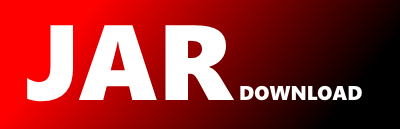
io.smooch.client.api.DeploymentApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api Show documentation
Show all versions of api Show documentation
io.smooch - api - 5.29.0
/*
* Smooch
* The Smooch API is a unified interface for powering messaging in your customer experiences across every channel. Our API speeds access to new markets, reduces time to ship, eliminates complexity, and helps you build the best experiences for your customers. For more information, visit our [official documentation](https://docs.smooch.io).
*
* OpenAPI spec version: 5.8
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.smooch.client.api;
import io.smooch.client.ApiCallback;
import io.smooch.client.ApiClient;
import io.smooch.client.ApiException;
import io.smooch.client.ApiResponse;
import io.smooch.client.Configuration;
import io.smooch.client.Pair;
import io.smooch.client.ProgressRequestBody;
import io.smooch.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import io.smooch.client.model.DeploymentActivatePhoneNumber;
import io.smooch.client.model.DeploymentConfirmCode;
import io.smooch.client.model.DeploymentCreate;
import io.smooch.client.model.DeploymentResponse;
import io.smooch.client.model.ListDeploymentsResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class DeploymentApi {
private ApiClient apiClient;
public DeploymentApi() {
this(Configuration.getDefaultApiClient());
}
public DeploymentApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for activatePhoneNumber
* @param deploymentId Identifies the deployment. (required)
* @param deploymentActivatePhoneNumberBody Body for an activatePhoneNumber request. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call activatePhoneNumberCall(String deploymentId, DeploymentActivatePhoneNumber deploymentActivatePhoneNumberBody, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = deploymentActivatePhoneNumberBody;
// create path and map variables
String localVarPath = "/v1.1/whatsapp/deployments/{deploymentId}/activate"
.replaceAll("\\{" + "deploymentId" + "\\}", apiClient.escapeString(deploymentId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "jwt" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call activatePhoneNumberValidateBeforeCall(String deploymentId, DeploymentActivatePhoneNumber deploymentActivatePhoneNumberBody, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'deploymentId' is set
if (deploymentId == null) {
throw new ApiException("Missing the required parameter 'deploymentId' when calling activatePhoneNumber(Async)");
}
// verify the required parameter 'deploymentActivatePhoneNumberBody' is set
if (deploymentActivatePhoneNumberBody == null) {
throw new ApiException("Missing the required parameter 'deploymentActivatePhoneNumberBody' when calling activatePhoneNumber(Async)");
}
com.squareup.okhttp.Call call = activatePhoneNumberCall(deploymentId, deploymentActivatePhoneNumberBody, progressListener, progressRequestListener);
return call;
}
/**
*
* Activate a phone number on the specified deployment.
* @param deploymentId Identifies the deployment. (required)
* @param deploymentActivatePhoneNumberBody Body for an activatePhoneNumber request. (required)
* @return DeploymentResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public DeploymentResponse activatePhoneNumber(String deploymentId, DeploymentActivatePhoneNumber deploymentActivatePhoneNumberBody) throws ApiException {
ApiResponse resp = activatePhoneNumberWithHttpInfo(deploymentId, deploymentActivatePhoneNumberBody);
return resp.getData();
}
/**
*
* Activate a phone number on the specified deployment.
* @param deploymentId Identifies the deployment. (required)
* @param deploymentActivatePhoneNumberBody Body for an activatePhoneNumber request. (required)
* @return ApiResponse<DeploymentResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse activatePhoneNumberWithHttpInfo(String deploymentId, DeploymentActivatePhoneNumber deploymentActivatePhoneNumberBody) throws ApiException {
com.squareup.okhttp.Call call = activatePhoneNumberValidateBeforeCall(deploymentId, deploymentActivatePhoneNumberBody, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Activate a phone number on the specified deployment.
* @param deploymentId Identifies the deployment. (required)
* @param deploymentActivatePhoneNumberBody Body for an activatePhoneNumber request. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call activatePhoneNumberAsync(String deploymentId, DeploymentActivatePhoneNumber deploymentActivatePhoneNumberBody, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = activatePhoneNumberValidateBeforeCall(deploymentId, deploymentActivatePhoneNumberBody, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for confirmCode
* @param deploymentId Identifies the deployment. (required)
* @param deploymentConfirmCode Body for a confirmCode request. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call confirmCodeCall(String deploymentId, DeploymentConfirmCode deploymentConfirmCode, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = deploymentConfirmCode;
// create path and map variables
String localVarPath = "/v1.1/whatsapp/deployments/{deploymentId}/code/confirm"
.replaceAll("\\{" + "deploymentId" + "\\}", apiClient.escapeString(deploymentId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "jwt" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call confirmCodeValidateBeforeCall(String deploymentId, DeploymentConfirmCode deploymentConfirmCode, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'deploymentId' is set
if (deploymentId == null) {
throw new ApiException("Missing the required parameter 'deploymentId' when calling confirmCode(Async)");
}
// verify the required parameter 'deploymentConfirmCode' is set
if (deploymentConfirmCode == null) {
throw new ApiException("Missing the required parameter 'deploymentConfirmCode' when calling confirmCode(Async)");
}
com.squareup.okhttp.Call call = confirmCodeCall(deploymentId, deploymentConfirmCode, progressListener, progressRequestListener);
return call;
}
/**
*
* Confirm code to complete phone number activation.
* @param deploymentId Identifies the deployment. (required)
* @param deploymentConfirmCode Body for a confirmCode request. (required)
* @return DeploymentResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public DeploymentResponse confirmCode(String deploymentId, DeploymentConfirmCode deploymentConfirmCode) throws ApiException {
ApiResponse resp = confirmCodeWithHttpInfo(deploymentId, deploymentConfirmCode);
return resp.getData();
}
/**
*
* Confirm code to complete phone number activation.
* @param deploymentId Identifies the deployment. (required)
* @param deploymentConfirmCode Body for a confirmCode request. (required)
* @return ApiResponse<DeploymentResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse confirmCodeWithHttpInfo(String deploymentId, DeploymentConfirmCode deploymentConfirmCode) throws ApiException {
com.squareup.okhttp.Call call = confirmCodeValidateBeforeCall(deploymentId, deploymentConfirmCode, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Confirm code to complete phone number activation.
* @param deploymentId Identifies the deployment. (required)
* @param deploymentConfirmCode Body for a confirmCode request. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call confirmCodeAsync(String deploymentId, DeploymentConfirmCode deploymentConfirmCode, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = confirmCodeValidateBeforeCall(deploymentId, deploymentConfirmCode, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for createDeployment
* @param deploymentCreateBody Body for a createDeployment request. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call createDeploymentCall(DeploymentCreate deploymentCreateBody, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = deploymentCreateBody;
// create path and map variables
String localVarPath = "/v1.1/whatsapp/deployments";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "jwt" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call createDeploymentValidateBeforeCall(DeploymentCreate deploymentCreateBody, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'deploymentCreateBody' is set
if (deploymentCreateBody == null) {
throw new ApiException("Missing the required parameter 'deploymentCreateBody' when calling createDeployment(Async)");
}
com.squareup.okhttp.Call call = createDeploymentCall(deploymentCreateBody, progressListener, progressRequestListener);
return call;
}
/**
*
* Create a WhatsApp deployment.
* @param deploymentCreateBody Body for a createDeployment request. (required)
* @return DeploymentResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public DeploymentResponse createDeployment(DeploymentCreate deploymentCreateBody) throws ApiException {
ApiResponse resp = createDeploymentWithHttpInfo(deploymentCreateBody);
return resp.getData();
}
/**
*
* Create a WhatsApp deployment.
* @param deploymentCreateBody Body for a createDeployment request. (required)
* @return ApiResponse<DeploymentResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createDeploymentWithHttpInfo(DeploymentCreate deploymentCreateBody) throws ApiException {
com.squareup.okhttp.Call call = createDeploymentValidateBeforeCall(deploymentCreateBody, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Create a WhatsApp deployment.
* @param deploymentCreateBody Body for a createDeployment request. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call createDeploymentAsync(DeploymentCreate deploymentCreateBody, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = createDeploymentValidateBeforeCall(deploymentCreateBody, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for deleteDeployment
* @param deploymentId Identifies the deployment. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call deleteDeploymentCall(String deploymentId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1.1/whatsapp/deployments/{deploymentId}"
.replaceAll("\\{" + "deploymentId" + "\\}", apiClient.escapeString(deploymentId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "jwt" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call deleteDeploymentValidateBeforeCall(String deploymentId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'deploymentId' is set
if (deploymentId == null) {
throw new ApiException("Missing the required parameter 'deploymentId' when calling deleteDeployment(Async)");
}
com.squareup.okhttp.Call call = deleteDeploymentCall(deploymentId, progressListener, progressRequestListener);
return call;
}
/**
*
* Delete the specified deployment.
* @param deploymentId Identifies the deployment. (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteDeployment(String deploymentId) throws ApiException {
deleteDeploymentWithHttpInfo(deploymentId);
}
/**
*
* Delete the specified deployment.
* @param deploymentId Identifies the deployment. (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteDeploymentWithHttpInfo(String deploymentId) throws ApiException {
com.squareup.okhttp.Call call = deleteDeploymentValidateBeforeCall(deploymentId, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
* Delete the specified deployment.
* @param deploymentId Identifies the deployment. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call deleteDeploymentAsync(String deploymentId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = deleteDeploymentValidateBeforeCall(deploymentId, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for getDeployment
* @param deploymentId Identifies the deployment. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getDeploymentCall(String deploymentId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1.1/whatsapp/deployments/{deploymentId}"
.replaceAll("\\{" + "deploymentId" + "\\}", apiClient.escapeString(deploymentId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "jwt" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getDeploymentValidateBeforeCall(String deploymentId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'deploymentId' is set
if (deploymentId == null) {
throw new ApiException("Missing the required parameter 'deploymentId' when calling getDeployment(Async)");
}
com.squareup.okhttp.Call call = getDeploymentCall(deploymentId, progressListener, progressRequestListener);
return call;
}
/**
*
* Get the specified deployment.
* @param deploymentId Identifies the deployment. (required)
* @return DeploymentResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public DeploymentResponse getDeployment(String deploymentId) throws ApiException {
ApiResponse resp = getDeploymentWithHttpInfo(deploymentId);
return resp.getData();
}
/**
*
* Get the specified deployment.
* @param deploymentId Identifies the deployment. (required)
* @return ApiResponse<DeploymentResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getDeploymentWithHttpInfo(String deploymentId) throws ApiException {
com.squareup.okhttp.Call call = getDeploymentValidateBeforeCall(deploymentId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Get the specified deployment.
* @param deploymentId Identifies the deployment. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getDeploymentAsync(String deploymentId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getDeploymentValidateBeforeCall(deploymentId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for listDeployments
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call listDeploymentsCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1.1/whatsapp/deployments";
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "jwt" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call listDeploymentsValidateBeforeCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
com.squareup.okhttp.Call call = listDeploymentsCall(progressListener, progressRequestListener);
return call;
}
/**
*
* List owned WhatsApp deployments.
* @return ListDeploymentsResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ListDeploymentsResponse listDeployments() throws ApiException {
ApiResponse resp = listDeploymentsWithHttpInfo();
return resp.getData();
}
/**
*
* List owned WhatsApp deployments.
* @return ApiResponse<ListDeploymentsResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse listDeploymentsWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = listDeploymentsValidateBeforeCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* List owned WhatsApp deployments.
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call listDeploymentsAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = listDeploymentsValidateBeforeCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy