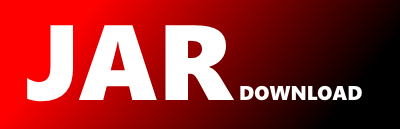
io.snappydata.thrift.OpenConnectionArgs Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package io.snappydata.thrift;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2018-12-10")
public class OpenConnectionArgs implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("OpenConnectionArgs");
private static final org.apache.thrift.protocol.TField CLIENT_HOST_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("clientHostName", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField CLIENT_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("clientID", org.apache.thrift.protocol.TType.STRING, (short)2);
private static final org.apache.thrift.protocol.TField SECURITY_FIELD_DESC = new org.apache.thrift.protocol.TField("security", org.apache.thrift.protocol.TType.I32, (short)3);
private static final org.apache.thrift.protocol.TField USER_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("userName", org.apache.thrift.protocol.TType.STRING, (short)4);
private static final org.apache.thrift.protocol.TField PASSWORD_FIELD_DESC = new org.apache.thrift.protocol.TField("password", org.apache.thrift.protocol.TType.STRING, (short)5);
private static final org.apache.thrift.protocol.TField FOR_XA_FIELD_DESC = new org.apache.thrift.protocol.TField("forXA", org.apache.thrift.protocol.TType.BOOL, (short)6);
private static final org.apache.thrift.protocol.TField TOKEN_SIZE_FIELD_DESC = new org.apache.thrift.protocol.TField("tokenSize", org.apache.thrift.protocol.TType.I32, (short)7);
private static final org.apache.thrift.protocol.TField USE_STRING_FOR_DECIMAL_FIELD_DESC = new org.apache.thrift.protocol.TField("useStringForDecimal", org.apache.thrift.protocol.TType.BOOL, (short)8);
private static final org.apache.thrift.protocol.TField PROPERTIES_FIELD_DESC = new org.apache.thrift.protocol.TField("properties", org.apache.thrift.protocol.TType.MAP, (short)9);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new OpenConnectionArgsStandardSchemeFactory());
schemes.put(TupleScheme.class, new OpenConnectionArgsTupleSchemeFactory());
}
public String clientHostName; // required
public String clientID; // required
/**
*
* @see SecurityMechanism
*/
public SecurityMechanism security; // required
public String userName; // optional
public String password; // optional
public boolean forXA; // optional
public int tokenSize; // optional
public boolean useStringForDecimal; // optional
public Map properties; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
CLIENT_HOST_NAME((short)1, "clientHostName"),
CLIENT_ID((short)2, "clientID"),
/**
*
* @see SecurityMechanism
*/
SECURITY((short)3, "security"),
USER_NAME((short)4, "userName"),
PASSWORD((short)5, "password"),
FOR_XA((short)6, "forXA"),
TOKEN_SIZE((short)7, "tokenSize"),
USE_STRING_FOR_DECIMAL((short)8, "useStringForDecimal"),
PROPERTIES((short)9, "properties");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // CLIENT_HOST_NAME
return CLIENT_HOST_NAME;
case 2: // CLIENT_ID
return CLIENT_ID;
case 3: // SECURITY
return SECURITY;
case 4: // USER_NAME
return USER_NAME;
case 5: // PASSWORD
return PASSWORD;
case 6: // FOR_XA
return FOR_XA;
case 7: // TOKEN_SIZE
return TOKEN_SIZE;
case 8: // USE_STRING_FOR_DECIMAL
return USE_STRING_FOR_DECIMAL;
case 9: // PROPERTIES
return PROPERTIES;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __FORXA_ISSET_ID = 0;
private static final int __TOKENSIZE_ISSET_ID = 1;
private static final int __USESTRINGFORDECIMAL_ISSET_ID = 2;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.USER_NAME,_Fields.PASSWORD,_Fields.FOR_XA,_Fields.TOKEN_SIZE,_Fields.USE_STRING_FOR_DECIMAL,_Fields.PROPERTIES};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.CLIENT_HOST_NAME, new org.apache.thrift.meta_data.FieldMetaData("clientHostName", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.CLIENT_ID, new org.apache.thrift.meta_data.FieldMetaData("clientID", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.SECURITY, new org.apache.thrift.meta_data.FieldMetaData("security", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, SecurityMechanism.class)));
tmpMap.put(_Fields.USER_NAME, new org.apache.thrift.meta_data.FieldMetaData("userName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.PASSWORD, new org.apache.thrift.meta_data.FieldMetaData("password", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.FOR_XA, new org.apache.thrift.meta_data.FieldMetaData("forXA", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.TOKEN_SIZE, new org.apache.thrift.meta_data.FieldMetaData("tokenSize", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.USE_STRING_FOR_DECIMAL, new org.apache.thrift.meta_data.FieldMetaData("useStringForDecimal", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.PROPERTIES, new org.apache.thrift.meta_data.FieldMetaData("properties", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.MapMetaData(org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING),
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(OpenConnectionArgs.class, metaDataMap);
}
public OpenConnectionArgs() {
}
public OpenConnectionArgs(
String clientHostName,
String clientID,
SecurityMechanism security)
{
this();
this.clientHostName = clientHostName;
this.clientID = clientID;
this.security = security;
}
/**
* Performs a deep copy on other.
*/
public OpenConnectionArgs(OpenConnectionArgs other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetClientHostName()) {
this.clientHostName = other.clientHostName;
}
if (other.isSetClientID()) {
this.clientID = other.clientID;
}
if (other.isSetSecurity()) {
this.security = other.security;
}
if (other.isSetUserName()) {
this.userName = other.userName;
}
if (other.isSetPassword()) {
this.password = other.password;
}
this.forXA = other.forXA;
this.tokenSize = other.tokenSize;
this.useStringForDecimal = other.useStringForDecimal;
if (other.isSetProperties()) {
Map __this__properties = new HashMap(other.properties);
this.properties = __this__properties;
}
}
public OpenConnectionArgs deepCopy() {
return new OpenConnectionArgs(this);
}
@Override
public void clear() {
this.clientHostName = null;
this.clientID = null;
this.security = null;
this.userName = null;
this.password = null;
setForXAIsSet(false);
this.forXA = false;
setTokenSizeIsSet(false);
this.tokenSize = 0;
setUseStringForDecimalIsSet(false);
this.useStringForDecimal = false;
this.properties = null;
}
public String getClientHostName() {
return this.clientHostName;
}
public OpenConnectionArgs setClientHostName(String clientHostName) {
this.clientHostName = clientHostName;
return this;
}
public void unsetClientHostName() {
this.clientHostName = null;
}
/** Returns true if field clientHostName is set (has been assigned a value) and false otherwise */
public boolean isSetClientHostName() {
return this.clientHostName != null;
}
public void setClientHostNameIsSet(boolean value) {
if (!value) {
this.clientHostName = null;
}
}
public String getClientID() {
return this.clientID;
}
public OpenConnectionArgs setClientID(String clientID) {
this.clientID = clientID;
return this;
}
public void unsetClientID() {
this.clientID = null;
}
/** Returns true if field clientID is set (has been assigned a value) and false otherwise */
public boolean isSetClientID() {
return this.clientID != null;
}
public void setClientIDIsSet(boolean value) {
if (!value) {
this.clientID = null;
}
}
/**
*
* @see SecurityMechanism
*/
public SecurityMechanism getSecurity() {
return this.security;
}
/**
*
* @see SecurityMechanism
*/
public OpenConnectionArgs setSecurity(SecurityMechanism security) {
this.security = security;
return this;
}
public void unsetSecurity() {
this.security = null;
}
/** Returns true if field security is set (has been assigned a value) and false otherwise */
public boolean isSetSecurity() {
return this.security != null;
}
public void setSecurityIsSet(boolean value) {
if (!value) {
this.security = null;
}
}
public String getUserName() {
return this.userName;
}
public OpenConnectionArgs setUserName(String userName) {
this.userName = userName;
return this;
}
public void unsetUserName() {
this.userName = null;
}
/** Returns true if field userName is set (has been assigned a value) and false otherwise */
public boolean isSetUserName() {
return this.userName != null;
}
public void setUserNameIsSet(boolean value) {
if (!value) {
this.userName = null;
}
}
public String getPassword() {
return this.password;
}
public OpenConnectionArgs setPassword(String password) {
this.password = password;
return this;
}
public void unsetPassword() {
this.password = null;
}
/** Returns true if field password is set (has been assigned a value) and false otherwise */
public boolean isSetPassword() {
return this.password != null;
}
public void setPasswordIsSet(boolean value) {
if (!value) {
this.password = null;
}
}
public boolean isForXA() {
return this.forXA;
}
public OpenConnectionArgs setForXA(boolean forXA) {
this.forXA = forXA;
setForXAIsSet(true);
return this;
}
public void unsetForXA() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __FORXA_ISSET_ID);
}
/** Returns true if field forXA is set (has been assigned a value) and false otherwise */
public boolean isSetForXA() {
return EncodingUtils.testBit(__isset_bitfield, __FORXA_ISSET_ID);
}
public void setForXAIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __FORXA_ISSET_ID, value);
}
public int getTokenSize() {
return this.tokenSize;
}
public OpenConnectionArgs setTokenSize(int tokenSize) {
this.tokenSize = tokenSize;
setTokenSizeIsSet(true);
return this;
}
public void unsetTokenSize() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __TOKENSIZE_ISSET_ID);
}
/** Returns true if field tokenSize is set (has been assigned a value) and false otherwise */
public boolean isSetTokenSize() {
return EncodingUtils.testBit(__isset_bitfield, __TOKENSIZE_ISSET_ID);
}
public void setTokenSizeIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __TOKENSIZE_ISSET_ID, value);
}
public boolean isUseStringForDecimal() {
return this.useStringForDecimal;
}
public OpenConnectionArgs setUseStringForDecimal(boolean useStringForDecimal) {
this.useStringForDecimal = useStringForDecimal;
setUseStringForDecimalIsSet(true);
return this;
}
public void unsetUseStringForDecimal() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __USESTRINGFORDECIMAL_ISSET_ID);
}
/** Returns true if field useStringForDecimal is set (has been assigned a value) and false otherwise */
public boolean isSetUseStringForDecimal() {
return EncodingUtils.testBit(__isset_bitfield, __USESTRINGFORDECIMAL_ISSET_ID);
}
public void setUseStringForDecimalIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __USESTRINGFORDECIMAL_ISSET_ID, value);
}
public int getPropertiesSize() {
return (this.properties == null) ? 0 : this.properties.size();
}
public void putToProperties(String key, String val) {
if (this.properties == null) {
this.properties = new HashMap();
}
this.properties.put(key, val);
}
public Map getProperties() {
return this.properties;
}
public OpenConnectionArgs setProperties(Map properties) {
this.properties = properties;
return this;
}
public void unsetProperties() {
this.properties = null;
}
/** Returns true if field properties is set (has been assigned a value) and false otherwise */
public boolean isSetProperties() {
return this.properties != null;
}
public void setPropertiesIsSet(boolean value) {
if (!value) {
this.properties = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case CLIENT_HOST_NAME:
if (value == null) {
unsetClientHostName();
} else {
setClientHostName((String)value);
}
break;
case CLIENT_ID:
if (value == null) {
unsetClientID();
} else {
setClientID((String)value);
}
break;
case SECURITY:
if (value == null) {
unsetSecurity();
} else {
setSecurity((SecurityMechanism)value);
}
break;
case USER_NAME:
if (value == null) {
unsetUserName();
} else {
setUserName((String)value);
}
break;
case PASSWORD:
if (value == null) {
unsetPassword();
} else {
setPassword((String)value);
}
break;
case FOR_XA:
if (value == null) {
unsetForXA();
} else {
setForXA((Boolean)value);
}
break;
case TOKEN_SIZE:
if (value == null) {
unsetTokenSize();
} else {
setTokenSize((Integer)value);
}
break;
case USE_STRING_FOR_DECIMAL:
if (value == null) {
unsetUseStringForDecimal();
} else {
setUseStringForDecimal((Boolean)value);
}
break;
case PROPERTIES:
if (value == null) {
unsetProperties();
} else {
setProperties((Map)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case CLIENT_HOST_NAME:
return getClientHostName();
case CLIENT_ID:
return getClientID();
case SECURITY:
return getSecurity();
case USER_NAME:
return getUserName();
case PASSWORD:
return getPassword();
case FOR_XA:
return isForXA();
case TOKEN_SIZE:
return getTokenSize();
case USE_STRING_FOR_DECIMAL:
return isUseStringForDecimal();
case PROPERTIES:
return getProperties();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case CLIENT_HOST_NAME:
return isSetClientHostName();
case CLIENT_ID:
return isSetClientID();
case SECURITY:
return isSetSecurity();
case USER_NAME:
return isSetUserName();
case PASSWORD:
return isSetPassword();
case FOR_XA:
return isSetForXA();
case TOKEN_SIZE:
return isSetTokenSize();
case USE_STRING_FOR_DECIMAL:
return isSetUseStringForDecimal();
case PROPERTIES:
return isSetProperties();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof OpenConnectionArgs)
return this.equals((OpenConnectionArgs)that);
return false;
}
public boolean equals(OpenConnectionArgs that) {
if (that == null)
return false;
boolean this_present_clientHostName = true && this.isSetClientHostName();
boolean that_present_clientHostName = true && that.isSetClientHostName();
if (this_present_clientHostName || that_present_clientHostName) {
if (!(this_present_clientHostName && that_present_clientHostName))
return false;
if (!this.clientHostName.equals(that.clientHostName))
return false;
}
boolean this_present_clientID = true && this.isSetClientID();
boolean that_present_clientID = true && that.isSetClientID();
if (this_present_clientID || that_present_clientID) {
if (!(this_present_clientID && that_present_clientID))
return false;
if (!this.clientID.equals(that.clientID))
return false;
}
boolean this_present_security = true && this.isSetSecurity();
boolean that_present_security = true && that.isSetSecurity();
if (this_present_security || that_present_security) {
if (!(this_present_security && that_present_security))
return false;
if (!this.security.equals(that.security))
return false;
}
boolean this_present_userName = true && this.isSetUserName();
boolean that_present_userName = true && that.isSetUserName();
if (this_present_userName || that_present_userName) {
if (!(this_present_userName && that_present_userName))
return false;
if (!this.userName.equals(that.userName))
return false;
}
boolean this_present_password = true && this.isSetPassword();
boolean that_present_password = true && that.isSetPassword();
if (this_present_password || that_present_password) {
if (!(this_present_password && that_present_password))
return false;
if (!this.password.equals(that.password))
return false;
}
boolean this_present_forXA = true && this.isSetForXA();
boolean that_present_forXA = true && that.isSetForXA();
if (this_present_forXA || that_present_forXA) {
if (!(this_present_forXA && that_present_forXA))
return false;
if (this.forXA != that.forXA)
return false;
}
boolean this_present_tokenSize = true && this.isSetTokenSize();
boolean that_present_tokenSize = true && that.isSetTokenSize();
if (this_present_tokenSize || that_present_tokenSize) {
if (!(this_present_tokenSize && that_present_tokenSize))
return false;
if (this.tokenSize != that.tokenSize)
return false;
}
boolean this_present_useStringForDecimal = true && this.isSetUseStringForDecimal();
boolean that_present_useStringForDecimal = true && that.isSetUseStringForDecimal();
if (this_present_useStringForDecimal || that_present_useStringForDecimal) {
if (!(this_present_useStringForDecimal && that_present_useStringForDecimal))
return false;
if (this.useStringForDecimal != that.useStringForDecimal)
return false;
}
boolean this_present_properties = true && this.isSetProperties();
boolean that_present_properties = true && that.isSetProperties();
if (this_present_properties || that_present_properties) {
if (!(this_present_properties && that_present_properties))
return false;
if (!this.properties.equals(that.properties))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy