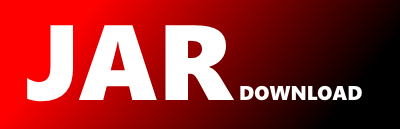
io.snappydata.thrift.BlobChunk Maven / Gradle / Ivy
/*
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
/*
* Changes for SnappyData data platform.
*
* Portions Copyright (c) 2018 SnappyData, Inc. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You
* may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License. See accompanying
* LICENSE file.
*/
package io.snappydata.thrift;
import java.nio.ByteBuffer;
import java.util.*;
import javax.annotation.Generated;
import com.gemstone.gemfire.internal.shared.BufferAllocator;
import com.gemstone.gemfire.internal.shared.ByteBufferReference;
import com.gemstone.gemfire.internal.shared.ClientSharedData;
import com.gemstone.gemfire.internal.shared.FetchRequest;
import io.snappydata.thrift.common.SocketTimeout;
import io.snappydata.thrift.common.TProtocolDirectBinary;
import io.snappydata.thrift.common.ThriftUtils;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.transport.TTransport;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2017-02-07")
public class BlobChunk implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("BlobChunk");
private static final org.apache.thrift.protocol.TField CHUNK_FIELD_DESC = new org.apache.thrift.protocol.TField("chunk", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField LAST_FIELD_DESC = new org.apache.thrift.protocol.TField("last", org.apache.thrift.protocol.TType.BOOL, (short)2);
private static final org.apache.thrift.protocol.TField LOB_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("lobId", org.apache.thrift.protocol.TType.I64, (short)3);
private static final org.apache.thrift.protocol.TField OFFSET_FIELD_DESC = new org.apache.thrift.protocol.TField("offset", org.apache.thrift.protocol.TType.I64, (short)4);
private static final org.apache.thrift.protocol.TField TOTAL_LENGTH_FIELD_DESC = new org.apache.thrift.protocol.TField("totalLength", org.apache.thrift.protocol.TType.I64, (short)5);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new BlobChunkStandardSchemeFactory());
schemes.put(TupleScheme.class, new BlobChunkTupleSchemeFactory());
}
public ByteBuffer chunk; // required
public boolean last; // required
public long lobId; // optional
public long offset; // optional
public long totalLength; // optional
/**
* Transient field to explicitly release reference to ByteBuffer
* (which is in column table region) after write. The "reference"
* part indicates the same that this is a direct handle to region value
* and hence reference count is increased/decreased to avoid having
* to read from disk in case the region value is removed in the interim.
*/
private transient ByteBufferReference chunkReference;
public BlobChunk(ByteBufferReference reference, boolean last) {
this();
assignChunkReference(reference);
this.last = last;
setLastIsSet(true);
}
private void assignChunkReference(ByteBufferReference reference) {
// chunkReference will be incremented and released at the time of write
this.chunk = ClientSharedData.NULL_BUFFER;
this.chunkReference = reference;
}
/**
* Mark the ByteBufferReference refCount as having been already incremented.
*/
public boolean initChunkFromReference() {
ByteBufferReference reference = this.chunkReference;
if (reference != null && this.chunk == ClientSharedData.NULL_BUFFER) {
this.chunk = reference.getBuffer();
return true;
} else {
return false;
}
}
public int size() {
return this.chunk != null ? this.chunk.remaining() : 0;
}
public void free() {
final ByteBufferReference reference = this.chunkReference;
if (reference != null) {
if (this.chunk != ClientSharedData.NULL_BUFFER) {
reference.release();
}
this.chunkReference = null;
} else if (this.chunk != null) {
BufferAllocator.releaseBuffer(this.chunk);
}
this.chunk = ClientSharedData.NULL_BUFFER;
}
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
CHUNK((short)1, "chunk"),
LAST((short)2, "last"),
LOB_ID((short)3, "lobId"),
OFFSET((short)4, "offset"),
TOTAL_LENGTH((short)5, "totalLength");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // CHUNK
return CHUNK;
case 2: // LAST
return LAST;
case 3: // LOB_ID
return LOB_ID;
case 4: // OFFSET
return OFFSET;
case 5: // TOTAL_LENGTH
return TOTAL_LENGTH;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __LAST_ISSET_ID = 0;
private static final int __LOBID_ISSET_ID = 1;
private static final int __OFFSET_ISSET_ID = 2;
private static final int __TOTALLENGTH_ISSET_ID = 3;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.LOB_ID,_Fields.OFFSET,_Fields.TOTAL_LENGTH};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.CHUNK, new org.apache.thrift.meta_data.FieldMetaData("chunk", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.LAST, new org.apache.thrift.meta_data.FieldMetaData("last", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.LOB_ID, new org.apache.thrift.meta_data.FieldMetaData("lobId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.OFFSET, new org.apache.thrift.meta_data.FieldMetaData("offset", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.TOTAL_LENGTH, new org.apache.thrift.meta_data.FieldMetaData("totalLength", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(BlobChunk.class, metaDataMap);
}
public BlobChunk() {
}
public BlobChunk(
ByteBuffer chunk,
boolean last)
{
this();
this.chunk = chunk;
this.last = last;
setLastIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public BlobChunk(BlobChunk other) {
__isset_bitfield = other.__isset_bitfield;
ByteBufferReference reference = other.chunkReference;
if (reference != null) {
assignChunkReference(reference);
} else if (other.chunk != null) {
// always own the chunk
if (other.chunk.isDirect()) {
this.chunk = ByteBuffer.wrap(ThriftUtils.toBytes(other.chunk));
} else {
this.chunk = other.chunk.duplicate();
}
}
this.last = other.last;
this.lobId = other.lobId;
this.offset = other.offset;
this.totalLength = other.totalLength;
}
public BlobChunk deepCopy() {
return new BlobChunk(this);
}
@Override
public void clear() {
free();
this.chunk = null;
setLastIsSet(false);
this.last = false;
setLobIdIsSet(false);
this.lobId = 0;
setOffsetIsSet(false);
this.offset = 0;
setTotalLengthIsSet(false);
this.totalLength = 0;
}
public byte[] getChunk() {
return ThriftUtils.toBytes(chunk);
}
public BlobChunk setChunk(byte[] chunk) {
free();
this.chunk = chunk == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(chunk, chunk.length));
return this;
}
public BlobChunk setChunk(ByteBuffer chunk) {
free();
this.chunk = chunk != null ? ByteBuffer.wrap(ThriftUtils.toBytes(chunk)) : null;
return this;
}
public void unsetChunk() {
this.chunk = null;
}
/** Returns true if field chunk is set (has been assigned a value) and false otherwise */
public boolean isSetChunk() {
return this.chunk != null;
}
public boolean isSetChunkReference() {
return this.chunkReference != null;
}
public void setChunkIsSet(boolean value) {
if (!value) {
free();
this.chunk = null;
}
}
public boolean isLast() {
return this.last;
}
public BlobChunk setLast(boolean last) {
this.last = last;
setLastIsSet(true);
return this;
}
public void unsetLast() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __LAST_ISSET_ID);
}
/** Returns true if field last is set (has been assigned a value) and false otherwise */
public boolean isSetLast() {
return EncodingUtils.testBit(__isset_bitfield, __LAST_ISSET_ID);
}
public void setLastIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __LAST_ISSET_ID, value);
}
public long getLobId() {
return this.lobId;
}
public BlobChunk setLobId(long lobId) {
this.lobId = lobId;
setLobIdIsSet(true);
return this;
}
public void unsetLobId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __LOBID_ISSET_ID);
}
/** Returns true if field lobId is set (has been assigned a value) and false otherwise */
public boolean isSetLobId() {
return EncodingUtils.testBit(__isset_bitfield, __LOBID_ISSET_ID);
}
public void setLobIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __LOBID_ISSET_ID, value);
}
public long getOffset() {
return this.offset;
}
public BlobChunk setOffset(long offset) {
this.offset = offset;
setOffsetIsSet(true);
return this;
}
public void unsetOffset() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __OFFSET_ISSET_ID);
}
/** Returns true if field offset is set (has been assigned a value) and false otherwise */
public boolean isSetOffset() {
return EncodingUtils.testBit(__isset_bitfield, __OFFSET_ISSET_ID);
}
public void setOffsetIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __OFFSET_ISSET_ID, value);
}
public long getTotalLength() {
return this.totalLength;
}
public BlobChunk setTotalLength(long totalLength) {
this.totalLength = totalLength;
setTotalLengthIsSet(true);
return this;
}
public void unsetTotalLength() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __TOTALLENGTH_ISSET_ID);
}
/** Returns true if field totalLength is set (has been assigned a value) and false otherwise */
public boolean isSetTotalLength() {
return EncodingUtils.testBit(__isset_bitfield, __TOTALLENGTH_ISSET_ID);
}
public void setTotalLengthIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __TOTALLENGTH_ISSET_ID, value);
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case CHUNK:
if (value == null) {
unsetChunk();
} else {
setChunk((ByteBuffer)value);
}
break;
case LAST:
if (value == null) {
unsetLast();
} else {
setLast((Boolean)value);
}
break;
case LOB_ID:
if (value == null) {
unsetLobId();
} else {
setLobId((Long)value);
}
break;
case OFFSET:
if (value == null) {
unsetOffset();
} else {
setOffset((Long)value);
}
break;
case TOTAL_LENGTH:
if (value == null) {
unsetTotalLength();
} else {
setTotalLength((Long)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case CHUNK:
return getChunk();
case LAST:
return isLast();
case LOB_ID:
return getLobId();
case OFFSET:
return getOffset();
case TOTAL_LENGTH:
return getTotalLength();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case CHUNK:
return isSetChunk();
case LAST:
return isSetLast();
case LOB_ID:
return isSetLobId();
case OFFSET:
return isSetOffset();
case TOTAL_LENGTH:
return isSetTotalLength();
}
throw new IllegalStateException();
}
/**
* Compress if sending to remote or else decompress if the blob can be
* stored in memory (so future reads will not need to decompress)
* else send as is.
*/
private ByteBuffer getBufferForWrite(org.apache.thrift.protocol.TProtocol oprot) {
final ByteBufferReference reference = this.chunkReference;
if (reference != null) {
TTransport transport = oprot.getTransport();
if (transport instanceof SocketTimeout) {
// check if a reference is already held
boolean hasOldReference = this.chunk != ClientSharedData.NULL_BUFFER;
if (((SocketTimeout)transport).isSocketToSameHost()) {
// try to decompress only if the value can be stored in region else
// avoid decompression overhead on server rather do on client/connector
if (hasOldReference) {
// release reference upfront if this not the only remaining reference
// (under the lock on value to ensure atomicity of check and release)
synchronized (reference) {
if (reference.referenceCount() > 1) {
reference.release();
hasOldReference = false;
}
this.chunkReference = reference.getValueRetain(
FetchRequest.DECOMPRESS_IF_IN_MEMORY);
}
} else {
this.chunkReference = reference.getValueRetain(
FetchRequest.DECOMPRESS_IF_IN_MEMORY);
}
if (this.chunkReference == null) {
this.chunkReference = reference.getValueRetain(FetchRequest.ORIGINAL);
}
} else {
// always send compressed to remote node
this.chunkReference = reference.getValueRetain(FetchRequest.COMPRESS);
}
this.chunk = this.chunkReference.getBuffer();
if (hasOldReference) reference.release();
} else if (this.chunk == ClientSharedData.NULL_BUFFER) {
this.chunk = reference.getBufferRetain();
}
}
return this.chunk;
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof BlobChunk)
return this.equals((BlobChunk)that);
return false;
}
public boolean equals(BlobChunk that) {
if (that == null)
return false;
final ByteBuffer thisChunk = this.chunk;
final ByteBuffer thatChunk = that.chunk;
boolean this_present_chunk = thisChunk != null;
boolean that_present_chunk = thatChunk != null;
if (this_present_chunk || that_present_chunk) {
if (!(this_present_chunk && that_present_chunk))
return false;
if (!thisChunk.equals(thatChunk))
return false;
}
boolean this_present_last = true;
boolean that_present_last = true;
if (this_present_last || that_present_last) {
if (!(this_present_last && that_present_last))
return false;
if (this.last != that.last)
return false;
}
boolean this_present_lobId = true && this.isSetLobId();
boolean that_present_lobId = true && that.isSetLobId();
if (this_present_lobId || that_present_lobId) {
if (!(this_present_lobId && that_present_lobId))
return false;
if (this.lobId != that.lobId)
return false;
}
boolean this_present_offset = true && this.isSetOffset();
boolean that_present_offset = true && that.isSetOffset();
if (this_present_offset || that_present_offset) {
if (!(this_present_offset && that_present_offset))
return false;
if (this.offset != that.offset)
return false;
}
boolean this_present_totalLength = true && this.isSetTotalLength();
boolean that_present_totalLength = true && that.isSetTotalLength();
if (this_present_totalLength || that_present_totalLength) {
if (!(this_present_totalLength && that_present_totalLength))
return false;
if (this.totalLength != that.totalLength)
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy