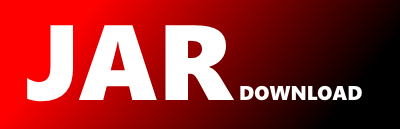
io.smilego.tenant.flyway.TenantFlywayMigrationInitializer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of multi-tenant-spring-boot-starter Show documentation
Show all versions of multi-tenant-spring-boot-starter Show documentation
A application used as an example on how to set up pushing
its components to the Central Repository.
package io.smilego.tenant.flyway;
import io.smilego.tenant.model.Tenant;
import io.smilego.tenant.persistence.TenantRepository;
import io.smilego.tenant.util.AESUtils;
import io.smilego.tenant.util.LogBuilder;
import org.flywaydb.core.Flyway;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.jdbc.datasource.SingleConnectionDataSource;
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.Collection;
public class TenantFlywayMigrationInitializer implements InitializingBean {
Logger log = LoggerFactory.getLogger(this.getClass());
@Value("${multitenancy.security.encryption-key}")
private String encryptionKey;
@Value("${multitenancy.application.name}")
private String applicationName;
@Autowired
private TenantRepository tenantRepository;
public void migrateAllTenants(Collection tenants) {
for(Tenant t : tenants){
try (Connection connection = DriverManager.getConnection(t.getUrl().concat("/").concat(Tenant.TENANT_DATABASE_PREFIX).concat(applicationName),
t.getDb(),
AESUtils.decrypt(encryptionKey, t.getPassword()))){
String scriptLocation = "classpath:db/migration/tenant";
DataSource tenantDataSource = new SingleConnectionDataSource(connection, false);
Flyway flyway = Flyway.configure()
.locations(scriptLocation)
.baselineOnMigrate(Boolean.TRUE)
.dataSource(tenantDataSource)
.schemas("public")
.load();
flyway.migrate();
}catch (SQLException e ){
log.error("Failed to run Flyway migrations for tenant " + t.getTenantId(), e);
}
}
}
@Override
public void afterPropertiesSet() throws Exception {
log.info(LogBuilder.of()
.header("Starting flyway migrations")
.row("Database: tenantDataSource").build());
this.migrateAllTenants(tenantRepository.findAll());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy