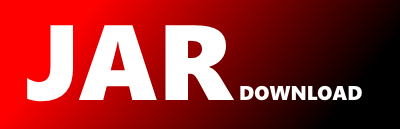
io.snappydata.thrift.ServiceMetaDataArgs Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package io.snappydata.thrift;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-08-09")
public class ServiceMetaDataArgs implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("ServiceMetaDataArgs");
private static final org.apache.thrift.protocol.TField CONN_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("connId", org.apache.thrift.protocol.TType.I64, (short)1);
private static final org.apache.thrift.protocol.TField DRIVER_TYPE_FIELD_DESC = new org.apache.thrift.protocol.TField("driverType", org.apache.thrift.protocol.TType.BYTE, (short)2);
private static final org.apache.thrift.protocol.TField TOKEN_FIELD_DESC = new org.apache.thrift.protocol.TField("token", org.apache.thrift.protocol.TType.STRING, (short)3);
private static final org.apache.thrift.protocol.TField SCHEMA_FIELD_DESC = new org.apache.thrift.protocol.TField("schema", org.apache.thrift.protocol.TType.STRING, (short)4);
private static final org.apache.thrift.protocol.TField TABLE_FIELD_DESC = new org.apache.thrift.protocol.TField("table", org.apache.thrift.protocol.TType.STRING, (short)5);
private static final org.apache.thrift.protocol.TField TABLE_TYPES_FIELD_DESC = new org.apache.thrift.protocol.TField("tableTypes", org.apache.thrift.protocol.TType.LIST, (short)6);
private static final org.apache.thrift.protocol.TField COLUMN_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("columnName", org.apache.thrift.protocol.TType.STRING, (short)7);
private static final org.apache.thrift.protocol.TField FOREIGN_SCHEMA_FIELD_DESC = new org.apache.thrift.protocol.TField("foreignSchema", org.apache.thrift.protocol.TType.STRING, (short)8);
private static final org.apache.thrift.protocol.TField FOREIGN_TABLE_FIELD_DESC = new org.apache.thrift.protocol.TField("foreignTable", org.apache.thrift.protocol.TType.STRING, (short)9);
private static final org.apache.thrift.protocol.TField PROCEDURE_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("procedureName", org.apache.thrift.protocol.TType.STRING, (short)10);
private static final org.apache.thrift.protocol.TField FUNCTION_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("functionName", org.apache.thrift.protocol.TType.STRING, (short)11);
private static final org.apache.thrift.protocol.TField ATTRIBUTE_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("attributeName", org.apache.thrift.protocol.TType.STRING, (short)12);
private static final org.apache.thrift.protocol.TField TYPE_NAME_FIELD_DESC = new org.apache.thrift.protocol.TField("typeName", org.apache.thrift.protocol.TType.STRING, (short)13);
private static final org.apache.thrift.protocol.TField TYPE_ID_FIELD_DESC = new org.apache.thrift.protocol.TField("typeId", org.apache.thrift.protocol.TType.I32, (short)14);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new ServiceMetaDataArgsStandardSchemeFactory());
schemes.put(TupleScheme.class, new ServiceMetaDataArgsTupleSchemeFactory());
}
public long connId; // required
public byte driverType; // required
public ByteBuffer token; // required
public String schema; // optional
public String table; // optional
public List tableTypes; // optional
public String columnName; // optional
public String foreignSchema; // optional
public String foreignTable; // optional
public String procedureName; // optional
public String functionName; // optional
public String attributeName; // optional
public String typeName; // optional
/**
*
* @see SnappyType
*/
public SnappyType typeId; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
CONN_ID((short)1, "connId"),
DRIVER_TYPE((short)2, "driverType"),
TOKEN((short)3, "token"),
SCHEMA((short)4, "schema"),
TABLE((short)5, "table"),
TABLE_TYPES((short)6, "tableTypes"),
COLUMN_NAME((short)7, "columnName"),
FOREIGN_SCHEMA((short)8, "foreignSchema"),
FOREIGN_TABLE((short)9, "foreignTable"),
PROCEDURE_NAME((short)10, "procedureName"),
FUNCTION_NAME((short)11, "functionName"),
ATTRIBUTE_NAME((short)12, "attributeName"),
TYPE_NAME((short)13, "typeName"),
/**
*
* @see SnappyType
*/
TYPE_ID((short)14, "typeId");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // CONN_ID
return CONN_ID;
case 2: // DRIVER_TYPE
return DRIVER_TYPE;
case 3: // TOKEN
return TOKEN;
case 4: // SCHEMA
return SCHEMA;
case 5: // TABLE
return TABLE;
case 6: // TABLE_TYPES
return TABLE_TYPES;
case 7: // COLUMN_NAME
return COLUMN_NAME;
case 8: // FOREIGN_SCHEMA
return FOREIGN_SCHEMA;
case 9: // FOREIGN_TABLE
return FOREIGN_TABLE;
case 10: // PROCEDURE_NAME
return PROCEDURE_NAME;
case 11: // FUNCTION_NAME
return FUNCTION_NAME;
case 12: // ATTRIBUTE_NAME
return ATTRIBUTE_NAME;
case 13: // TYPE_NAME
return TYPE_NAME;
case 14: // TYPE_ID
return TYPE_ID;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __CONNID_ISSET_ID = 0;
private static final int __DRIVERTYPE_ISSET_ID = 1;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.SCHEMA,_Fields.TABLE,_Fields.TABLE_TYPES,_Fields.COLUMN_NAME,_Fields.FOREIGN_SCHEMA,_Fields.FOREIGN_TABLE,_Fields.PROCEDURE_NAME,_Fields.FUNCTION_NAME,_Fields.ATTRIBUTE_NAME,_Fields.TYPE_NAME,_Fields.TYPE_ID};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.CONN_ID, new org.apache.thrift.meta_data.FieldMetaData("connId", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.DRIVER_TYPE, new org.apache.thrift.meta_data.FieldMetaData("driverType", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BYTE)));
tmpMap.put(_Fields.TOKEN, new org.apache.thrift.meta_data.FieldMetaData("token", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.SCHEMA, new org.apache.thrift.meta_data.FieldMetaData("schema", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.TABLE, new org.apache.thrift.meta_data.FieldMetaData("table", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.TABLE_TYPES, new org.apache.thrift.meta_data.FieldMetaData("tableTypes", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
tmpMap.put(_Fields.COLUMN_NAME, new org.apache.thrift.meta_data.FieldMetaData("columnName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.FOREIGN_SCHEMA, new org.apache.thrift.meta_data.FieldMetaData("foreignSchema", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.FOREIGN_TABLE, new org.apache.thrift.meta_data.FieldMetaData("foreignTable", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.PROCEDURE_NAME, new org.apache.thrift.meta_data.FieldMetaData("procedureName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.FUNCTION_NAME, new org.apache.thrift.meta_data.FieldMetaData("functionName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.ATTRIBUTE_NAME, new org.apache.thrift.meta_data.FieldMetaData("attributeName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.TYPE_NAME, new org.apache.thrift.meta_data.FieldMetaData("typeName", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.TYPE_ID, new org.apache.thrift.meta_data.FieldMetaData("typeId", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, SnappyType.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(ServiceMetaDataArgs.class, metaDataMap);
}
public ServiceMetaDataArgs() {
}
public ServiceMetaDataArgs(
long connId,
byte driverType,
ByteBuffer token)
{
this();
this.connId = connId;
setConnIdIsSet(true);
this.driverType = driverType;
setDriverTypeIsSet(true);
this.token = org.apache.thrift.TBaseHelper.copyBinary(token);
}
/**
* Performs a deep copy on other.
*/
public ServiceMetaDataArgs(ServiceMetaDataArgs other) {
__isset_bitfield = other.__isset_bitfield;
this.connId = other.connId;
this.driverType = other.driverType;
if (other.isSetToken()) {
this.token = org.apache.thrift.TBaseHelper.copyBinary(other.token);
}
if (other.isSetSchema()) {
this.schema = other.schema;
}
if (other.isSetTable()) {
this.table = other.table;
}
if (other.isSetTableTypes()) {
List __this__tableTypes = new ArrayList(other.tableTypes);
this.tableTypes = __this__tableTypes;
}
if (other.isSetColumnName()) {
this.columnName = other.columnName;
}
if (other.isSetForeignSchema()) {
this.foreignSchema = other.foreignSchema;
}
if (other.isSetForeignTable()) {
this.foreignTable = other.foreignTable;
}
if (other.isSetProcedureName()) {
this.procedureName = other.procedureName;
}
if (other.isSetFunctionName()) {
this.functionName = other.functionName;
}
if (other.isSetAttributeName()) {
this.attributeName = other.attributeName;
}
if (other.isSetTypeName()) {
this.typeName = other.typeName;
}
if (other.isSetTypeId()) {
this.typeId = other.typeId;
}
}
public ServiceMetaDataArgs deepCopy() {
return new ServiceMetaDataArgs(this);
}
@Override
public void clear() {
setConnIdIsSet(false);
this.connId = 0;
setDriverTypeIsSet(false);
this.driverType = 0;
this.token = null;
this.schema = null;
this.table = null;
this.tableTypes = null;
this.columnName = null;
this.foreignSchema = null;
this.foreignTable = null;
this.procedureName = null;
this.functionName = null;
this.attributeName = null;
this.typeName = null;
this.typeId = null;
}
public long getConnId() {
return this.connId;
}
public ServiceMetaDataArgs setConnId(long connId) {
this.connId = connId;
setConnIdIsSet(true);
return this;
}
public void unsetConnId() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __CONNID_ISSET_ID);
}
/** Returns true if field connId is set (has been assigned a value) and false otherwise */
public boolean isSetConnId() {
return EncodingUtils.testBit(__isset_bitfield, __CONNID_ISSET_ID);
}
public void setConnIdIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __CONNID_ISSET_ID, value);
}
public byte getDriverType() {
return this.driverType;
}
public ServiceMetaDataArgs setDriverType(byte driverType) {
this.driverType = driverType;
setDriverTypeIsSet(true);
return this;
}
public void unsetDriverType() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __DRIVERTYPE_ISSET_ID);
}
/** Returns true if field driverType is set (has been assigned a value) and false otherwise */
public boolean isSetDriverType() {
return EncodingUtils.testBit(__isset_bitfield, __DRIVERTYPE_ISSET_ID);
}
public void setDriverTypeIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __DRIVERTYPE_ISSET_ID, value);
}
public byte[] getToken() {
setToken(org.apache.thrift.TBaseHelper.rightSize(token));
return token == null ? null : token.array();
}
public ByteBuffer bufferForToken() {
return org.apache.thrift.TBaseHelper.copyBinary(token);
}
public ServiceMetaDataArgs setToken(byte[] token) {
this.token = token == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(token, token.length));
return this;
}
public ServiceMetaDataArgs setToken(ByteBuffer token) {
this.token = org.apache.thrift.TBaseHelper.copyBinary(token);
return this;
}
public void unsetToken() {
this.token = null;
}
/** Returns true if field token is set (has been assigned a value) and false otherwise */
public boolean isSetToken() {
return this.token != null;
}
public void setTokenIsSet(boolean value) {
if (!value) {
this.token = null;
}
}
public String getSchema() {
return this.schema;
}
public ServiceMetaDataArgs setSchema(String schema) {
this.schema = schema;
return this;
}
public void unsetSchema() {
this.schema = null;
}
/** Returns true if field schema is set (has been assigned a value) and false otherwise */
public boolean isSetSchema() {
return this.schema != null;
}
public void setSchemaIsSet(boolean value) {
if (!value) {
this.schema = null;
}
}
public String getTable() {
return this.table;
}
public ServiceMetaDataArgs setTable(String table) {
this.table = table;
return this;
}
public void unsetTable() {
this.table = null;
}
/** Returns true if field table is set (has been assigned a value) and false otherwise */
public boolean isSetTable() {
return this.table != null;
}
public void setTableIsSet(boolean value) {
if (!value) {
this.table = null;
}
}
public int getTableTypesSize() {
return (this.tableTypes == null) ? 0 : this.tableTypes.size();
}
public java.util.Iterator getTableTypesIterator() {
return (this.tableTypes == null) ? null : this.tableTypes.iterator();
}
public void addToTableTypes(String elem) {
if (this.tableTypes == null) {
this.tableTypes = new ArrayList();
}
this.tableTypes.add(elem);
}
public List getTableTypes() {
return this.tableTypes;
}
public ServiceMetaDataArgs setTableTypes(List tableTypes) {
this.tableTypes = tableTypes;
return this;
}
public void unsetTableTypes() {
this.tableTypes = null;
}
/** Returns true if field tableTypes is set (has been assigned a value) and false otherwise */
public boolean isSetTableTypes() {
return this.tableTypes != null;
}
public void setTableTypesIsSet(boolean value) {
if (!value) {
this.tableTypes = null;
}
}
public String getColumnName() {
return this.columnName;
}
public ServiceMetaDataArgs setColumnName(String columnName) {
this.columnName = columnName;
return this;
}
public void unsetColumnName() {
this.columnName = null;
}
/** Returns true if field columnName is set (has been assigned a value) and false otherwise */
public boolean isSetColumnName() {
return this.columnName != null;
}
public void setColumnNameIsSet(boolean value) {
if (!value) {
this.columnName = null;
}
}
public String getForeignSchema() {
return this.foreignSchema;
}
public ServiceMetaDataArgs setForeignSchema(String foreignSchema) {
this.foreignSchema = foreignSchema;
return this;
}
public void unsetForeignSchema() {
this.foreignSchema = null;
}
/** Returns true if field foreignSchema is set (has been assigned a value) and false otherwise */
public boolean isSetForeignSchema() {
return this.foreignSchema != null;
}
public void setForeignSchemaIsSet(boolean value) {
if (!value) {
this.foreignSchema = null;
}
}
public String getForeignTable() {
return this.foreignTable;
}
public ServiceMetaDataArgs setForeignTable(String foreignTable) {
this.foreignTable = foreignTable;
return this;
}
public void unsetForeignTable() {
this.foreignTable = null;
}
/** Returns true if field foreignTable is set (has been assigned a value) and false otherwise */
public boolean isSetForeignTable() {
return this.foreignTable != null;
}
public void setForeignTableIsSet(boolean value) {
if (!value) {
this.foreignTable = null;
}
}
public String getProcedureName() {
return this.procedureName;
}
public ServiceMetaDataArgs setProcedureName(String procedureName) {
this.procedureName = procedureName;
return this;
}
public void unsetProcedureName() {
this.procedureName = null;
}
/** Returns true if field procedureName is set (has been assigned a value) and false otherwise */
public boolean isSetProcedureName() {
return this.procedureName != null;
}
public void setProcedureNameIsSet(boolean value) {
if (!value) {
this.procedureName = null;
}
}
public String getFunctionName() {
return this.functionName;
}
public ServiceMetaDataArgs setFunctionName(String functionName) {
this.functionName = functionName;
return this;
}
public void unsetFunctionName() {
this.functionName = null;
}
/** Returns true if field functionName is set (has been assigned a value) and false otherwise */
public boolean isSetFunctionName() {
return this.functionName != null;
}
public void setFunctionNameIsSet(boolean value) {
if (!value) {
this.functionName = null;
}
}
public String getAttributeName() {
return this.attributeName;
}
public ServiceMetaDataArgs setAttributeName(String attributeName) {
this.attributeName = attributeName;
return this;
}
public void unsetAttributeName() {
this.attributeName = null;
}
/** Returns true if field attributeName is set (has been assigned a value) and false otherwise */
public boolean isSetAttributeName() {
return this.attributeName != null;
}
public void setAttributeNameIsSet(boolean value) {
if (!value) {
this.attributeName = null;
}
}
public String getTypeName() {
return this.typeName;
}
public ServiceMetaDataArgs setTypeName(String typeName) {
this.typeName = typeName;
return this;
}
public void unsetTypeName() {
this.typeName = null;
}
/** Returns true if field typeName is set (has been assigned a value) and false otherwise */
public boolean isSetTypeName() {
return this.typeName != null;
}
public void setTypeNameIsSet(boolean value) {
if (!value) {
this.typeName = null;
}
}
/**
*
* @see SnappyType
*/
public SnappyType getTypeId() {
return this.typeId;
}
/**
*
* @see SnappyType
*/
public ServiceMetaDataArgs setTypeId(SnappyType typeId) {
this.typeId = typeId;
return this;
}
public void unsetTypeId() {
this.typeId = null;
}
/** Returns true if field typeId is set (has been assigned a value) and false otherwise */
public boolean isSetTypeId() {
return this.typeId != null;
}
public void setTypeIdIsSet(boolean value) {
if (!value) {
this.typeId = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case CONN_ID:
if (value == null) {
unsetConnId();
} else {
setConnId((Long)value);
}
break;
case DRIVER_TYPE:
if (value == null) {
unsetDriverType();
} else {
setDriverType((Byte)value);
}
break;
case TOKEN:
if (value == null) {
unsetToken();
} else {
setToken((ByteBuffer)value);
}
break;
case SCHEMA:
if (value == null) {
unsetSchema();
} else {
setSchema((String)value);
}
break;
case TABLE:
if (value == null) {
unsetTable();
} else {
setTable((String)value);
}
break;
case TABLE_TYPES:
if (value == null) {
unsetTableTypes();
} else {
setTableTypes((List)value);
}
break;
case COLUMN_NAME:
if (value == null) {
unsetColumnName();
} else {
setColumnName((String)value);
}
break;
case FOREIGN_SCHEMA:
if (value == null) {
unsetForeignSchema();
} else {
setForeignSchema((String)value);
}
break;
case FOREIGN_TABLE:
if (value == null) {
unsetForeignTable();
} else {
setForeignTable((String)value);
}
break;
case PROCEDURE_NAME:
if (value == null) {
unsetProcedureName();
} else {
setProcedureName((String)value);
}
break;
case FUNCTION_NAME:
if (value == null) {
unsetFunctionName();
} else {
setFunctionName((String)value);
}
break;
case ATTRIBUTE_NAME:
if (value == null) {
unsetAttributeName();
} else {
setAttributeName((String)value);
}
break;
case TYPE_NAME:
if (value == null) {
unsetTypeName();
} else {
setTypeName((String)value);
}
break;
case TYPE_ID:
if (value == null) {
unsetTypeId();
} else {
setTypeId((SnappyType)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case CONN_ID:
return getConnId();
case DRIVER_TYPE:
return getDriverType();
case TOKEN:
return getToken();
case SCHEMA:
return getSchema();
case TABLE:
return getTable();
case TABLE_TYPES:
return getTableTypes();
case COLUMN_NAME:
return getColumnName();
case FOREIGN_SCHEMA:
return getForeignSchema();
case FOREIGN_TABLE:
return getForeignTable();
case PROCEDURE_NAME:
return getProcedureName();
case FUNCTION_NAME:
return getFunctionName();
case ATTRIBUTE_NAME:
return getAttributeName();
case TYPE_NAME:
return getTypeName();
case TYPE_ID:
return getTypeId();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case CONN_ID:
return isSetConnId();
case DRIVER_TYPE:
return isSetDriverType();
case TOKEN:
return isSetToken();
case SCHEMA:
return isSetSchema();
case TABLE:
return isSetTable();
case TABLE_TYPES:
return isSetTableTypes();
case COLUMN_NAME:
return isSetColumnName();
case FOREIGN_SCHEMA:
return isSetForeignSchema();
case FOREIGN_TABLE:
return isSetForeignTable();
case PROCEDURE_NAME:
return isSetProcedureName();
case FUNCTION_NAME:
return isSetFunctionName();
case ATTRIBUTE_NAME:
return isSetAttributeName();
case TYPE_NAME:
return isSetTypeName();
case TYPE_ID:
return isSetTypeId();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof ServiceMetaDataArgs)
return this.equals((ServiceMetaDataArgs)that);
return false;
}
public boolean equals(ServiceMetaDataArgs that) {
if (that == null)
return false;
boolean this_present_connId = true;
boolean that_present_connId = true;
if (this_present_connId || that_present_connId) {
if (!(this_present_connId && that_present_connId))
return false;
if (this.connId != that.connId)
return false;
}
boolean this_present_driverType = true;
boolean that_present_driverType = true;
if (this_present_driverType || that_present_driverType) {
if (!(this_present_driverType && that_present_driverType))
return false;
if (this.driverType != that.driverType)
return false;
}
boolean this_present_token = true && this.isSetToken();
boolean that_present_token = true && that.isSetToken();
if (this_present_token || that_present_token) {
if (!(this_present_token && that_present_token))
return false;
if (!this.token.equals(that.token))
return false;
}
boolean this_present_schema = true && this.isSetSchema();
boolean that_present_schema = true && that.isSetSchema();
if (this_present_schema || that_present_schema) {
if (!(this_present_schema && that_present_schema))
return false;
if (!this.schema.equals(that.schema))
return false;
}
boolean this_present_table = true && this.isSetTable();
boolean that_present_table = true && that.isSetTable();
if (this_present_table || that_present_table) {
if (!(this_present_table && that_present_table))
return false;
if (!this.table.equals(that.table))
return false;
}
boolean this_present_tableTypes = true && this.isSetTableTypes();
boolean that_present_tableTypes = true && that.isSetTableTypes();
if (this_present_tableTypes || that_present_tableTypes) {
if (!(this_present_tableTypes && that_present_tableTypes))
return false;
if (!this.tableTypes.equals(that.tableTypes))
return false;
}
boolean this_present_columnName = true && this.isSetColumnName();
boolean that_present_columnName = true && that.isSetColumnName();
if (this_present_columnName || that_present_columnName) {
if (!(this_present_columnName && that_present_columnName))
return false;
if (!this.columnName.equals(that.columnName))
return false;
}
boolean this_present_foreignSchema = true && this.isSetForeignSchema();
boolean that_present_foreignSchema = true && that.isSetForeignSchema();
if (this_present_foreignSchema || that_present_foreignSchema) {
if (!(this_present_foreignSchema && that_present_foreignSchema))
return false;
if (!this.foreignSchema.equals(that.foreignSchema))
return false;
}
boolean this_present_foreignTable = true && this.isSetForeignTable();
boolean that_present_foreignTable = true && that.isSetForeignTable();
if (this_present_foreignTable || that_present_foreignTable) {
if (!(this_present_foreignTable && that_present_foreignTable))
return false;
if (!this.foreignTable.equals(that.foreignTable))
return false;
}
boolean this_present_procedureName = true && this.isSetProcedureName();
boolean that_present_procedureName = true && that.isSetProcedureName();
if (this_present_procedureName || that_present_procedureName) {
if (!(this_present_procedureName && that_present_procedureName))
return false;
if (!this.procedureName.equals(that.procedureName))
return false;
}
boolean this_present_functionName = true && this.isSetFunctionName();
boolean that_present_functionName = true && that.isSetFunctionName();
if (this_present_functionName || that_present_functionName) {
if (!(this_present_functionName && that_present_functionName))
return false;
if (!this.functionName.equals(that.functionName))
return false;
}
boolean this_present_attributeName = true && this.isSetAttributeName();
boolean that_present_attributeName = true && that.isSetAttributeName();
if (this_present_attributeName || that_present_attributeName) {
if (!(this_present_attributeName && that_present_attributeName))
return false;
if (!this.attributeName.equals(that.attributeName))
return false;
}
boolean this_present_typeName = true && this.isSetTypeName();
boolean that_present_typeName = true && that.isSetTypeName();
if (this_present_typeName || that_present_typeName) {
if (!(this_present_typeName && that_present_typeName))
return false;
if (!this.typeName.equals(that.typeName))
return false;
}
boolean this_present_typeId = true && this.isSetTypeId();
boolean that_present_typeId = true && that.isSetTypeId();
if (this_present_typeId || that_present_typeId) {
if (!(this_present_typeId && that_present_typeId))
return false;
if (!this.typeId.equals(that.typeId))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy