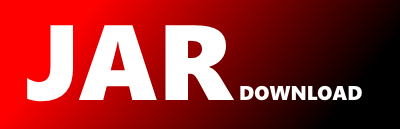
io.snappydata.thrift.ColumnDescriptor Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package io.snappydata.thrift;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-08-09")
public class ColumnDescriptor implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("ColumnDescriptor");
private static final org.apache.thrift.protocol.TField TYPE_FIELD_DESC = new org.apache.thrift.protocol.TField("type", org.apache.thrift.protocol.TType.I32, (short)1);
private static final org.apache.thrift.protocol.TField PRECISION_FIELD_DESC = new org.apache.thrift.protocol.TField("precision", org.apache.thrift.protocol.TType.I16, (short)2);
private static final org.apache.thrift.protocol.TField SCALE_FIELD_DESC = new org.apache.thrift.protocol.TField("scale", org.apache.thrift.protocol.TType.I16, (short)3);
private static final org.apache.thrift.protocol.TField NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("name", org.apache.thrift.protocol.TType.STRING, (short)4);
private static final org.apache.thrift.protocol.TField FULL_TABLE_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("fullTableName", org.apache.thrift.protocol.TType.STRING, (short)5);
private static final org.apache.thrift.protocol.TField UPDATABLE_FIELD_DESC = new org.apache.thrift.protocol.TField("updatable", org.apache.thrift.protocol.TType.BOOL, (short)6);
private static final org.apache.thrift.protocol.TField DEFINITELY_UPDATABLE_FIELD_DESC = new org.apache.thrift.protocol.TField("definitelyUpdatable", org.apache.thrift.protocol.TType.BOOL, (short)7);
private static final org.apache.thrift.protocol.TField NULLABLE_FIELD_DESC = new org.apache.thrift.protocol.TField("nullable", org.apache.thrift.protocol.TType.BOOL, (short)8);
private static final org.apache.thrift.protocol.TField AUTO_INCREMENT_FIELD_DESC = new org.apache.thrift.protocol.TField("autoIncrement", org.apache.thrift.protocol.TType.BOOL, (short)9);
private static final org.apache.thrift.protocol.TField PARAMETER_IN_FIELD_DESC = new org.apache.thrift.protocol.TField("parameterIn", org.apache.thrift.protocol.TType.BOOL, (short)10);
private static final org.apache.thrift.protocol.TField PARAMETER_OUT_FIELD_DESC = new org.apache.thrift.protocol.TField("parameterOut", org.apache.thrift.protocol.TType.BOOL, (short)11);
private static final org.apache.thrift.protocol.TField ELEMENT_TYPES_FIELD_DESC = new org.apache.thrift.protocol.TField("elementTypes", org.apache.thrift.protocol.TType.LIST, (short)12);
private static final org.apache.thrift.protocol.TField UDT_TYPE_AND_CLASS_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("udtTypeAndClassName", org.apache.thrift.protocol.TType.STRING, (short)13);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new ColumnDescriptorStandardSchemeFactory());
schemes.put(TupleScheme.class, new ColumnDescriptorTupleSchemeFactory());
}
/**
*
* @see SnappyType
*/
public SnappyType type; // required
public short precision; // required
public short scale; // optional
public String name; // optional
public String fullTableName; // optional
public boolean updatable; // optional
public boolean definitelyUpdatable; // optional
public boolean nullable; // optional
public boolean autoIncrement; // optional
public boolean parameterIn; // optional
public boolean parameterOut; // optional
public List elementTypes; // optional
public String udtTypeAndClassName; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
/**
*
* @see SnappyType
*/
TYPE((short)1, "type"),
PRECISION((short)2, "precision"),
SCALE((short)3, "scale"),
NAME((short)4, "name"),
FULL_TABLE_NAME((short)5, "fullTableName"),
UPDATABLE((short)6, "updatable"),
DEFINITELY_UPDATABLE((short)7, "definitelyUpdatable"),
NULLABLE((short)8, "nullable"),
AUTO_INCREMENT((short)9, "autoIncrement"),
PARAMETER_IN((short)10, "parameterIn"),
PARAMETER_OUT((short)11, "parameterOut"),
ELEMENT_TYPES((short)12, "elementTypes"),
UDT_TYPE_AND_CLASS_NAME((short)13, "udtTypeAndClassName");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // TYPE
return TYPE;
case 2: // PRECISION
return PRECISION;
case 3: // SCALE
return SCALE;
case 4: // NAME
return NAME;
case 5: // FULL_TABLE_NAME
return FULL_TABLE_NAME;
case 6: // UPDATABLE
return UPDATABLE;
case 7: // DEFINITELY_UPDATABLE
return DEFINITELY_UPDATABLE;
case 8: // NULLABLE
return NULLABLE;
case 9: // AUTO_INCREMENT
return AUTO_INCREMENT;
case 10: // PARAMETER_IN
return PARAMETER_IN;
case 11: // PARAMETER_OUT
return PARAMETER_OUT;
case 12: // ELEMENT_TYPES
return ELEMENT_TYPES;
case 13: // UDT_TYPE_AND_CLASS_NAME
return UDT_TYPE_AND_CLASS_NAME;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __PRECISION_ISSET_ID = 0;
private static final int __SCALE_ISSET_ID = 1;
private static final int __UPDATABLE_ISSET_ID = 2;
private static final int __DEFINITELYUPDATABLE_ISSET_ID = 3;
private static final int __NULLABLE_ISSET_ID = 4;
private static final int __AUTOINCREMENT_ISSET_ID = 5;
private static final int __PARAMETERIN_ISSET_ID = 6;
private static final int __PARAMETEROUT_ISSET_ID = 7;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.SCALE,_Fields.NAME,_Fields.FULL_TABLE_NAME,_Fields.UPDATABLE,_Fields.DEFINITELY_UPDATABLE,_Fields.NULLABLE,_Fields.AUTO_INCREMENT,_Fields.PARAMETER_IN,_Fields.PARAMETER_OUT,_Fields.ELEMENT_TYPES,_Fields.UDT_TYPE_AND_CLASS_NAME};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.TYPE, new org.apache.thrift.meta_data.FieldMetaData("type", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, SnappyType.class)));
tmpMap.put(_Fields.PRECISION, new org.apache.thrift.meta_data.FieldMetaData("precision", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I16)));
tmpMap.put(_Fields.SCALE, new org.apache.thrift.meta_data.FieldMetaData("scale", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I16)));
tmpMap.put(_Fields.NAME, new org.apache.thrift.meta_data.FieldMetaData("name", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.FULL_TABLE_NAME, new org.apache.thrift.meta_data.FieldMetaData("fullTableName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.UPDATABLE, new org.apache.thrift.meta_data.FieldMetaData("updatable", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.DEFINITELY_UPDATABLE, new org.apache.thrift.meta_data.FieldMetaData("definitelyUpdatable", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.NULLABLE, new org.apache.thrift.meta_data.FieldMetaData("nullable", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.AUTO_INCREMENT, new org.apache.thrift.meta_data.FieldMetaData("autoIncrement", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.PARAMETER_IN, new org.apache.thrift.meta_data.FieldMetaData("parameterIn", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.PARAMETER_OUT, new org.apache.thrift.meta_data.FieldMetaData("parameterOut", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.ELEMENT_TYPES, new org.apache.thrift.meta_data.FieldMetaData("elementTypes", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, SnappyType.class))));
tmpMap.put(_Fields.UDT_TYPE_AND_CLASS_NAME, new org.apache.thrift.meta_data.FieldMetaData("udtTypeAndClassName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(ColumnDescriptor.class, metaDataMap);
}
public ColumnDescriptor() {
}
public ColumnDescriptor(
SnappyType type,
short precision)
{
this();
this.type = type;
this.precision = precision;
setPrecisionIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public ColumnDescriptor(ColumnDescriptor other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetType()) {
this.type = other.type;
}
this.precision = other.precision;
this.scale = other.scale;
if (other.isSetName()) {
this.name = other.name;
}
if (other.isSetFullTableName()) {
this.fullTableName = other.fullTableName;
}
this.updatable = other.updatable;
this.definitelyUpdatable = other.definitelyUpdatable;
this.nullable = other.nullable;
this.autoIncrement = other.autoIncrement;
this.parameterIn = other.parameterIn;
this.parameterOut = other.parameterOut;
if (other.isSetElementTypes()) {
List __this__elementTypes = new ArrayList(other.elementTypes.size());
for (SnappyType other_element : other.elementTypes) {
__this__elementTypes.add(other_element);
}
this.elementTypes = __this__elementTypes;
}
if (other.isSetUdtTypeAndClassName()) {
this.udtTypeAndClassName = other.udtTypeAndClassName;
}
}
public ColumnDescriptor deepCopy() {
return new ColumnDescriptor(this);
}
@Override
public void clear() {
this.type = null;
setPrecisionIsSet(false);
this.precision = 0;
setScaleIsSet(false);
this.scale = 0;
this.name = null;
this.fullTableName = null;
setUpdatableIsSet(false);
this.updatable = false;
setDefinitelyUpdatableIsSet(false);
this.definitelyUpdatable = false;
setNullableIsSet(false);
this.nullable = false;
setAutoIncrementIsSet(false);
this.autoIncrement = false;
setParameterInIsSet(false);
this.parameterIn = false;
setParameterOutIsSet(false);
this.parameterOut = false;
this.elementTypes = null;
this.udtTypeAndClassName = null;
}
/**
*
* @see SnappyType
*/
public SnappyType getType() {
return this.type;
}
/**
*
* @see SnappyType
*/
public ColumnDescriptor setType(SnappyType type) {
this.type = type;
return this;
}
public void unsetType() {
this.type = null;
}
/** Returns true if field type is set (has been assigned a value) and false otherwise */
public boolean isSetType() {
return this.type != null;
}
public void setTypeIsSet(boolean value) {
if (!value) {
this.type = null;
}
}
public short getPrecision() {
return this.precision;
}
public ColumnDescriptor setPrecision(short precision) {
this.precision = precision;
setPrecisionIsSet(true);
return this;
}
public void unsetPrecision() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __PRECISION_ISSET_ID);
}
/** Returns true if field precision is set (has been assigned a value) and false otherwise */
public boolean isSetPrecision() {
return EncodingUtils.testBit(__isset_bitfield, __PRECISION_ISSET_ID);
}
public void setPrecisionIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __PRECISION_ISSET_ID, value);
}
public short getScale() {
return this.scale;
}
public ColumnDescriptor setScale(short scale) {
this.scale = scale;
setScaleIsSet(true);
return this;
}
public void unsetScale() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SCALE_ISSET_ID);
}
/** Returns true if field scale is set (has been assigned a value) and false otherwise */
public boolean isSetScale() {
return EncodingUtils.testBit(__isset_bitfield, __SCALE_ISSET_ID);
}
public void setScaleIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SCALE_ISSET_ID, value);
}
public String getName() {
return this.name;
}
public ColumnDescriptor setName(String name) {
this.name = name;
return this;
}
public void unsetName() {
this.name = null;
}
/** Returns true if field name is set (has been assigned a value) and false otherwise */
public boolean isSetName() {
return this.name != null;
}
public void setNameIsSet(boolean value) {
if (!value) {
this.name = null;
}
}
public String getFullTableName() {
return this.fullTableName;
}
public ColumnDescriptor setFullTableName(String fullTableName) {
this.fullTableName = fullTableName;
return this;
}
public void unsetFullTableName() {
this.fullTableName = null;
}
/** Returns true if field fullTableName is set (has been assigned a value) and false otherwise */
public boolean isSetFullTableName() {
return this.fullTableName != null;
}
public void setFullTableNameIsSet(boolean value) {
if (!value) {
this.fullTableName = null;
}
}
public boolean isUpdatable() {
return this.updatable;
}
public ColumnDescriptor setUpdatable(boolean updatable) {
this.updatable = updatable;
setUpdatableIsSet(true);
return this;
}
public void unsetUpdatable() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __UPDATABLE_ISSET_ID);
}
/** Returns true if field updatable is set (has been assigned a value) and false otherwise */
public boolean isSetUpdatable() {
return EncodingUtils.testBit(__isset_bitfield, __UPDATABLE_ISSET_ID);
}
public void setUpdatableIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __UPDATABLE_ISSET_ID, value);
}
public boolean isDefinitelyUpdatable() {
return this.definitelyUpdatable;
}
public ColumnDescriptor setDefinitelyUpdatable(boolean definitelyUpdatable) {
this.definitelyUpdatable = definitelyUpdatable;
setDefinitelyUpdatableIsSet(true);
return this;
}
public void unsetDefinitelyUpdatable() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __DEFINITELYUPDATABLE_ISSET_ID);
}
/** Returns true if field definitelyUpdatable is set (has been assigned a value) and false otherwise */
public boolean isSetDefinitelyUpdatable() {
return EncodingUtils.testBit(__isset_bitfield, __DEFINITELYUPDATABLE_ISSET_ID);
}
public void setDefinitelyUpdatableIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __DEFINITELYUPDATABLE_ISSET_ID, value);
}
public boolean isNullable() {
return this.nullable;
}
public ColumnDescriptor setNullable(boolean nullable) {
this.nullable = nullable;
setNullableIsSet(true);
return this;
}
public void unsetNullable() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __NULLABLE_ISSET_ID);
}
/** Returns true if field nullable is set (has been assigned a value) and false otherwise */
public boolean isSetNullable() {
return EncodingUtils.testBit(__isset_bitfield, __NULLABLE_ISSET_ID);
}
public void setNullableIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __NULLABLE_ISSET_ID, value);
}
public boolean isAutoIncrement() {
return this.autoIncrement;
}
public ColumnDescriptor setAutoIncrement(boolean autoIncrement) {
this.autoIncrement = autoIncrement;
setAutoIncrementIsSet(true);
return this;
}
public void unsetAutoIncrement() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __AUTOINCREMENT_ISSET_ID);
}
/** Returns true if field autoIncrement is set (has been assigned a value) and false otherwise */
public boolean isSetAutoIncrement() {
return EncodingUtils.testBit(__isset_bitfield, __AUTOINCREMENT_ISSET_ID);
}
public void setAutoIncrementIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __AUTOINCREMENT_ISSET_ID, value);
}
public boolean isParameterIn() {
return this.parameterIn;
}
public ColumnDescriptor setParameterIn(boolean parameterIn) {
this.parameterIn = parameterIn;
setParameterInIsSet(true);
return this;
}
public void unsetParameterIn() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __PARAMETERIN_ISSET_ID);
}
/** Returns true if field parameterIn is set (has been assigned a value) and false otherwise */
public boolean isSetParameterIn() {
return EncodingUtils.testBit(__isset_bitfield, __PARAMETERIN_ISSET_ID);
}
public void setParameterInIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __PARAMETERIN_ISSET_ID, value);
}
public boolean isParameterOut() {
return this.parameterOut;
}
public ColumnDescriptor setParameterOut(boolean parameterOut) {
this.parameterOut = parameterOut;
setParameterOutIsSet(true);
return this;
}
public void unsetParameterOut() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __PARAMETEROUT_ISSET_ID);
}
/** Returns true if field parameterOut is set (has been assigned a value) and false otherwise */
public boolean isSetParameterOut() {
return EncodingUtils.testBit(__isset_bitfield, __PARAMETEROUT_ISSET_ID);
}
public void setParameterOutIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __PARAMETEROUT_ISSET_ID, value);
}
public int getElementTypesSize() {
return (this.elementTypes == null) ? 0 : this.elementTypes.size();
}
public java.util.Iterator getElementTypesIterator() {
return (this.elementTypes == null) ? null : this.elementTypes.iterator();
}
public void addToElementTypes(SnappyType elem) {
if (this.elementTypes == null) {
this.elementTypes = new ArrayList();
}
this.elementTypes.add(elem);
}
public List getElementTypes() {
return this.elementTypes;
}
public ColumnDescriptor setElementTypes(List elementTypes) {
this.elementTypes = elementTypes;
return this;
}
public void unsetElementTypes() {
this.elementTypes = null;
}
/** Returns true if field elementTypes is set (has been assigned a value) and false otherwise */
public boolean isSetElementTypes() {
return this.elementTypes != null;
}
public void setElementTypesIsSet(boolean value) {
if (!value) {
this.elementTypes = null;
}
}
public String getUdtTypeAndClassName() {
return this.udtTypeAndClassName;
}
public ColumnDescriptor setUdtTypeAndClassName(String udtTypeAndClassName) {
this.udtTypeAndClassName = udtTypeAndClassName;
return this;
}
public void unsetUdtTypeAndClassName() {
this.udtTypeAndClassName = null;
}
/** Returns true if field udtTypeAndClassName is set (has been assigned a value) and false otherwise */
public boolean isSetUdtTypeAndClassName() {
return this.udtTypeAndClassName != null;
}
public void setUdtTypeAndClassNameIsSet(boolean value) {
if (!value) {
this.udtTypeAndClassName = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case TYPE:
if (value == null) {
unsetType();
} else {
setType((SnappyType)value);
}
break;
case PRECISION:
if (value == null) {
unsetPrecision();
} else {
setPrecision((Short)value);
}
break;
case SCALE:
if (value == null) {
unsetScale();
} else {
setScale((Short)value);
}
break;
case NAME:
if (value == null) {
unsetName();
} else {
setName((String)value);
}
break;
case FULL_TABLE_NAME:
if (value == null) {
unsetFullTableName();
} else {
setFullTableName((String)value);
}
break;
case UPDATABLE:
if (value == null) {
unsetUpdatable();
} else {
setUpdatable((Boolean)value);
}
break;
case DEFINITELY_UPDATABLE:
if (value == null) {
unsetDefinitelyUpdatable();
} else {
setDefinitelyUpdatable((Boolean)value);
}
break;
case NULLABLE:
if (value == null) {
unsetNullable();
} else {
setNullable((Boolean)value);
}
break;
case AUTO_INCREMENT:
if (value == null) {
unsetAutoIncrement();
} else {
setAutoIncrement((Boolean)value);
}
break;
case PARAMETER_IN:
if (value == null) {
unsetParameterIn();
} else {
setParameterIn((Boolean)value);
}
break;
case PARAMETER_OUT:
if (value == null) {
unsetParameterOut();
} else {
setParameterOut((Boolean)value);
}
break;
case ELEMENT_TYPES:
if (value == null) {
unsetElementTypes();
} else {
setElementTypes((List)value);
}
break;
case UDT_TYPE_AND_CLASS_NAME:
if (value == null) {
unsetUdtTypeAndClassName();
} else {
setUdtTypeAndClassName((String)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case TYPE:
return getType();
case PRECISION:
return getPrecision();
case SCALE:
return getScale();
case NAME:
return getName();
case FULL_TABLE_NAME:
return getFullTableName();
case UPDATABLE:
return isUpdatable();
case DEFINITELY_UPDATABLE:
return isDefinitelyUpdatable();
case NULLABLE:
return isNullable();
case AUTO_INCREMENT:
return isAutoIncrement();
case PARAMETER_IN:
return isParameterIn();
case PARAMETER_OUT:
return isParameterOut();
case ELEMENT_TYPES:
return getElementTypes();
case UDT_TYPE_AND_CLASS_NAME:
return getUdtTypeAndClassName();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case TYPE:
return isSetType();
case PRECISION:
return isSetPrecision();
case SCALE:
return isSetScale();
case NAME:
return isSetName();
case FULL_TABLE_NAME:
return isSetFullTableName();
case UPDATABLE:
return isSetUpdatable();
case DEFINITELY_UPDATABLE:
return isSetDefinitelyUpdatable();
case NULLABLE:
return isSetNullable();
case AUTO_INCREMENT:
return isSetAutoIncrement();
case PARAMETER_IN:
return isSetParameterIn();
case PARAMETER_OUT:
return isSetParameterOut();
case ELEMENT_TYPES:
return isSetElementTypes();
case UDT_TYPE_AND_CLASS_NAME:
return isSetUdtTypeAndClassName();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof ColumnDescriptor)
return this.equals((ColumnDescriptor)that);
return false;
}
public boolean equals(ColumnDescriptor that) {
if (that == null)
return false;
boolean this_present_type = true && this.isSetType();
boolean that_present_type = true && that.isSetType();
if (this_present_type || that_present_type) {
if (!(this_present_type && that_present_type))
return false;
if (!this.type.equals(that.type))
return false;
}
boolean this_present_precision = true;
boolean that_present_precision = true;
if (this_present_precision || that_present_precision) {
if (!(this_present_precision && that_present_precision))
return false;
if (this.precision != that.precision)
return false;
}
boolean this_present_scale = true && this.isSetScale();
boolean that_present_scale = true && that.isSetScale();
if (this_present_scale || that_present_scale) {
if (!(this_present_scale && that_present_scale))
return false;
if (this.scale != that.scale)
return false;
}
boolean this_present_name = true && this.isSetName();
boolean that_present_name = true && that.isSetName();
if (this_present_name || that_present_name) {
if (!(this_present_name && that_present_name))
return false;
if (!this.name.equals(that.name))
return false;
}
boolean this_present_fullTableName = true && this.isSetFullTableName();
boolean that_present_fullTableName = true && that.isSetFullTableName();
if (this_present_fullTableName || that_present_fullTableName) {
if (!(this_present_fullTableName && that_present_fullTableName))
return false;
if (!this.fullTableName.equals(that.fullTableName))
return false;
}
boolean this_present_updatable = true && this.isSetUpdatable();
boolean that_present_updatable = true && that.isSetUpdatable();
if (this_present_updatable || that_present_updatable) {
if (!(this_present_updatable && that_present_updatable))
return false;
if (this.updatable != that.updatable)
return false;
}
boolean this_present_definitelyUpdatable = true && this.isSetDefinitelyUpdatable();
boolean that_present_definitelyUpdatable = true && that.isSetDefinitelyUpdatable();
if (this_present_definitelyUpdatable || that_present_definitelyUpdatable) {
if (!(this_present_definitelyUpdatable && that_present_definitelyUpdatable))
return false;
if (this.definitelyUpdatable != that.definitelyUpdatable)
return false;
}
boolean this_present_nullable = true && this.isSetNullable();
boolean that_present_nullable = true && that.isSetNullable();
if (this_present_nullable || that_present_nullable) {
if (!(this_present_nullable && that_present_nullable))
return false;
if (this.nullable != that.nullable)
return false;
}
boolean this_present_autoIncrement = true && this.isSetAutoIncrement();
boolean that_present_autoIncrement = true && that.isSetAutoIncrement();
if (this_present_autoIncrement || that_present_autoIncrement) {
if (!(this_present_autoIncrement && that_present_autoIncrement))
return false;
if (this.autoIncrement != that.autoIncrement)
return false;
}
boolean this_present_parameterIn = true && this.isSetParameterIn();
boolean that_present_parameterIn = true && that.isSetParameterIn();
if (this_present_parameterIn || that_present_parameterIn) {
if (!(this_present_parameterIn && that_present_parameterIn))
return false;
if (this.parameterIn != that.parameterIn)
return false;
}
boolean this_present_parameterOut = true && this.isSetParameterOut();
boolean that_present_parameterOut = true && that.isSetParameterOut();
if (this_present_parameterOut || that_present_parameterOut) {
if (!(this_present_parameterOut && that_present_parameterOut))
return false;
if (this.parameterOut != that.parameterOut)
return false;
}
boolean this_present_elementTypes = true && this.isSetElementTypes();
boolean that_present_elementTypes = true && that.isSetElementTypes();
if (this_present_elementTypes || that_present_elementTypes) {
if (!(this_present_elementTypes && that_present_elementTypes))
return false;
if (!this.elementTypes.equals(that.elementTypes))
return false;
}
boolean this_present_udtTypeAndClassName = true && this.isSetUdtTypeAndClassName();
boolean that_present_udtTypeAndClassName = true && that.isSetUdtTypeAndClassName();
if (this_present_udtTypeAndClassName || that_present_udtTypeAndClassName) {
if (!(this_present_udtTypeAndClassName && that_present_udtTypeAndClassName))
return false;
if (!this.udtTypeAndClassName.equals(that.udtTypeAndClassName))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy