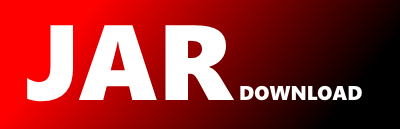
io.snappydata.thrift.ColumnValue Maven / Gradle / Ivy
/*
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
/*
* Changes for SnappyData data platform.
*
* Portions Copyright (c) 2017-2019 TIBCO Software Inc. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you
* may not use this file except in compliance with the License. You
* may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
* implied. See the License for the specific language governing
* permissions and limitations under the License. See accompanying
* LICENSE file.
*/
package io.snappydata.thrift;
import java.nio.ByteBuffer;
import java.util.*;
import io.snappydata.thrift.common.ThriftUtils;
import org.apache.thrift.TBaseHelper;
import org.apache.thrift.TException;
import org.apache.thrift.protocol.TProtocol;
import org.apache.thrift.protocol.TProtocolException;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
public final class ColumnValue extends org.apache.thrift.TUnion {
public static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("ColumnValue");
public static final org.apache.thrift.protocol.TField BOOL_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("bool_val", org.apache.thrift.protocol.TType.BOOL, (short)1);
public static final org.apache.thrift.protocol.TField BYTE_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("byte_val", org.apache.thrift.protocol.TType.BYTE, (short)2);
public static final org.apache.thrift.protocol.TField I16_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("i16_val", org.apache.thrift.protocol.TType.I16, (short)3);
public static final org.apache.thrift.protocol.TField I32_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("i32_val", org.apache.thrift.protocol.TType.I32, (short)4);
public static final org.apache.thrift.protocol.TField I64_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("i64_val", org.apache.thrift.protocol.TType.I64, (short)5);
public static final org.apache.thrift.protocol.TField FLOAT_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("float_val", org.apache.thrift.protocol.TType.I32, (short)6);
public static final org.apache.thrift.protocol.TField DOUBLE_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("double_val", org.apache.thrift.protocol.TType.DOUBLE, (short)7);
public static final org.apache.thrift.protocol.TField STRING_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("string_val", org.apache.thrift.protocol.TType.STRING, (short)8);
public static final org.apache.thrift.protocol.TField DECIMAL_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("decimal_val", org.apache.thrift.protocol.TType.STRUCT, (short)9);
public static final org.apache.thrift.protocol.TField DATE_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("date_val", org.apache.thrift.protocol.TType.I64, (short)10);
public static final org.apache.thrift.protocol.TField TIME_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("time_val", org.apache.thrift.protocol.TType.I64, (short)11);
public static final org.apache.thrift.protocol.TField TIMESTAMP_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("timestamp_val", org.apache.thrift.protocol.TType.I64, (short)12);
public static final org.apache.thrift.protocol.TField BINARY_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("binary_val", org.apache.thrift.protocol.TType.STRING, (short)13);
public static final org.apache.thrift.protocol.TField BLOB_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("blob_val", org.apache.thrift.protocol.TType.STRUCT, (short)14);
public static final org.apache.thrift.protocol.TField CLOB_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("clob_val", org.apache.thrift.protocol.TType.STRUCT, (short)15);
public static final org.apache.thrift.protocol.TField ARRAY_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("array_val", org.apache.thrift.protocol.TType.LIST, (short)16);
public static final org.apache.thrift.protocol.TField MAP_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("map_val", org.apache.thrift.protocol.TType.MAP, (short)17);
public static final org.apache.thrift.protocol.TField STRUCT_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("struct_val", org.apache.thrift.protocol.TType.LIST, (short)18);
public static final org.apache.thrift.protocol.TField NULL_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("null_val", org.apache.thrift.protocol.TType.BOOL, (short)19);
public static final org.apache.thrift.protocol.TField JAVA_VAL_FIELD_DESC = new org.apache.thrift.protocol.TField("java_val", org.apache.thrift.protocol.TType.STRING, (short)20);
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
BOOL_VAL((short)1, "bool_val"),
BYTE_VAL((short)2, "byte_val"),
I16_VAL((short)3, "i16_val"),
I32_VAL((short)4, "i32_val"),
I64_VAL((short)5, "i64_val"),
FLOAT_VAL((short)6, "float_val"),
DOUBLE_VAL((short)7, "double_val"),
STRING_VAL((short)8, "string_val"),
DECIMAL_VAL((short)9, "decimal_val"),
DATE_VAL((short)10, "date_val"),
TIME_VAL((short)11, "time_val"),
TIMESTAMP_VAL((short)12, "timestamp_val"),
BINARY_VAL((short)13, "binary_val"),
BLOB_VAL((short)14, "blob_val"),
CLOB_VAL((short)15, "clob_val"),
ARRAY_VAL((short)16, "array_val"),
MAP_VAL((short)17, "map_val"),
STRUCT_VAL((short)18, "struct_val"),
NULL_VAL((short)19, "null_val"),
JAVA_VAL((short)20, "java_val");
private static final Map byName = new HashMap<>();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // BOOL_VAL
return BOOL_VAL;
case 2: // BYTE_VAL
return BYTE_VAL;
case 3: // I16_VAL
return I16_VAL;
case 4: // I32_VAL
return I32_VAL;
case 5: // I64_VAL
return I64_VAL;
case 6: // FLOAT_VAL
return FLOAT_VAL;
case 7: // DOUBLE_VAL
return DOUBLE_VAL;
case 8: // STRING_VAL
return STRING_VAL;
case 9: // DECIMAL_VAL
return DECIMAL_VAL;
case 10: // DATE_VAL
return DATE_VAL;
case 11: // TIME_VAL
return TIME_VAL;
case 12: // TIMESTAMP_VAL
return TIMESTAMP_VAL;
case 13: // BINARY_VAL
return BINARY_VAL;
case 14: // BLOB_VAL
return BLOB_VAL;
case 15: // CLOB_VAL
return CLOB_VAL;
case 16: // ARRAY_VAL
return ARRAY_VAL;
case 17: // MAP_VAL
return MAP_VAL;
case 18: // STRUCT_VAL
return STRUCT_VAL;
case 19: // NULL_VAL
return NULL_VAL;
case 20: // JAVA_VAL
return JAVA_VAL;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<>(_Fields.class);
tmpMap.put(_Fields.BOOL_VAL, new org.apache.thrift.meta_data.FieldMetaData("bool_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.BYTE_VAL, new org.apache.thrift.meta_data.FieldMetaData("byte_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BYTE)));
tmpMap.put(_Fields.I16_VAL, new org.apache.thrift.meta_data.FieldMetaData("i16_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I16)));
tmpMap.put(_Fields.I32_VAL, new org.apache.thrift.meta_data.FieldMetaData("i32_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.I64_VAL, new org.apache.thrift.meta_data.FieldMetaData("i64_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.FLOAT_VAL, new org.apache.thrift.meta_data.FieldMetaData("float_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.DOUBLE_VAL, new org.apache.thrift.meta_data.FieldMetaData("double_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.DOUBLE)));
tmpMap.put(_Fields.STRING_VAL, new org.apache.thrift.meta_data.FieldMetaData("string_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.DECIMAL_VAL, new org.apache.thrift.meta_data.FieldMetaData("decimal_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Decimal.class)));
tmpMap.put(_Fields.DATE_VAL, new org.apache.thrift.meta_data.FieldMetaData("date_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.TIME_VAL, new org.apache.thrift.meta_data.FieldMetaData("time_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.TIMESTAMP_VAL, new org.apache.thrift.meta_data.FieldMetaData("timestamp_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.BINARY_VAL, new org.apache.thrift.meta_data.FieldMetaData("binary_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.BLOB_VAL, new org.apache.thrift.meta_data.FieldMetaData("blob_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, BlobChunk.class)));
tmpMap.put(_Fields.CLOB_VAL, new org.apache.thrift.meta_data.FieldMetaData("clob_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, ClobChunk.class)));
tmpMap.put(_Fields.ARRAY_VAL, new org.apache.thrift.meta_data.FieldMetaData("array_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "ColumnValue"))));
tmpMap.put(_Fields.MAP_VAL, new org.apache.thrift.meta_data.FieldMetaData("map_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.MapMetaData(org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "ColumnValue"),
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "ColumnValue"))));
tmpMap.put(_Fields.STRUCT_VAL, new org.apache.thrift.meta_data.FieldMetaData("struct_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "ColumnValue"))));
tmpMap.put(_Fields.NULL_VAL, new org.apache.thrift.meta_data.FieldMetaData("null_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.JAVA_VAL, new org.apache.thrift.meta_data.FieldMetaData("java_val", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(ColumnValue.class, metaDataMap);
}
/** prevent boxing/unboxing for primitive values */
private long primitiveValue;
public ColumnValue() {
super();
}
public ColumnValue(ColumnValue other) {
super(other);
this.primitiveValue = other.primitiveValue;
}
public ColumnValue deepCopy() {
return new ColumnValue(this);
}
public static ColumnValue bool_val(boolean value) {
ColumnValue x = new ColumnValue();
x.setBool_val(value);
return x;
}
public static ColumnValue byte_val(byte value) {
ColumnValue x = new ColumnValue();
x.setByte_val(value);
return x;
}
public static ColumnValue i16_val(short value) {
ColumnValue x = new ColumnValue();
x.setI16_val(value);
return x;
}
public static ColumnValue i32_val(int value) {
ColumnValue x = new ColumnValue();
x.setI32_val(value);
return x;
}
public static ColumnValue i64_val(long value) {
ColumnValue x = new ColumnValue();
x.setI64_val(value);
return x;
}
public static ColumnValue float_val(int value) {
ColumnValue x = new ColumnValue();
x.setFloat_val(value);
return x;
}
public static ColumnValue double_val(double value) {
ColumnValue x = new ColumnValue();
x.setDouble_val(value);
return x;
}
public static ColumnValue string_val(String value) {
ColumnValue x = new ColumnValue();
x.setString_val(value);
return x;
}
public static ColumnValue decimal_val(Decimal value) {
ColumnValue x = new ColumnValue();
x.setDecimal_val(value);
return x;
}
public static ColumnValue date_val(long value) {
ColumnValue x = new ColumnValue();
x.setDate_val(value);
return x;
}
public static ColumnValue time_val(long value) {
ColumnValue x = new ColumnValue();
x.setTime_val(value);
return x;
}
public static ColumnValue timestamp_val(long value) {
ColumnValue x = new ColumnValue();
x.setTimestamp_val(value);
return x;
}
public static ColumnValue binary_val(ByteBuffer value) {
ColumnValue x = new ColumnValue();
x.setBinary_val(value);
return x;
}
public static ColumnValue binary_val(byte[] value) {
ColumnValue x = new ColumnValue();
x.setBinary_val(ByteBuffer.wrap(Arrays.copyOf(value, value.length)));
return x;
}
public static ColumnValue blob_val(BlobChunk value) {
ColumnValue x = new ColumnValue();
x.setBlob_val(value);
return x;
}
public static ColumnValue clob_val(ClobChunk value) {
ColumnValue x = new ColumnValue();
x.setClob_val(value);
return x;
}
public static ColumnValue array_val(List value) {
ColumnValue x = new ColumnValue();
x.setArray_val(value);
return x;
}
public static ColumnValue map_val(Map value) {
ColumnValue x = new ColumnValue();
x.setMap_val(value);
return x;
}
public static ColumnValue struct_val(List value) {
ColumnValue x = new ColumnValue();
x.setStruct_val(value);
return x;
}
public static ColumnValue null_val(boolean value) {
ColumnValue x = new ColumnValue();
x.setNull_val(value);
return x;
}
public static ColumnValue java_val(ByteBuffer value) {
ColumnValue x = new ColumnValue();
x.setJava_val(value);
return x;
}
public static ColumnValue java_val(byte[] value) {
ColumnValue x = new ColumnValue();
x.setJava_val(ByteBuffer.wrap(Arrays.copyOf(value, value.length)));
return x;
}
@Override
protected void checkType(_Fields setField, Object value) throws ClassCastException {
switch (setField) {
case BOOL_VAL:
if (value instanceof Boolean) {
break;
}
throw new ClassCastException("Was expecting value of type Boolean for field 'bool_val', but got " + value.getClass().getSimpleName());
case BYTE_VAL:
if (value instanceof Byte) {
break;
}
throw new ClassCastException("Was expecting value of type Byte for field 'byte_val', but got " + value.getClass().getSimpleName());
case I16_VAL:
if (value instanceof Short) {
break;
}
throw new ClassCastException("Was expecting value of type Short for field 'i16_val', but got " + value.getClass().getSimpleName());
case I32_VAL:
if (value instanceof Integer) {
break;
}
throw new ClassCastException("Was expecting value of type Integer for field 'i32_val', but got " + value.getClass().getSimpleName());
case I64_VAL:
if (value instanceof Long) {
break;
}
throw new ClassCastException("Was expecting value of type Long for field 'i64_val', but got " + value.getClass().getSimpleName());
case FLOAT_VAL:
if (value instanceof Integer) {
break;
}
throw new ClassCastException("Was expecting value of type Integer for field 'float_val', but got " + value.getClass().getSimpleName());
case DOUBLE_VAL:
if (value instanceof Double) {
break;
}
throw new ClassCastException("Was expecting value of type Double for field 'double_val', but got " + value.getClass().getSimpleName());
case STRING_VAL:
if (value instanceof String) {
break;
}
throw new ClassCastException("Was expecting value of type String for field 'string_val', but got " + value.getClass().getSimpleName());
case DECIMAL_VAL:
if (value instanceof Decimal) {
break;
}
throw new ClassCastException("Was expecting value of type Decimal for field 'decimal_val', but got " + value.getClass().getSimpleName());
case DATE_VAL:
if (value instanceof Long) {
break;
}
throw new ClassCastException("Was expecting value of type Long for field 'date_val', but got " + value.getClass().getSimpleName());
case TIME_VAL:
if (value instanceof Long) {
break;
}
throw new ClassCastException("Was expecting value of type Long for field 'time_val', but got " + value.getClass().getSimpleName());
case TIMESTAMP_VAL:
if (value instanceof Long) {
break;
}
throw new ClassCastException("Was expecting value of type Long for field 'timestamp_val', but got " + value.getClass().getSimpleName());
case BINARY_VAL:
if (value instanceof ByteBuffer) {
break;
}
throw new ClassCastException("Was expecting value of type ByteBuffer for field 'binary_val', but got " + value.getClass().getSimpleName());
case BLOB_VAL:
if (value instanceof BlobChunk) {
break;
}
throw new ClassCastException("Was expecting value of type BlobChunk for field 'blob_val', but got " + value.getClass().getSimpleName());
case CLOB_VAL:
if (value instanceof ClobChunk) {
break;
}
throw new ClassCastException("Was expecting value of type ClobChunk for field 'clob_val', but got " + value.getClass().getSimpleName());
case ARRAY_VAL:
if (value instanceof List) {
break;
}
throw new ClassCastException("Was expecting value of type List for field 'array_val', but got " + value.getClass().getSimpleName());
case MAP_VAL:
if (value instanceof Map) {
break;
}
throw new ClassCastException("Was expecting value of type Map for field 'map_val', but got " + value.getClass().getSimpleName());
case STRUCT_VAL:
if (value instanceof List) {
break;
}
throw new ClassCastException("Was expecting value of type List for field 'struct_val', but got " + value.getClass().getSimpleName());
case NULL_VAL:
if (value instanceof Boolean) {
break;
}
throw new ClassCastException("Was expecting value of type Boolean for field 'null_val', but got " + value.getClass().getSimpleName());
case JAVA_VAL:
if (value instanceof ByteBuffer) {
break;
}
throw new ClassCastException("Was expecting value of type ByteBuffer for field 'java_val', but got " + value.getClass().getSimpleName());
default:
throw new IllegalArgumentException("Unknown field id " + setField);
}
}
@Override
protected Object standardSchemeReadValue(org.apache.thrift.protocol.TProtocol iprot, org.apache.thrift.protocol.TField field) throws org.apache.thrift.TException {
_Fields setField = _Fields.findByThriftId(field.id);
if (setField != null) {
switch (setField) {
case BOOL_VAL:
if (field.type == BOOL_VAL_FIELD_DESC.type) {
this.primitiveValue = iprot.readBool() ? 1L : 0L;
setField_ = _Fields.BOOL_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case BYTE_VAL:
if (field.type == BYTE_VAL_FIELD_DESC.type) {
this.primitiveValue = iprot.readByte();
setField_ = _Fields.BYTE_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case I16_VAL:
if (field.type == I16_VAL_FIELD_DESC.type) {
this.primitiveValue = iprot.readI16();
setField_ = _Fields.I16_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case I32_VAL:
if (field.type == I32_VAL_FIELD_DESC.type) {
this.primitiveValue = iprot.readI32();
setField_ = _Fields.I32_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case I64_VAL:
if (field.type == I64_VAL_FIELD_DESC.type) {
this.primitiveValue = iprot.readI64();
setField_ = _Fields.I64_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case FLOAT_VAL:
if (field.type == FLOAT_VAL_FIELD_DESC.type) {
this.primitiveValue = iprot.readI32();
setField_ = _Fields.FLOAT_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case DOUBLE_VAL:
if (field.type == DOUBLE_VAL_FIELD_DESC.type) {
this.primitiveValue = Double.doubleToLongBits(iprot.readDouble());
setField_ = _Fields.DOUBLE_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case STRING_VAL:
if (field.type == STRING_VAL_FIELD_DESC.type) {
String string_val;
string_val = iprot.readString();
return string_val;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case DECIMAL_VAL:
if (field.type == DECIMAL_VAL_FIELD_DESC.type) {
Decimal decimal_val;
decimal_val = new Decimal();
decimal_val.read(iprot);
return decimal_val;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case DATE_VAL:
if (field.type == DATE_VAL_FIELD_DESC.type) {
this.primitiveValue = iprot.readI64();
setField_ = _Fields.DATE_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case TIME_VAL:
if (field.type == TIME_VAL_FIELD_DESC.type) {
this.primitiveValue = iprot.readI64();
setField_ = _Fields.TIME_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case TIMESTAMP_VAL:
if (field.type == TIMESTAMP_VAL_FIELD_DESC.type) {
this.primitiveValue = iprot.readI64();
setField_ = _Fields.TIMESTAMP_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case BINARY_VAL:
if (field.type == BINARY_VAL_FIELD_DESC.type) {
ByteBuffer binary_val;
binary_val = iprot.readBinary();
return binary_val;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case BLOB_VAL:
if (field.type == BLOB_VAL_FIELD_DESC.type) {
BlobChunk blob_val;
blob_val = new BlobChunk();
blob_val.read(iprot);
return blob_val;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case CLOB_VAL:
if (field.type == CLOB_VAL_FIELD_DESC.type) {
ClobChunk clob_val;
clob_val = new ClobChunk();
clob_val.read(iprot);
return clob_val;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case ARRAY_VAL:
if (field.type == ARRAY_VAL_FIELD_DESC.type) {
List array_val;
{
org.apache.thrift.protocol.TList _list146 = iprot.readListBegin();
array_val = new ArrayList<>(_list146.size);
ColumnValue _elem147;
for (int _i148 = 0; _i148 < _list146.size; ++_i148)
{
_elem147 = new ColumnValue();
_elem147.read(iprot);
array_val.add(_elem147);
}
iprot.readListEnd();
}
return array_val;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case MAP_VAL:
if (field.type == MAP_VAL_FIELD_DESC.type) {
Map map_val;
{
org.apache.thrift.protocol.TMap _map149 = iprot.readMapBegin();
map_val = new HashMap<>(2 * _map149.size);
ColumnValue _key150;
ColumnValue _val151;
for (int _i152 = 0; _i152 < _map149.size; ++_i152)
{
_key150 = new ColumnValue();
_key150.read(iprot);
_val151 = new ColumnValue();
_val151.read(iprot);
map_val.put(_key150, _val151);
}
iprot.readMapEnd();
}
return map_val;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case STRUCT_VAL:
if (field.type == STRUCT_VAL_FIELD_DESC.type) {
List struct_val;
{
org.apache.thrift.protocol.TList _list153 = iprot.readListBegin();
struct_val = new ArrayList<>(_list153.size);
ColumnValue _elem154;
for (int _i155 = 0; _i155 < _list153.size; ++_i155)
{
_elem154 = new ColumnValue();
_elem154.read(iprot);
struct_val.add(_elem154);
}
iprot.readListEnd();
}
return struct_val;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case NULL_VAL:
if (field.type == NULL_VAL_FIELD_DESC.type) {
this.primitiveValue = iprot.readBool() ? 1L : 0L;
setField_ = _Fields.NULL_VAL;
return null;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
case JAVA_VAL:
if (field.type == JAVA_VAL_FIELD_DESC.type) {
ByteBuffer java_val;
java_val = iprot.readBinary();
return java_val;
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
default:
throw new IllegalStateException("setField wasn't null, but didn't match any of the case statements!");
}
} else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot, field.type);
return null;
}
}
@Override
public void write(TProtocol oprot) throws TException {
if (getSetField() == null || getFieldValue() == null) {
throw new TProtocolException("Cannot write a TUnion with no set value!");
}
oprot.writeStructBegin(getStructDesc());
oprot.writeFieldBegin(getFieldDesc(setField_));
standardSchemeWriteValue(oprot);
oprot.writeFieldEnd();
oprot.writeFieldStop();
oprot.writeStructEnd();
}
@Override
protected void standardSchemeWriteValue(org.apache.thrift.protocol.TProtocol oprot)
throws org.apache.thrift.TException {
switch (setField_) {
case BOOL_VAL:
boolean bool_val = this.primitiveValue != 0L;
oprot.writeBool(bool_val);
return;
case BYTE_VAL:
byte byte_val = (byte)this.primitiveValue;
oprot.writeByte(byte_val);
return;
case I16_VAL:
short i16_val = (short)this.primitiveValue;
oprot.writeI16(i16_val);
return;
case I32_VAL:
int i32_val = (int)this.primitiveValue;
oprot.writeI32(i32_val);
return;
case I64_VAL:
long i64_val = this.primitiveValue;
oprot.writeI64(i64_val);
return;
case FLOAT_VAL:
int float_val = (int)this.primitiveValue;
oprot.writeI32(float_val);
return;
case DOUBLE_VAL:
double double_val = Double.longBitsToDouble(this.primitiveValue);
oprot.writeDouble(double_val);
return;
case STRING_VAL:
String string_val = (String)value_;
oprot.writeString(string_val);
return;
case DECIMAL_VAL:
Decimal decimal_val = (Decimal)value_;
decimal_val.write(oprot);
return;
case DATE_VAL:
long date_val = this.primitiveValue;
oprot.writeI64(date_val);
return;
case TIME_VAL:
long time_val = this.primitiveValue;
oprot.writeI64(time_val);
return;
case TIMESTAMP_VAL:
long timestamp_val = this.primitiveValue;
oprot.writeI64(timestamp_val);
return;
case BINARY_VAL:
ByteBuffer binary_val = (ByteBuffer)value_;
oprot.writeBinary(binary_val);
return;
case BLOB_VAL:
BlobChunk blob_val = (BlobChunk)value_;
blob_val.write(oprot);
return;
case CLOB_VAL:
ClobChunk clob_val = (ClobChunk)value_;
clob_val.write(oprot);
return;
case ARRAY_VAL:
List array_val = (List)value_;
{
oprot.writeListBegin(new org.apache.thrift.protocol.TList(
org.apache.thrift.protocol.TType.STRUCT, array_val.size()));
for (ColumnValue _iter156 : array_val)
{
_iter156.write(oprot);
}
oprot.writeListEnd();
}
return;
case MAP_VAL:
Map map_val = (Map)value_;
{
oprot.writeMapBegin(new org.apache.thrift.protocol.TMap(
org.apache.thrift.protocol.TType.STRUCT,
org.apache.thrift.protocol.TType.STRUCT, map_val.size()));
for (Map.Entry _iter157 : map_val.entrySet())
{
_iter157.getKey().write(oprot);
_iter157.getValue().write(oprot);
}
oprot.writeMapEnd();
}
return;
case STRUCT_VAL:
List struct_val = (List)value_;
{
oprot.writeListBegin(new org.apache.thrift.protocol.TList(
org.apache.thrift.protocol.TType.STRUCT, struct_val.size()));
for (ColumnValue _iter158 : struct_val)
{
_iter158.write(oprot);
}
oprot.writeListEnd();
}
return;
case NULL_VAL:
boolean null_val = this.primitiveValue != 0L;
oprot.writeBool(null_val);
return;
case JAVA_VAL:
ByteBuffer java_val = (ByteBuffer)value_;
oprot.writeBinary(java_val);
return;
default:
throw new IllegalStateException("Cannot write union with unknown field " + setField_);
}
}
@Override
protected Object tupleSchemeReadValue(org.apache.thrift.protocol.TProtocol iprot,
short fieldID) throws org.apache.thrift.TException {
throw new TProtocolException("Tuple scheme not supported");
}
@Override
protected void tupleSchemeWriteValue(org.apache.thrift.protocol.TProtocol oprot)
throws org.apache.thrift.TException {
throw new TProtocolException("Tuple scheme not supported");
}
@Override
protected org.apache.thrift.protocol.TField getFieldDesc(_Fields setField) {
switch (setField) {
case BOOL_VAL:
return BOOL_VAL_FIELD_DESC;
case BYTE_VAL:
return BYTE_VAL_FIELD_DESC;
case I16_VAL:
return I16_VAL_FIELD_DESC;
case I32_VAL:
return I32_VAL_FIELD_DESC;
case I64_VAL:
return I64_VAL_FIELD_DESC;
case FLOAT_VAL:
return FLOAT_VAL_FIELD_DESC;
case DOUBLE_VAL:
return DOUBLE_VAL_FIELD_DESC;
case STRING_VAL:
return STRING_VAL_FIELD_DESC;
case DECIMAL_VAL:
return DECIMAL_VAL_FIELD_DESC;
case DATE_VAL:
return DATE_VAL_FIELD_DESC;
case TIME_VAL:
return TIME_VAL_FIELD_DESC;
case TIMESTAMP_VAL:
return TIMESTAMP_VAL_FIELD_DESC;
case BINARY_VAL:
return BINARY_VAL_FIELD_DESC;
case BLOB_VAL:
return BLOB_VAL_FIELD_DESC;
case CLOB_VAL:
return CLOB_VAL_FIELD_DESC;
case ARRAY_VAL:
return ARRAY_VAL_FIELD_DESC;
case MAP_VAL:
return MAP_VAL_FIELD_DESC;
case STRUCT_VAL:
return STRUCT_VAL_FIELD_DESC;
case NULL_VAL:
return NULL_VAL_FIELD_DESC;
case JAVA_VAL:
return JAVA_VAL_FIELD_DESC;
default:
throw new IllegalArgumentException("Unknown field id " + setField);
}
}
@Override
protected org.apache.thrift.protocol.TStruct getStructDesc() {
return STRUCT_DESC;
}
@Override
protected _Fields enumForId(short id) {
return _Fields.findByThriftIdOrThrow(id);
}
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public final long getPrimitiveLong() {
return primitiveValue;
}
public boolean getBool_val() {
if (getSetField() == _Fields.BOOL_VAL) {
return primitiveValue != 0L;
} else {
throw new RuntimeException("Cannot get field 'bool_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setBool_val(boolean value) {
setField_ = _Fields.BOOL_VAL;
primitiveValue = value ? 1L : 0L;
value_ = Boolean.TRUE; // dummy value
}
public byte getByte_val() {
if (getSetField() == _Fields.BYTE_VAL) {
return (byte)primitiveValue;
} else {
throw new RuntimeException("Cannot get field 'byte_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setByte_val(byte value) {
setField_ = _Fields.BYTE_VAL;
primitiveValue = value;
value_ = Boolean.TRUE; // dummy value
}
public short getI16_val() {
if (getSetField() == _Fields.I16_VAL) {
return (short)primitiveValue;
} else {
throw new RuntimeException("Cannot get field 'i16_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setI16_val(short value) {
setField_ = _Fields.I16_VAL;
primitiveValue = value;
value_ = Boolean.TRUE; // dummy value
}
public int getI32_val() {
if (getSetField() == _Fields.I32_VAL) {
return (int)primitiveValue;
} else {
throw new RuntimeException("Cannot get field 'i32_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setI32_val(int value) {
setField_ = _Fields.I32_VAL;
primitiveValue = value;
value_ = Boolean.TRUE; // dummy value
}
public long getI64_val() {
if (getSetField() == _Fields.I64_VAL) {
return primitiveValue;
} else {
throw new RuntimeException("Cannot get field 'i64_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setI64_val(long value) {
setField_ = _Fields.I64_VAL;
primitiveValue = value;
value_ = Boolean.TRUE; // dummy value
}
public int getFloat_val() {
if (getSetField() == _Fields.FLOAT_VAL) {
return (int)primitiveValue;
} else {
throw new RuntimeException("Cannot get field 'float_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setFloat_val(int value) {
setField_ = _Fields.FLOAT_VAL;
primitiveValue = value;
value_ = Boolean.TRUE; // dummy value
}
public double getDouble_val() {
if (getSetField() == _Fields.DOUBLE_VAL) {
return Double.longBitsToDouble(primitiveValue);
} else {
throw new RuntimeException("Cannot get field 'double_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setDouble_val(double value) {
setField_ = _Fields.DOUBLE_VAL;
primitiveValue = Double.doubleToLongBits(value);
value_ = Boolean.TRUE; // dummy value
}
public String getString_val() {
if (getSetField() == _Fields.STRING_VAL) {
return (String)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'string_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setString_val(String value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.STRING_VAL;
value_ = value;
primitiveValue = 0L; // dummy value
}
public Decimal getDecimal_val() {
if (getSetField() == _Fields.DECIMAL_VAL) {
return (Decimal)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'decimal_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setDecimal_val(Decimal value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.DECIMAL_VAL;
value_ = value;
primitiveValue = 0L; // dummy value
}
public long getDate_val() {
if (getSetField() == _Fields.DATE_VAL) {
return primitiveValue;
} else {
throw new RuntimeException("Cannot get field 'date_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setDate_val(long value) {
setField_ = _Fields.DATE_VAL;
primitiveValue = value;
value_ = Boolean.TRUE; // dummy value
}
public long getTime_val() {
if (getSetField() == _Fields.TIME_VAL) {
return primitiveValue;
} else {
throw new RuntimeException("Cannot get field 'time_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setTime_val(long value) {
setField_ = _Fields.TIME_VAL;
primitiveValue = value;
value_ = Boolean.TRUE; // dummy value
}
public long getTimestamp_val() {
if (getSetField() == _Fields.TIMESTAMP_VAL) {
return primitiveValue;
} else {
throw new RuntimeException("Cannot get field 'timestamp_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setTimestamp_val(long value) {
setField_ = _Fields.TIMESTAMP_VAL;
primitiveValue = value;
value_ = Boolean.TRUE; // dummy value
}
public byte[] getBinary_val() {
if (getSetField() == _Fields.BINARY_VAL) {
ByteBuffer buffer = (ByteBuffer)getFieldValue();
if (buffer == null) {
return null;
}
if (!TBaseHelper.wrapsFullArray(buffer)) {
// replace with byte array wrapper
buffer = ByteBuffer.wrap(ThriftUtils.toBytes(buffer));
setBinary_val(buffer);
}
return buffer.array();
} else {
throw new RuntimeException(
"Cannot get field 'binary_val' because union is currently set to " +
getFieldDesc(getSetField()).name);
}
}
public void setBinary_val(byte[] value) {
setBinary_val(ByteBuffer.wrap(Arrays.copyOf(value, value.length)));
}
public void setBinary_val(ByteBuffer value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.BINARY_VAL;
value_ = value;
primitiveValue = 0L; // dummy value
}
public BlobChunk getBlob_val() {
if (getSetField() == _Fields.BLOB_VAL) {
return (BlobChunk)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'blob_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setBlob_val(BlobChunk value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.BLOB_VAL;
value_ = value;
primitiveValue = 0L; // dummy value
}
public ClobChunk getClob_val() {
if (getSetField() == _Fields.CLOB_VAL) {
return (ClobChunk)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'clob_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setClob_val(ClobChunk value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.CLOB_VAL;
value_ = value;
primitiveValue = 0L; // dummy value
}
public List getArray_val() {
if (getSetField() == _Fields.ARRAY_VAL) {
return (List)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'array_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setArray_val(List value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.ARRAY_VAL;
value_ = value;
primitiveValue = 0L; // dummy value
}
public Map getMap_val() {
if (getSetField() == _Fields.MAP_VAL) {
return (Map)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'map_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setMap_val(Map value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.MAP_VAL;
value_ = value;
primitiveValue = 0L; // dummy value
}
public List getStruct_val() {
if (getSetField() == _Fields.STRUCT_VAL) {
return (List)getFieldValue();
} else {
throw new RuntimeException("Cannot get field 'struct_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setStruct_val(List value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.STRUCT_VAL;
value_ = value;
primitiveValue = 0L; // dummy value
}
public boolean getNull_val() {
if (getSetField() == _Fields.NULL_VAL) {
return primitiveValue != 0L;
} else {
throw new RuntimeException("Cannot get field 'null_val' because union is currently set to " + getFieldDesc(getSetField()).name);
}
}
public void setNull_val(boolean value) {
setField_ = _Fields.NULL_VAL;
primitiveValue = value ? 1L : 0L;
value_ = Boolean.TRUE; // dummy value
}
public byte[] getJava_val() {
if (getSetField() == _Fields.JAVA_VAL) {
ByteBuffer buffer = (ByteBuffer)getFieldValue();
if (buffer == null) {
return null;
}
if (!TBaseHelper.wrapsFullArray(buffer)) {
// replace with byte array wrapper
buffer = ByteBuffer.wrap(ThriftUtils.toBytes(buffer));
setJava_val(buffer);
}
return buffer.array();
} else {
throw new RuntimeException(
"Cannot get field 'java_val' because union is currently set to " +
getFieldDesc(getSetField()).name);
}
}
public void setJava_val(byte[] value) {
setJava_val(ByteBuffer.wrap(Arrays.copyOf(value, value.length)));
}
public void setJava_val(ByteBuffer value) {
if (value == null) throw new NullPointerException();
setField_ = _Fields.JAVA_VAL;
value_ = value;
primitiveValue = 0L; // dummy value
}
public boolean isSetBool_val() {
return setField_ == _Fields.BOOL_VAL;
}
public boolean isSetByte_val() {
return setField_ == _Fields.BYTE_VAL;
}
public boolean isSetI16_val() {
return setField_ == _Fields.I16_VAL;
}
public boolean isSetI32_val() {
return setField_ == _Fields.I32_VAL;
}
public boolean isSetI64_val() {
return setField_ == _Fields.I64_VAL;
}
public boolean isSetFloat_val() {
return setField_ == _Fields.FLOAT_VAL;
}
public boolean isSetDouble_val() {
return setField_ == _Fields.DOUBLE_VAL;
}
public boolean isSetString_val() {
return setField_ == _Fields.STRING_VAL;
}
public boolean isSetDecimal_val() {
return setField_ == _Fields.DECIMAL_VAL;
}
public boolean isSetDate_val() {
return setField_ == _Fields.DATE_VAL;
}
public boolean isSetTime_val() {
return setField_ == _Fields.TIME_VAL;
}
public boolean isSetTimestamp_val() {
return setField_ == _Fields.TIMESTAMP_VAL;
}
public boolean isSetBinary_val() {
return setField_ == _Fields.BINARY_VAL;
}
public boolean isSetBlob_val() {
return setField_ == _Fields.BLOB_VAL;
}
public boolean isSetClob_val() {
return setField_ == _Fields.CLOB_VAL;
}
public boolean isSetArray_val() {
return setField_ == _Fields.ARRAY_VAL;
}
public boolean isSetMap_val() {
return setField_ == _Fields.MAP_VAL;
}
public boolean isSetStruct_val() {
return setField_ == _Fields.STRUCT_VAL;
}
public boolean isSetNull_val() {
return setField_ == _Fields.NULL_VAL;
}
public boolean isSetJava_val() {
return setField_ == _Fields.JAVA_VAL;
}
public boolean equals(Object other) {
return other instanceof ColumnValue && equals((ColumnValue)other);
}
public boolean equals(ColumnValue other) {
return other != null && getSetField() == other.getSetField() &&
primitiveValue == other.primitiveValue &&
getFieldValue().equals(other.getFieldValue());
}
@Override
public int compareTo(@SuppressWarnings("NullableProblems") ColumnValue other) {
int lastComparison = org.apache.thrift.TBaseHelper.compareTo(getSetField(), other.getSetField());
if (lastComparison == 0) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(primitiveValue, other.primitiveValue);
}
if (lastComparison == 0) {
return org.apache.thrift.TBaseHelper.compareTo(getFieldValue(), other.getFieldValue());
}
return lastComparison;
}
@Override
public int hashCode() {
int hash = 1;
hash = 31 * hash + this.getClass().getName().hashCode();
org.apache.thrift.TFieldIdEnum setField = getSetField();
if (setField != null) {
hash = 31 * hash + setField.getThriftFieldId();
Object value = getFieldValue();
if (value == null) {
hash = 31 * hash + (int)(primitiveValue ^ (primitiveValue >>> 32));
} else if (value instanceof org.apache.thrift.TEnum) {
hash = 31 * hash + ((org.apache.thrift.TEnum)getFieldValue()).getValue();
} else {
hash = 31 * hash + value.hashCode();
}
}
return hash;
}
private void writeObject(java.io.ObjectOutputStream out) throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(out)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in) throws java.io.IOException, ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(new org.apache.thrift.transport.TIOStreamTransport(in)));
} catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy