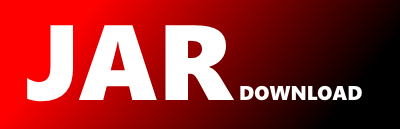
io.smilego.tenant.model.Param Maven / Gradle / Ivy
package io.smilego.tenant.model;
import javax.persistence.*;
import javax.validation.constraints.NotNull;
@Entity
@Table(name = "t_param", schema = "tenant")
public class Param extends BaseEntity {
private static final long serialVersionUID = 1L;
@NotNull
@ManyToOne
@JoinColumn(name = "tenant_id", nullable = false)
private Tenant tenant;
@NotNull
@ManyToOne
@JoinColumn(name = "service_id", nullable = false)
private Service service;
@NotNull
@ManyToOne
@JoinColumn(name = "property_id", nullable = false)
private Property property;
@NotNull
@Column(name = "value", length = 248, nullable = false)
private String value;
public Param() {
}
public Param(Tenant tenant, Service service, Property property, String value) {
this.tenant = tenant;
this.service = service;
this.property = property;
this.value = value;
}
public Tenant getTenant() {
return tenant;
}
public void setTenant(Tenant tenant) {
this.tenant = tenant;
}
public Service getService() {
return service;
}
public void setService(Service service) {
this.service = service;
}
public Property getProperty() {
return property;
}
public void setProperty(Property property) {
this.property = property;
}
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
@Override
public String toString() {
return "Param{" +
"tenant=" + tenant +
", service=" + service +
", property=" + property +
", value='" + value + '\'' +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy