusOne(result);
return result;
}
protected String signingKeyCanNotBeLoadedFromLocation$str() {
return "SRJWT05020: Signing key can not be loaded from: %s";
}
@Override
public final IllegalArgumentException signingKeyCanNotBeLoadedFromLocation(final String keyLocation, final Throwable throwable) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), signingKeyCanNotBeLoadedFromLocation$str(), keyLocation), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String signKeyNotConfigured$str() {
return "SRJWT05021: Please set 'smallrye.jwt.sign.key.location' or 'smallrye.jwt.sign.key' property";
}
@Override
public final IllegalArgumentException signKeyNotConfigured() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), signKeyNotConfigured$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String failureToParseJWTClaims$str() {
return "SRJWT05022: Failure to parse the JWT claims: %s";
}
@Override
public final JwtException failureToParseJWTClaims(final String exceptionMessage, final Throwable throwable) {
final JwtException result = new JwtException(String.format(getLoggingLocale(), failureToParseJWTClaims$str(), exceptionMessage), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String failureToReadPrivateKey$str() {
return "SRJWT05024: Failure to read the private key: %s";
}
@Override
public final JwtException failureToReadPrivateKey(final String exceptionMessage, final Throwable throwable) {
final JwtException result = new JwtException(String.format(getLoggingLocale(), failureToReadPrivateKey$str(), exceptionMessage), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String failureToOpenInputStreamFromJsonResName$str() {
return "SRJWT05025: Failure to open the input stream from %s";
}
@Override
public final JwtException failureToOpenInputStreamFromJsonResName(final String jsonResName) {
final JwtException result = new JwtException(String.format(getLoggingLocale(), failureToOpenInputStreamFromJsonResName$str(), jsonResName));
_copyStackTraceMinusOne(result);
return result;
}
protected String failureToReadJsonContentFromJsonResName$str() {
return "SRJWT05026: Failure to read the json content from %s: %s";
}
@Override
public final JwtException failureToReadJsonContentFromJsonResName(final String jsonResName, final String exceptionMessage, final Throwable throwable) {
final JwtException result = new JwtException(String.format(getLoggingLocale(), failureToReadJsonContentFromJsonResName$str(), jsonResName, exceptionMessage), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String encryptionException$str() {
return "SRJWT05027: Failure to encrypt the token";
}
@Override
public final JwtEncryptionException encryptionException(final Throwable throwable) {
final JwtEncryptionException result = new JwtEncryptionException(String.format(getLoggingLocale(), encryptionException$str()), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String signingKeyCanNotBeCreatedFromContent$str() {
return "SRJWT05028: Signing key can not be created from the loaded content";
}
@Override
public final IllegalArgumentException signingKeyCanNotBeCreatedFromContent() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), signingKeyCanNotBeCreatedFromContent$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String encryptionKeyCanNotBeCreatedFromContent$str() {
return "SRJWT05029: Encryption key can not be created from the loaded content";
}
@Override
public final IllegalArgumentException encryptionKeyCanNotBeCreatedFromContent() {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), encryptionKeyCanNotBeCreatedFromContent$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String signingKeyCanNotBeReadFromKeystore$str() {
return "SRJWT05030: Signing key can not be read from the keystore";
}
@Override
public final IllegalArgumentException signingKeyCanNotBeReadFromKeystore(final Throwable throwable) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), signingKeyCanNotBeReadFromKeystore$str()), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String encryptionKeyCanNotBeReadFromKeystore$str() {
return "SRJWT05031: Encryption key can not be read from the keystore";
}
@Override
public final IllegalArgumentException encryptionKeyCanNotBeReadFromKeystore(final Throwable throwable) {
final IllegalArgumentException result = new IllegalArgumentException(String.format(getLoggingLocale(), encryptionKeyCanNotBeReadFromKeystore$str()), throwable);
_copyStackTraceMinusOne(result);
return result;
}
protected String signingKeyIsNull$str() {
return "SRJWT05032: Signing key is null";
}
@Override
public final NullPointerException signingKeyIsNull() {
final NullPointerException result = new NullPointerException(String.format(getLoggingLocale(), signingKeyIsNull$str()));
_copyStackTraceMinusOne(result);
return result;
}
protected String encryptionKeyIsNull$str() {
return "SRJWT05033: Encryption key is null";
}
@Override
public final NullPointerException encryptionKeyIsNull() {
final NullPointerException result = new NullPointerException(String.format(getLoggingLocale(), encryptionKeyIsNull$str()));
_copyStackTraceMinusOne(result);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy