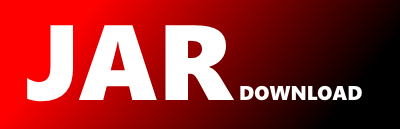
io.snappydata.thrift.RowSet Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package io.snappydata.thrift;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-08-09")
public class RowSet implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("RowSet");
private static final org.apache.thrift.protocol.TField ROWS_FIELD_DESC = new org.apache.thrift.protocol.TField("rows", org.apache.thrift.protocol.TType.LIST, (short)1);
private static final org.apache.thrift.protocol.TField FLAGS_FIELD_DESC = new org.apache.thrift.protocol.TField("flags", org.apache.thrift.protocol.TType.BYTE, (short)2);
private static final org.apache.thrift.protocol.TField CURSOR_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("cursorId", org.apache.thrift.protocol.TType.I64, (short)3);
private static final org.apache.thrift.protocol.TField STATEMENT_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("statementId", org.apache.thrift.protocol.TType.I64, (short)4);
private static final org.apache.thrift.protocol.TField CONN_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("connId", org.apache.thrift.protocol.TType.I64, (short)5);
private static final org.apache.thrift.protocol.TField TOKEN_FIELD_DESC = new org.apache.thrift.protocol.TField("token", org.apache.thrift.protocol.TType.STRING, (short)6);
private static final org.apache.thrift.protocol.TField SOURCE_FIELD_DESC = new org.apache.thrift.protocol.TField("source", org.apache.thrift.protocol.TType.STRUCT, (short)7);
private static final org.apache.thrift.protocol.TField OFFSET_FIELD_DESC = new org.apache.thrift.protocol.TField("offset", org.apache.thrift.protocol.TType.I32, (short)8);
private static final org.apache.thrift.protocol.TField METADATA_FIELD_DESC = new org.apache.thrift.protocol.TField("metadata", org.apache.thrift.protocol.TType.LIST, (short)9);
private static final org.apache.thrift.protocol.TField CURSOR_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("cursorName", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField WARNINGS_FIELD_DESC = new org.apache.thrift.protocol.TField("warnings", org.apache.thrift.protocol.TType.STRUCT, (short)11);
private static final org.apache.thrift.protocol.TField ROW_IDS_FOR_UPDATE_OR_DELETE_FIELD_DESC = new org.apache.thrift.protocol.TField("rowIdsForUpdateOrDelete", org.apache.thrift.protocol.TType.LIST, (short)12);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new RowSetStandardSchemeFactory());
schemes.put(TupleScheme.class, new RowSetTupleSchemeFactory());
}
public List rows; // required
public byte flags; // required
public long cursorId; // required
public long statementId; // required
public long connId; // required
public ByteBuffer token; // optional
public HostAddress source; // optional
public int offset; // optional
public List metadata; // optional
public String cursorName; // optional
public SnappyExceptionData warnings; // optional
public List rowIdsForUpdateOrDelete; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
ROWS((short)1, "rows"),
FLAGS((short)2, "flags"),
CURSOR_ID((short)3, "cursorId"),
STATEMENT_ID((short)4, "statementId"),
CONN_ID((short)5, "connId"),
TOKEN((short)6, "token"),
SOURCE((short)7, "source"),
OFFSET((short)8, "offset"),
METADATA((short)9, "metadata"),
CURSOR_NAME((short)10, "cursorName"),
WARNINGS((short)11, "warnings"),
ROW_IDS_FOR_UPDATE_OR_DELETE((short)12, "rowIdsForUpdateOrDelete");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // ROWS
return ROWS;
case 2: // FLAGS
return FLAGS;
case 3: // CURSOR_ID
return CURSOR_ID;
case 4: // STATEMENT_ID
return STATEMENT_ID;
case 5: // CONN_ID
return CONN_ID;
case 6: // TOKEN
return TOKEN;
case 7: // SOURCE
return SOURCE;
case 8: // OFFSET
return OFFSET;
case 9: // METADATA
return METADATA;
case 10: // CURSOR_NAME
return CURSOR_NAME;
case 11: // WARNINGS
return WARNINGS;
case 12: // ROW_IDS_FOR_UPDATE_OR_DELETE
return ROW_IDS_FOR_UPDATE_OR_DELETE;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __FLAGS_ISSET_ID = 0;
private static final int __CURSORID_ISSET_ID = 1;
private static final int __STATEMENTID_ISSET_ID = 2;
private static final int __CONNID_ISSET_ID = 3;
private static final int __OFFSET_ISSET_ID = 4;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.TOKEN,_Fields.SOURCE,_Fields.OFFSET,_Fields.METADATA,_Fields.CURSOR_NAME,_Fields.WARNINGS,_Fields.ROW_IDS_FOR_UPDATE_OR_DELETE};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.ROWS, new org.apache.thrift.meta_data.FieldMetaData("rows", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Row.class))));
tmpMap.put(_Fields.FLAGS, new org.apache.thrift.meta_data.FieldMetaData("flags", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BYTE)));
tmpMap.put(_Fields.CURSOR_ID, new org.apache.thrift.meta_data.FieldMetaData("cursorId", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.STATEMENT_ID, new org.apache.thrift.meta_data.FieldMetaData("statementId", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.CONN_ID, new org.apache.thrift.meta_data.FieldMetaData("connId", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.TOKEN, new org.apache.thrift.meta_data.FieldMetaData("token", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.SOURCE, new org.apache.thrift.meta_data.FieldMetaData("source", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, HostAddress.class)));
tmpMap.put(_Fields.OFFSET, new org.apache.thrift.meta_data.FieldMetaData("offset", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.METADATA, new org.apache.thrift.meta_data.FieldMetaData("metadata", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, ColumnDescriptor.class))));
tmpMap.put(_Fields.CURSOR_NAME, new org.apache.thrift.meta_data.FieldMetaData("cursorName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.WARNINGS, new org.apache.thrift.meta_data.FieldMetaData("warnings", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, SnappyExceptionData.class)));
tmpMap.put(_Fields.ROW_IDS_FOR_UPDATE_OR_DELETE, new org.apache.thrift.meta_data.FieldMetaData("rowIdsForUpdateOrDelete", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64))));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(RowSet.class, metaDataMap);
}
public RowSet() {
}
public RowSet(
List rows,
byte flags,
long cursorId,
long statementId,
long connId)
{
this();
this.rows = rows;
this.flags = flags;
setFlagsIsSet(true);
this.cursorId = cursorId;
setCursorIdIsSet(true);
this.statementId = statementId;
setStatementIdIsSet(true);
this.connId = connId;
setConnIdIsSet(true);
}
/**
* Performs a deep copy on other.
*/
public RowSet(RowSet other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetRows()) {
List __this__rows = new ArrayList(other.rows.size());
for (Row other_element : other.rows) {
__this__rows.add(new Row(other_element));
}
this.rows = __this__rows;
}
this.flags = other.flags;
this.cursorId = other.cursorId;
this.statementId = other.statementId;
this.connId = other.connId;
if (other.isSetToken()) {
this.token = org.apache.thrift.TBaseHelper.copyBinary(other.token);
}
if (other.isSetSource()) {
this.source = new HostAddress(other.source);
}
this.offset = other.offset;
if (other.isSetMetadata()) {
List __this__metadata = new ArrayList(other.metadata.size());
for (ColumnDescriptor other_element : other.metadata) {
__this__metadata.add(new ColumnDescriptor(other_element));
}
this.metadata = __this__metadata;
}
if (other.isSetCursorName()) {
this.cursorName = other.cursorName;
}
if (other.isSetWarnings()) {
this.warnings = new SnappyExceptionData(other.warnings);
}
if (other.isSetRowIdsForUpdateOrDelete()) {
List __this__rowIdsForUpdateOrDelete = new ArrayList(other.rowIdsForUpdateOrDelete);
this.rowIdsForUpdateOrDelete = __this__rowIdsForUpdateOrDelete;
}
}
public RowSet deepCopy() {
return new RowSet(this);
}
@Override
public void clear() {
this.rows = null;
setFlagsIsSet(false);
this.flags = 0;
setCursorIdIsSet(false);
this.cursorId = 0;
setStatementIdIsSet(false);
this.statementId = 0;
setConnIdIsSet(false);
this.connId = 0;
this.token = null;
this.source = null;
setOffsetIsSet(false);
this.offset = 0;
this.metadata = null;
this.cursorName = null;
this.warnings = null;
this.rowIdsForUpdateOrDelete = null;
}
public int getRowsSize() {
return (this.rows == null) ? 0 : this.rows.size();
}
public java.util.Iterator getRowsIterator() {
return (this.rows == null) ? null : this.rows.iterator();
}
public void addToRows(Row elem) {
if (this.rows == null) {
this.rows = new ArrayList();
}
this.rows.add(elem);
}
public List getRows() {
return this.rows;
}
public RowSet setRows(List rows) {
this.rows = rows;
return this;
}
public void unsetRows() {
this.rows = null;
}
/** Returns true if field rows is set (has been assigned a value) and false otherwise */
public boolean isSetRows() {
return this.rows != null;
}
public void setRowsIsSet(boolean value) {
if (!value) {
this.rows = null;
}
}
public byte getFlags() {
return this.flags;
}
public RowSet setFlags(byte flags) {
this.flags = flags;
setFlagsIsSet(true);
return this;
}
public void unsetFlags() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __FLAGS_ISSET_ID);
}
/** Returns true if field flags is set (has been assigned a value) and false otherwise */
public boolean isSetFlags() {
return EncodingUtils.testBit(__isset_bitfield, __FLAGS_ISSET_ID);
}
public void setFlagsIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __FLAGS_ISSET_ID, value);
}
public long getCursorId() {
return this.cursorId;
}
public RowSet setCursorId(long cursorId) {
this.cursorId = cursorId;
setCursorIdIsSet(true);
return this;
}
public void unsetCursorId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __CURSORID_ISSET_ID);
}
/** Returns true if field cursorId is set (has been assigned a value) and false otherwise */
public boolean isSetCursorId() {
return EncodingUtils.testBit(__isset_bitfield, __CURSORID_ISSET_ID);
}
public void setCursorIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __CURSORID_ISSET_ID, value);
}
public long getStatementId() {
return this.statementId;
}
public RowSet setStatementId(long statementId) {
this.statementId = statementId;
setStatementIdIsSet(true);
return this;
}
public void unsetStatementId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __STATEMENTID_ISSET_ID);
}
/** Returns true if field statementId is set (has been assigned a value) and false otherwise */
public boolean isSetStatementId() {
return EncodingUtils.testBit(__isset_bitfield, __STATEMENTID_ISSET_ID);
}
public void setStatementIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __STATEMENTID_ISSET_ID, value);
}
public long getConnId() {
return this.connId;
}
public RowSet setConnId(long connId) {
this.connId = connId;
setConnIdIsSet(true);
return this;
}
public void unsetConnId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __CONNID_ISSET_ID);
}
/** Returns true if field connId is set (has been assigned a value) and false otherwise */
public boolean isSetConnId() {
return EncodingUtils.testBit(__isset_bitfield, __CONNID_ISSET_ID);
}
public void setConnIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __CONNID_ISSET_ID, value);
}
public byte[] getToken() {
setToken(org.apache.thrift.TBaseHelper.rightSize(token));
return token == null ? null : token.array();
}
public ByteBuffer bufferForToken() {
return org.apache.thrift.TBaseHelper.copyBinary(token);
}
public RowSet setToken(byte[] token) {
this.token = token == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(token, token.length));
return this;
}
public RowSet setToken(ByteBuffer token) {
this.token = org.apache.thrift.TBaseHelper.copyBinary(token);
return this;
}
public void unsetToken() {
this.token = null;
}
/** Returns true if field token is set (has been assigned a value) and false otherwise */
public boolean isSetToken() {
return this.token != null;
}
public void setTokenIsSet(boolean value) {
if (!value) {
this.token = null;
}
}
public HostAddress getSource() {
return this.source;
}
public RowSet setSource(HostAddress source) {
this.source = source;
return this;
}
public void unsetSource() {
this.source = null;
}
/** Returns true if field source is set (has been assigned a value) and false otherwise */
public boolean isSetSource() {
return this.source != null;
}
public void setSourceIsSet(boolean value) {
if (!value) {
this.source = null;
}
}
public int getOffset() {
return this.offset;
}
public RowSet setOffset(int offset) {
this.offset = offset;
setOffsetIsSet(true);
return this;
}
public void unsetOffset() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __OFFSET_ISSET_ID);
}
/** Returns true if field offset is set (has been assigned a value) and false otherwise */
public boolean isSetOffset() {
return EncodingUtils.testBit(__isset_bitfield, __OFFSET_ISSET_ID);
}
public void setOffsetIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __OFFSET_ISSET_ID, value);
}
public int getMetadataSize() {
return (this.metadata == null) ? 0 : this.metadata.size();
}
public java.util.Iterator getMetadataIterator() {
return (this.metadata == null) ? null : this.metadata.iterator();
}
public void addToMetadata(ColumnDescriptor elem) {
if (this.metadata == null) {
this.metadata = new ArrayList();
}
this.metadata.add(elem);
}
public List getMetadata() {
return this.metadata;
}
public RowSet setMetadata(List metadata) {
this.metadata = metadata;
return this;
}
public void unsetMetadata() {
this.metadata = null;
}
/** Returns true if field metadata is set (has been assigned a value) and false otherwise */
public boolean isSetMetadata() {
return this.metadata != null;
}
public void setMetadataIsSet(boolean value) {
if (!value) {
this.metadata = null;
}
}
public String getCursorName() {
return this.cursorName;
}
public RowSet setCursorName(String cursorName) {
this.cursorName = cursorName;
return this;
}
public void unsetCursorName() {
this.cursorName = null;
}
/** Returns true if field cursorName is set (has been assigned a value) and false otherwise */
public boolean isSetCursorName() {
return this.cursorName != null;
}
public void setCursorNameIsSet(boolean value) {
if (!value) {
this.cursorName = null;
}
}
public SnappyExceptionData getWarnings() {
return this.warnings;
}
public RowSet setWarnings(SnappyExceptionData warnings) {
this.warnings = warnings;
return this;
}
public void unsetWarnings() {
this.warnings = null;
}
/** Returns true if field warnings is set (has been assigned a value) and false otherwise */
public boolean isSetWarnings() {
return this.warnings != null;
}
public void setWarningsIsSet(boolean value) {
if (!value) {
this.warnings = null;
}
}
public int getRowIdsForUpdateOrDeleteSize() {
return (this.rowIdsForUpdateOrDelete == null) ? 0 : this.rowIdsForUpdateOrDelete.size();
}
public java.util.Iterator getRowIdsForUpdateOrDeleteIterator() {
return (this.rowIdsForUpdateOrDelete == null) ? null : this.rowIdsForUpdateOrDelete.iterator();
}
public void addToRowIdsForUpdateOrDelete(long elem) {
if (this.rowIdsForUpdateOrDelete == null) {
this.rowIdsForUpdateOrDelete = new ArrayList();
}
this.rowIdsForUpdateOrDelete.add(elem);
}
public List getRowIdsForUpdateOrDelete() {
return this.rowIdsForUpdateOrDelete;
}
public RowSet setRowIdsForUpdateOrDelete(List rowIdsForUpdateOrDelete) {
this.rowIdsForUpdateOrDelete = rowIdsForUpdateOrDelete;
return this;
}
public void unsetRowIdsForUpdateOrDelete() {
this.rowIdsForUpdateOrDelete = null;
}
/** Returns true if field rowIdsForUpdateOrDelete is set (has been assigned a value) and false otherwise */
public boolean isSetRowIdsForUpdateOrDelete() {
return this.rowIdsForUpdateOrDelete != null;
}
public void setRowIdsForUpdateOrDeleteIsSet(boolean value) {
if (!value) {
this.rowIdsForUpdateOrDelete = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case ROWS:
if (value == null) {
unsetRows();
} else {
setRows((List)value);
}
break;
case FLAGS:
if (value == null) {
unsetFlags();
} else {
setFlags((Byte)value);
}
break;
case CURSOR_ID:
if (value == null) {
unsetCursorId();
} else {
setCursorId((Long)value);
}
break;
case STATEMENT_ID:
if (value == null) {
unsetStatementId();
} else {
setStatementId((Long)value);
}
break;
case CONN_ID:
if (value == null) {
unsetConnId();
} else {
setConnId((Long)value);
}
break;
case TOKEN:
if (value == null) {
unsetToken();
} else {
setToken((ByteBuffer)value);
}
break;
case SOURCE:
if (value == null) {
unsetSource();
} else {
setSource((HostAddress)value);
}
break;
case OFFSET:
if (value == null) {
unsetOffset();
} else {
setOffset((Integer)value);
}
break;
case METADATA:
if (value == null) {
unsetMetadata();
} else {
setMetadata((List)value);
}
break;
case CURSOR_NAME:
if (value == null) {
unsetCursorName();
} else {
setCursorName((String)value);
}
break;
case WARNINGS:
if (value == null) {
unsetWarnings();
} else {
setWarnings((SnappyExceptionData)value);
}
break;
case ROW_IDS_FOR_UPDATE_OR_DELETE:
if (value == null) {
unsetRowIdsForUpdateOrDelete();
} else {
setRowIdsForUpdateOrDelete((List)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case ROWS:
return getRows();
case FLAGS:
return getFlags();
case CURSOR_ID:
return getCursorId();
case STATEMENT_ID:
return getStatementId();
case CONN_ID:
return getConnId();
case TOKEN:
return getToken();
case SOURCE:
return getSource();
case OFFSET:
return getOffset();
case METADATA:
return getMetadata();
case CURSOR_NAME:
return getCursorName();
case WARNINGS:
return getWarnings();
case ROW_IDS_FOR_UPDATE_OR_DELETE:
return getRowIdsForUpdateOrDelete();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case ROWS:
return isSetRows();
case FLAGS:
return isSetFlags();
case CURSOR_ID:
return isSetCursorId();
case STATEMENT_ID:
return isSetStatementId();
case CONN_ID:
return isSetConnId();
case TOKEN:
return isSetToken();
case SOURCE:
return isSetSource();
case OFFSET:
return isSetOffset();
case METADATA:
return isSetMetadata();
case CURSOR_NAME:
return isSetCursorName();
case WARNINGS:
return isSetWarnings();
case ROW_IDS_FOR_UPDATE_OR_DELETE:
return isSetRowIdsForUpdateOrDelete();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof RowSet)
return this.equals((RowSet)that);
return false;
}
public boolean equals(RowSet that) {
if (that == null)
return false;
boolean this_present_rows = true && this.isSetRows();
boolean that_present_rows = true && that.isSetRows();
if (this_present_rows || that_present_rows) {
if (!(this_present_rows && that_present_rows))
return false;
if (!this.rows.equals(that.rows))
return false;
}
boolean this_present_flags = true;
boolean that_present_flags = true;
if (this_present_flags || that_present_flags) {
if (!(this_present_flags && that_present_flags))
return false;
if (this.flags != that.flags)
return false;
}
boolean this_present_cursorId = true;
boolean that_present_cursorId = true;
if (this_present_cursorId || that_present_cursorId) {
if (!(this_present_cursorId && that_present_cursorId))
return false;
if (this.cursorId != that.cursorId)
return false;
}
boolean this_present_statementId = true;
boolean that_present_statementId = true;
if (this_present_statementId || that_present_statementId) {
if (!(this_present_statementId && that_present_statementId))
return false;
if (this.statementId != that.statementId)
return false;
}
boolean this_present_connId = true;
boolean that_present_connId = true;
if (this_present_connId || that_present_connId) {
if (!(this_present_connId && that_present_connId))
return false;
if (this.connId != that.connId)
return false;
}
boolean this_present_token = true && this.isSetToken();
boolean that_present_token = true && that.isSetToken();
if (this_present_token || that_present_token) {
if (!(this_present_token && that_present_token))
return false;
if (!this.token.equals(that.token))
return false;
}
boolean this_present_source = true && this.isSetSource();
boolean that_present_source = true && that.isSetSource();
if (this_present_source || that_present_source) {
if (!(this_present_source && that_present_source))
return false;
if (!this.source.equals(that.source))
return false;
}
boolean this_present_offset = true && this.isSetOffset();
boolean that_present_offset = true && that.isSetOffset();
if (this_present_offset || that_present_offset) {
if (!(this_present_offset && that_present_offset))
return false;
if (this.offset != that.offset)
return false;
}
boolean this_present_metadata = true && this.isSetMetadata();
boolean that_present_metadata = true && that.isSetMetadata();
if (this_present_metadata || that_present_metadata) {
if (!(this_present_metadata && that_present_metadata))
return false;
if (!this.metadata.equals(that.metadata))
return false;
}
boolean this_present_cursorName = true && this.isSetCursorName();
boolean that_present_cursorName = true && that.isSetCursorName();
if (this_present_cursorName || that_present_cursorName) {
if (!(this_present_cursorName && that_present_cursorName))
return false;
if (!this.cursorName.equals(that.cursorName))
return false;
}
boolean this_present_warnings = true && this.isSetWarnings();
boolean that_present_warnings = true && that.isSetWarnings();
if (this_present_warnings || that_present_warnings) {
if (!(this_present_warnings && that_present_warnings))
return false;
if (!this.warnings.equals(that.warnings))
return false;
}
boolean this_present_rowIdsForUpdateOrDelete = true && this.isSetRowIdsForUpdateOrDelete();
boolean that_present_rowIdsForUpdateOrDelete = true && that.isSetRowIdsForUpdateOrDelete();
if (this_present_rowIdsForUpdateOrDelete || that_present_rowIdsForUpdateOrDelete) {
if (!(this_present_rowIdsForUpdateOrDelete && that_present_rowIdsForUpdateOrDelete))
return false;
if (!this.rowIdsForUpdateOrDelete.equals(that.rowIdsForUpdateOrDelete))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy