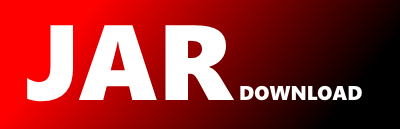
io.snappydata.thrift.StatementResult Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (0.9.3)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package io.snappydata.thrift;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked"})
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2019-08-09")
public class StatementResult implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("StatementResult");
private static final org.apache.thrift.protocol.TField RESULT_SET_FIELD_DESC = new org.apache.thrift.protocol.TField("resultSet", org.apache.thrift.protocol.TType.STRUCT, (short)1);
private static final org.apache.thrift.protocol.TField UPDATE_COUNT_FIELD_DESC = new org.apache.thrift.protocol.TField("updateCount", org.apache.thrift.protocol.TType.I32, (short)2);
private static final org.apache.thrift.protocol.TField BATCH_UPDATE_COUNTS_FIELD_DESC = new org.apache.thrift.protocol.TField("batchUpdateCounts", org.apache.thrift.protocol.TType.LIST, (short)3);
private static final org.apache.thrift.protocol.TField PROCEDURE_OUT_PARAMS_FIELD_DESC = new org.apache.thrift.protocol.TField("procedureOutParams", org.apache.thrift.protocol.TType.MAP, (short)4);
private static final org.apache.thrift.protocol.TField GENERATED_KEYS_FIELD_DESC = new org.apache.thrift.protocol.TField("generatedKeys", org.apache.thrift.protocol.TType.STRUCT, (short)5);
private static final org.apache.thrift.protocol.TField NEW_DEFAULT_SCHEMA_FIELD_DESC = new org.apache.thrift.protocol.TField("newDefaultSchema", org.apache.thrift.protocol.TType.STRING, (short)6);
private static final org.apache.thrift.protocol.TField WARNINGS_FIELD_DESC = new org.apache.thrift.protocol.TField("warnings", org.apache.thrift.protocol.TType.STRUCT, (short)7);
private static final org.apache.thrift.protocol.TField PREPARED_RESULT_FIELD_DESC = new org.apache.thrift.protocol.TField("preparedResult", org.apache.thrift.protocol.TType.STRUCT, (short)8);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class, new StatementResultStandardSchemeFactory());
schemes.put(TupleScheme.class, new StatementResultTupleSchemeFactory());
}
public RowSet resultSet; // optional
public int updateCount; // optional
public List batchUpdateCounts; // optional
public Map procedureOutParams; // optional
public RowSet generatedKeys; // optional
public String newDefaultSchema; // optional
public SnappyExceptionData warnings; // optional
public PrepareResult preparedResult; // optional
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
RESULT_SET((short)1, "resultSet"),
UPDATE_COUNT((short)2, "updateCount"),
BATCH_UPDATE_COUNTS((short)3, "batchUpdateCounts"),
PROCEDURE_OUT_PARAMS((short)4, "procedureOutParams"),
GENERATED_KEYS((short)5, "generatedKeys"),
NEW_DEFAULT_SCHEMA((short)6, "newDefaultSchema"),
WARNINGS((short)7, "warnings"),
PREPARED_RESULT((short)8, "preparedResult");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // RESULT_SET
return RESULT_SET;
case 2: // UPDATE_COUNT
return UPDATE_COUNT;
case 3: // BATCH_UPDATE_COUNTS
return BATCH_UPDATE_COUNTS;
case 4: // PROCEDURE_OUT_PARAMS
return PROCEDURE_OUT_PARAMS;
case 5: // GENERATED_KEYS
return GENERATED_KEYS;
case 6: // NEW_DEFAULT_SCHEMA
return NEW_DEFAULT_SCHEMA;
case 7: // WARNINGS
return WARNINGS;
case 8: // PREPARED_RESULT
return PREPARED_RESULT;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __UPDATECOUNT_ISSET_ID = 0;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.RESULT_SET,_Fields.UPDATE_COUNT,_Fields.BATCH_UPDATE_COUNTS,_Fields.PROCEDURE_OUT_PARAMS,_Fields.GENERATED_KEYS,_Fields.NEW_DEFAULT_SCHEMA,_Fields.WARNINGS,_Fields.PREPARED_RESULT};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.RESULT_SET, new org.apache.thrift.meta_data.FieldMetaData("resultSet", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, RowSet.class)));
tmpMap.put(_Fields.UPDATE_COUNT, new org.apache.thrift.meta_data.FieldMetaData("updateCount", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.BATCH_UPDATE_COUNTS, new org.apache.thrift.meta_data.FieldMetaData("batchUpdateCounts", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32))));
tmpMap.put(_Fields.PROCEDURE_OUT_PARAMS, new org.apache.thrift.meta_data.FieldMetaData("procedureOutParams", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.MapMetaData(org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32),
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, ColumnValue.class))));
tmpMap.put(_Fields.GENERATED_KEYS, new org.apache.thrift.meta_data.FieldMetaData("generatedKeys", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, RowSet.class)));
tmpMap.put(_Fields.NEW_DEFAULT_SCHEMA, new org.apache.thrift.meta_data.FieldMetaData("newDefaultSchema", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.WARNINGS, new org.apache.thrift.meta_data.FieldMetaData("warnings", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, SnappyExceptionData.class)));
tmpMap.put(_Fields.PREPARED_RESULT, new org.apache.thrift.meta_data.FieldMetaData("preparedResult", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, PrepareResult.class)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(StatementResult.class, metaDataMap);
}
public StatementResult() {
}
/**
* Performs a deep copy on other.
*/
public StatementResult(StatementResult other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetResultSet()) {
this.resultSet = new RowSet(other.resultSet);
}
this.updateCount = other.updateCount;
if (other.isSetBatchUpdateCounts()) {
List __this__batchUpdateCounts = new ArrayList(other.batchUpdateCounts);
this.batchUpdateCounts = __this__batchUpdateCounts;
}
if (other.isSetProcedureOutParams()) {
Map __this__procedureOutParams = new HashMap(other.procedureOutParams.size());
for (Map.Entry other_element : other.procedureOutParams.entrySet()) {
Integer other_element_key = other_element.getKey();
ColumnValue other_element_value = other_element.getValue();
Integer __this__procedureOutParams_copy_key = other_element_key;
ColumnValue __this__procedureOutParams_copy_value = new ColumnValue(other_element_value);
__this__procedureOutParams.put(__this__procedureOutParams_copy_key, __this__procedureOutParams_copy_value);
}
this.procedureOutParams = __this__procedureOutParams;
}
if (other.isSetGeneratedKeys()) {
this.generatedKeys = new RowSet(other.generatedKeys);
}
if (other.isSetNewDefaultSchema()) {
this.newDefaultSchema = other.newDefaultSchema;
}
if (other.isSetWarnings()) {
this.warnings = new SnappyExceptionData(other.warnings);
}
if (other.isSetPreparedResult()) {
this.preparedResult = new PrepareResult(other.preparedResult);
}
}
public StatementResult deepCopy() {
return new StatementResult(this);
}
@Override
public void clear() {
this.resultSet = null;
setUpdateCountIsSet(false);
this.updateCount = 0;
this.batchUpdateCounts = null;
this.procedureOutParams = null;
this.generatedKeys = null;
this.newDefaultSchema = null;
this.warnings = null;
this.preparedResult = null;
}
public RowSet getResultSet() {
return this.resultSet;
}
public StatementResult setResultSet(RowSet resultSet) {
this.resultSet = resultSet;
return this;
}
public void unsetResultSet() {
this.resultSet = null;
}
/** Returns true if field resultSet is set (has been assigned a value) and false otherwise */
public boolean isSetResultSet() {
return this.resultSet != null;
}
public void setResultSetIsSet(boolean value) {
if (!value) {
this.resultSet = null;
}
}
public int getUpdateCount() {
return this.updateCount;
}
public StatementResult setUpdateCount(int updateCount) {
this.updateCount = updateCount;
setUpdateCountIsSet(true);
return this;
}
public void unsetUpdateCount() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __UPDATECOUNT_ISSET_ID);
}
/** Returns true if field updateCount is set (has been assigned a value) and false otherwise */
public boolean isSetUpdateCount() {
return EncodingUtils.testBit(__isset_bitfield, __UPDATECOUNT_ISSET_ID);
}
public void setUpdateCountIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __UPDATECOUNT_ISSET_ID, value);
}
public int getBatchUpdateCountsSize() {
return (this.batchUpdateCounts == null) ? 0 : this.batchUpdateCounts.size();
}
public java.util.Iterator getBatchUpdateCountsIterator() {
return (this.batchUpdateCounts == null) ? null : this.batchUpdateCounts.iterator();
}
public void addToBatchUpdateCounts(int elem) {
if (this.batchUpdateCounts == null) {
this.batchUpdateCounts = new ArrayList();
}
this.batchUpdateCounts.add(elem);
}
public List getBatchUpdateCounts() {
return this.batchUpdateCounts;
}
public StatementResult setBatchUpdateCounts(List batchUpdateCounts) {
this.batchUpdateCounts = batchUpdateCounts;
return this;
}
public void unsetBatchUpdateCounts() {
this.batchUpdateCounts = null;
}
/** Returns true if field batchUpdateCounts is set (has been assigned a value) and false otherwise */
public boolean isSetBatchUpdateCounts() {
return this.batchUpdateCounts != null;
}
public void setBatchUpdateCountsIsSet(boolean value) {
if (!value) {
this.batchUpdateCounts = null;
}
}
public int getProcedureOutParamsSize() {
return (this.procedureOutParams == null) ? 0 : this.procedureOutParams.size();
}
public void putToProcedureOutParams(int key, ColumnValue val) {
if (this.procedureOutParams == null) {
this.procedureOutParams = new HashMap();
}
this.procedureOutParams.put(key, val);
}
public Map getProcedureOutParams() {
return this.procedureOutParams;
}
public StatementResult setProcedureOutParams(Map procedureOutParams) {
this.procedureOutParams = procedureOutParams;
return this;
}
public void unsetProcedureOutParams() {
this.procedureOutParams = null;
}
/** Returns true if field procedureOutParams is set (has been assigned a value) and false otherwise */
public boolean isSetProcedureOutParams() {
return this.procedureOutParams != null;
}
public void setProcedureOutParamsIsSet(boolean value) {
if (!value) {
this.procedureOutParams = null;
}
}
public RowSet getGeneratedKeys() {
return this.generatedKeys;
}
public StatementResult setGeneratedKeys(RowSet generatedKeys) {
this.generatedKeys = generatedKeys;
return this;
}
public void unsetGeneratedKeys() {
this.generatedKeys = null;
}
/** Returns true if field generatedKeys is set (has been assigned a value) and false otherwise */
public boolean isSetGeneratedKeys() {
return this.generatedKeys != null;
}
public void setGeneratedKeysIsSet(boolean value) {
if (!value) {
this.generatedKeys = null;
}
}
public String getNewDefaultSchema() {
return this.newDefaultSchema;
}
public StatementResult setNewDefaultSchema(String newDefaultSchema) {
this.newDefaultSchema = newDefaultSchema;
return this;
}
public void unsetNewDefaultSchema() {
this.newDefaultSchema = null;
}
/** Returns true if field newDefaultSchema is set (has been assigned a value) and false otherwise */
public boolean isSetNewDefaultSchema() {
return this.newDefaultSchema != null;
}
public void setNewDefaultSchemaIsSet(boolean value) {
if (!value) {
this.newDefaultSchema = null;
}
}
public SnappyExceptionData getWarnings() {
return this.warnings;
}
public StatementResult setWarnings(SnappyExceptionData warnings) {
this.warnings = warnings;
return this;
}
public void unsetWarnings() {
this.warnings = null;
}
/** Returns true if field warnings is set (has been assigned a value) and false otherwise */
public boolean isSetWarnings() {
return this.warnings != null;
}
public void setWarningsIsSet(boolean value) {
if (!value) {
this.warnings = null;
}
}
public PrepareResult getPreparedResult() {
return this.preparedResult;
}
public StatementResult setPreparedResult(PrepareResult preparedResult) {
this.preparedResult = preparedResult;
return this;
}
public void unsetPreparedResult() {
this.preparedResult = null;
}
/** Returns true if field preparedResult is set (has been assigned a value) and false otherwise */
public boolean isSetPreparedResult() {
return this.preparedResult != null;
}
public void setPreparedResultIsSet(boolean value) {
if (!value) {
this.preparedResult = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case RESULT_SET:
if (value == null) {
unsetResultSet();
} else {
setResultSet((RowSet)value);
}
break;
case UPDATE_COUNT:
if (value == null) {
unsetUpdateCount();
} else {
setUpdateCount((Integer)value);
}
break;
case BATCH_UPDATE_COUNTS:
if (value == null) {
unsetBatchUpdateCounts();
} else {
setBatchUpdateCounts((List)value);
}
break;
case PROCEDURE_OUT_PARAMS:
if (value == null) {
unsetProcedureOutParams();
} else {
setProcedureOutParams((Map)value);
}
break;
case GENERATED_KEYS:
if (value == null) {
unsetGeneratedKeys();
} else {
setGeneratedKeys((RowSet)value);
}
break;
case NEW_DEFAULT_SCHEMA:
if (value == null) {
unsetNewDefaultSchema();
} else {
setNewDefaultSchema((String)value);
}
break;
case WARNINGS:
if (value == null) {
unsetWarnings();
} else {
setWarnings((SnappyExceptionData)value);
}
break;
case PREPARED_RESULT:
if (value == null) {
unsetPreparedResult();
} else {
setPreparedResult((PrepareResult)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case RESULT_SET:
return getResultSet();
case UPDATE_COUNT:
return getUpdateCount();
case BATCH_UPDATE_COUNTS:
return getBatchUpdateCounts();
case PROCEDURE_OUT_PARAMS:
return getProcedureOutParams();
case GENERATED_KEYS:
return getGeneratedKeys();
case NEW_DEFAULT_SCHEMA:
return getNewDefaultSchema();
case WARNINGS:
return getWarnings();
case PREPARED_RESULT:
return getPreparedResult();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case RESULT_SET:
return isSetResultSet();
case UPDATE_COUNT:
return isSetUpdateCount();
case BATCH_UPDATE_COUNTS:
return isSetBatchUpdateCounts();
case PROCEDURE_OUT_PARAMS:
return isSetProcedureOutParams();
case GENERATED_KEYS:
return isSetGeneratedKeys();
case NEW_DEFAULT_SCHEMA:
return isSetNewDefaultSchema();
case WARNINGS:
return isSetWarnings();
case PREPARED_RESULT:
return isSetPreparedResult();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof StatementResult)
return this.equals((StatementResult)that);
return false;
}
public boolean equals(StatementResult that) {
if (that == null)
return false;
boolean this_present_resultSet = true && this.isSetResultSet();
boolean that_present_resultSet = true && that.isSetResultSet();
if (this_present_resultSet || that_present_resultSet) {
if (!(this_present_resultSet && that_present_resultSet))
return false;
if (!this.resultSet.equals(that.resultSet))
return false;
}
boolean this_present_updateCount = true && this.isSetUpdateCount();
boolean that_present_updateCount = true && that.isSetUpdateCount();
if (this_present_updateCount || that_present_updateCount) {
if (!(this_present_updateCount && that_present_updateCount))
return false;
if (this.updateCount != that.updateCount)
return false;
}
boolean this_present_batchUpdateCounts = true && this.isSetBatchUpdateCounts();
boolean that_present_batchUpdateCounts = true && that.isSetBatchUpdateCounts();
if (this_present_batchUpdateCounts || that_present_batchUpdateCounts) {
if (!(this_present_batchUpdateCounts && that_present_batchUpdateCounts))
return false;
if (!this.batchUpdateCounts.equals(that.batchUpdateCounts))
return false;
}
boolean this_present_procedureOutParams = true && this.isSetProcedureOutParams();
boolean that_present_procedureOutParams = true && that.isSetProcedureOutParams();
if (this_present_procedureOutParams || that_present_procedureOutParams) {
if (!(this_present_procedureOutParams && that_present_procedureOutParams))
return false;
if (!this.procedureOutParams.equals(that.procedureOutParams))
return false;
}
boolean this_present_generatedKeys = true && this.isSetGeneratedKeys();
boolean that_present_generatedKeys = true && that.isSetGeneratedKeys();
if (this_present_generatedKeys || that_present_generatedKeys) {
if (!(this_present_generatedKeys && that_present_generatedKeys))
return false;
if (!this.generatedKeys.equals(that.generatedKeys))
return false;
}
boolean this_present_newDefaultSchema = true && this.isSetNewDefaultSchema();
boolean that_present_newDefaultSchema = true && that.isSetNewDefaultSchema();
if (this_present_newDefaultSchema || that_present_newDefaultSchema) {
if (!(this_present_newDefaultSchema && that_present_newDefaultSchema))
return false;
if (!this.newDefaultSchema.equals(that.newDefaultSchema))
return false;
}
boolean this_present_warnings = true && this.isSetWarnings();
boolean that_present_warnings = true && that.isSetWarnings();
if (this_present_warnings || that_present_warnings) {
if (!(this_present_warnings && that_present_warnings))
return false;
if (!this.warnings.equals(that.warnings))
return false;
}
boolean this_present_preparedResult = true && this.isSetPreparedResult();
boolean that_present_preparedResult = true && that.isSetPreparedResult();
if (this_present_preparedResult || that_present_preparedResult) {
if (!(this_present_preparedResult && that_present_preparedResult))
return false;
if (!this.preparedResult.equals(that.preparedResult))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy