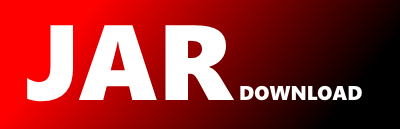
io.smooch.client.JSON Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
io.smooch - api - 5.29.0
/*
* Smooch
* The Smooch API is a unified interface for powering messaging in your customer experiences across every channel. Our API speeds access to new markets, reduces time to ship, eliminates complexity, and helps you build the best experiences for your customers. For more information, visit our [official documentation](https://docs.smooch.io).
*
* OpenAPI spec version: 5.8
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.smooch.client;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonNull;
import com.google.gson.JsonParseException;
import com.google.gson.JsonPrimitive;
import com.google.gson.JsonSerializationContext;
import com.google.gson.JsonSerializer;
import com.google.gson.TypeAdapter;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import java.io.IOException;
import java.io.StringReader;
import java.lang.reflect.Type;
import java.util.Date;
import org.joda.time.DateTime;
import org.joda.time.LocalDate;
import org.joda.time.format.DateTimeFormatter;
import org.joda.time.format.ISODateTimeFormat;
public class JSON {
private ApiClient apiClient;
private Gson gson;
/**
* JSON constructor.
*
* @param apiClient An instance of ApiClient
*/
public JSON(ApiClient apiClient) {
this.apiClient = apiClient;
gson = new GsonBuilder()
.registerTypeAdapter(Date.class, new DateAdapter(apiClient))
.registerTypeAdapter(DateTime.class, new DateTimeTypeAdapter())
.registerTypeAdapter(LocalDate.class, new LocalDateTypeAdapter())
.create();
}
/**
* Get Gson.
*
* @return Gson
*/
public Gson getGson() {
return gson;
}
/**
* Set Gson.
*
* @param gson Gson
*/
public void setGson(Gson gson) {
this.gson = gson;
}
/**
* Serialize the given Java object into JSON string.
*
* @param obj Object
* @return String representation of the JSON
*/
public String serialize(Object obj) {
return gson.toJson(obj);
}
/**
* Deserialize the given JSON string to Java object.
*
* @param Type
* @param body The JSON string
* @param returnType The type to deserialize into
* @return The deserialized Java object
*/
@SuppressWarnings("unchecked")
public T deserialize(String body, Type returnType) {
try {
if (apiClient.isLenientOnJson()) {
JsonReader jsonReader = new JsonReader(new StringReader(body));
// see https://google-gson.googlecode.com/svn/trunk/gson/docs/javadocs/com/google/gson/stream/JsonReader.html#setLenient(boolean)
jsonReader.setLenient(true);
return gson.fromJson(jsonReader, returnType);
} else {
return gson.fromJson(body, returnType);
}
} catch (JsonParseException e) {
// Fallback processing when failed to parse JSON form response body:
// return the response body string directly for the String return type;
// parse response body into date or datetime for the Date return type.
if (returnType.equals(String.class))
return (T) body;
else if (returnType.equals(Date.class))
return (T) apiClient.parseDateOrDatetime(body);
else throw(e);
}
}
}
class DateAdapter implements JsonSerializer, JsonDeserializer {
private final ApiClient apiClient;
/**
* Constructor for DateAdapter
*
* @param apiClient Api client
*/
public DateAdapter(ApiClient apiClient) {
super();
this.apiClient = apiClient;
}
/**
* Serialize
*
* @param src Date
* @param typeOfSrc Type
* @param context Json Serialization Context
* @return Json Element
*/
@Override
public JsonElement serialize(Date src, Type typeOfSrc, JsonSerializationContext context) {
if (src == null) {
return JsonNull.INSTANCE;
} else {
return new JsonPrimitive(apiClient.formatDatetime(src));
}
}
/**
* Deserialize
*
* @param json Json element
* @param date Type
* @param context Json Serialization Context
* @return Date
* @throws JsonParseException if fail to parse
*/
@Override
public Date deserialize(JsonElement json, Type date, JsonDeserializationContext context) throws JsonParseException {
String str = json.getAsJsonPrimitive().getAsString();
try {
return apiClient.parseDateOrDatetime(str);
} catch (RuntimeException e) {
throw new JsonParseException(e);
}
}
}
/**
* Gson TypeAdapter for Joda DateTime type
*/
class DateTimeTypeAdapter extends TypeAdapter {
private final DateTimeFormatter parseFormatter = ISODateTimeFormat.dateOptionalTimeParser();
private final DateTimeFormatter printFormatter = ISODateTimeFormat.dateTime();
@Override
public void write(JsonWriter out, DateTime date) throws IOException {
if (date == null) {
out.nullValue();
} else {
out.value(printFormatter.print(date));
}
}
@Override
public DateTime read(JsonReader in) throws IOException {
switch (in.peek()) {
case NULL:
in.nextNull();
return null;
default:
String date = in.nextString();
return parseFormatter.parseDateTime(date);
}
}
}
/**
* Gson TypeAdapter for Joda LocalDate type
*/
class LocalDateTypeAdapter extends TypeAdapter {
private final DateTimeFormatter formatter = ISODateTimeFormat.date();
@Override
public void write(JsonWriter out, LocalDate date) throws IOException {
if (date == null) {
out.nullValue();
} else {
out.value(formatter.print(date));
}
}
@Override
public LocalDate read(JsonReader in) throws IOException {
switch (in.peek()) {
case NULL:
in.nextNull();
return null;
default:
String date = in.nextString();
return formatter.parseLocalDate(date);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy